
Simple USB-MIDI foot controller
Dependencies: PinDetect USBDevice_STM32F103 mbed-STM32F103C8T6
midiswitch.h
00001 #ifndef MIDISWITCH_H 00002 #define MIDISWITCH_H 00003 00004 #include "mbed.h" 00005 #include "mbed_events.h" 00006 #include "PinDetect.h" 00007 #include "USBMIDI.h" 00008 00009 00010 typedef struct SwitchConfig { 00011 PinName pin; 00012 uint8_t type; 00013 uint8_t channel; 00014 uint8_t data1; 00015 int8_t on_value; 00016 int8_t off_value; 00017 } SwitchConfig; 00018 00019 typedef void (*MIDI_CB)(MIDIMessage); 00020 00021 class SwitchHandler { 00022 public: 00023 SwitchHandler(EventQueue * queue, MIDI_CB cb, SwitchConfig sw); 00024 void handle_pressed(void); 00025 void handle_released(void); 00026 void setConfig(SwitchConfig sw) { cfg = sw; }; 00027 private: 00028 bool make_message(bool onoff); 00029 EventQueue * queue; 00030 MIDI_CB write_cb; 00031 PinDetect btn; 00032 SwitchConfig cfg; 00033 MIDIMessage msg; 00034 }; 00035 00036 #endif /* MIDISWITCH_H */
Generated on Mon Jul 18 2022 23:54:16 by
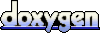