
WiFi DipCortex / CC3000 Demo - Contains a menu driven set of tests to initalise and control the CC3000 radio. Also allowing you to test various TCP and UDP connections.
Dependencies: NTPClient WebSocketClient cc3000_hostdriver_mbedsocket mbed HTTPClient
wifi.cpp
00001 #include "mbed.h" 00002 #include "cc3000.h" 00003 #include "wifi.h" 00004 00005 using namespace mbed_cc3000; 00006 00007 tUserFS cc_user_info; 00008 00009 const char * WIFI_STATUS[] = {"Disconnected", "Scanning", "Connecting", "Connected"}; 00010 00011 /* cc3000 module declaration specific for user's board. Check also init() */ 00012 #if (MY_BOARD == WIGO) 00013 cc3000 wifi(PTA16, PTA13, PTD0, SPI(PTD2, PTD3, PTC5), PORTA_IRQn); 00014 #elif (MY_BOARD == WIFI_DIPCORTEX) 00015 cc3000 wifi(p28, p27, p30, SPI(p21, p14, p37), PIN_INT0_IRQn); 00016 #else 00017 00018 #endif 00019 00020 #ifndef CC3000_UNENCRYPTED_SMART_CONFIG 00021 const uint8_t smartconfigkey[] = {0x73,0x6d,0x61,0x72,0x74,0x63,0x6f,0x6e,0x66,0x69,0x67,0x41,0x45,0x53,0x31,0x36}; 00022 #else 00023 const uint8_t smartconfigkey = 0; 00024 #endif 00025 00026 #if (MY_BOARD == WIGO) 00027 00028 #include "NVIC_set_all_priorities.h" 00029 00030 /** 00031 * \brief Wi-Go initialization 00032 * \param none 00033 * \return none 00034 */ 00035 void init() { 00036 DigitalOut PWR_EN1(PTB2); 00037 DigitalOut PWR_EN2(PTB3); 00038 00039 // Wi-Go set current to 500mA since we're turning on the Wi-Fi 00040 PWR_EN1 = 0; 00041 PWR_EN2 = 1; 00042 00043 NVIC_set_all_irq_priorities(3); 00044 NVIC_SetPriority(SPI0_IRQn, 0x0); // Wi-Fi SPI interrupt must be higher priority than SysTick 00045 NVIC_SetPriority(PORTA_IRQn, 0x1); 00046 NVIC_SetPriority(SysTick_IRQn, 0x2); // SysTick set to lower priority than Wi-Fi SPI bus interrupt 00047 PORTA->PCR[16] |=PORT_PCR_ISF_MASK; 00048 PORTA->ISFR |= (1<<16); 00049 } 00050 00051 #elif (MY_BOARD == WIFI_DIPCORTEX) 00052 00053 /** 00054 * \brief Wifi DipCortex initialization 00055 * \param none 00056 * \return none 00057 */ 00058 void init() 00059 { 00060 NVIC_SetPriority(SSP1_IRQn, 0x0); 00061 NVIC_SetPriority(PIN_INT0_IRQn, 0x1); 00062 00063 // SysTick set to lower priority than Wi-Fi SPI bus interrupt 00064 NVIC_SetPriority(SysTick_IRQn, 0x2); 00065 } 00066 00067 #else 00068 00069 /** 00070 * \brief Place here init routine for your board 00071 * \param none 00072 * \return none 00073 */ 00074 void init() { 00075 00076 } 00077 00078 #endif 00079 00080 00081 00082 00083 00084 /** 00085 * \brief Print cc3000 information 00086 * \param none 00087 * \return none 00088 */ 00089 void print_cc3000_info() { 00090 uint8_t myMAC[8]; 00091 uint8_t buffer[2]; 00092 int32_t status = 0; 00093 tNetappIpconfigRetArgs ipinfo2; 00094 00095 wifi.get_user_file_info((uint8_t *)&cc_user_info, sizeof(cc_user_info)); 00096 wifi.get_mac_address(myMAC); 00097 printf(" MAC address : %02x:%02x:%02x:%02x:%02x:%02x\r\n", myMAC[0], myMAC[1], myMAC[2], myMAC[3], myMAC[4], myMAC[5]); 00098 00099 if (! wifi._nvmem.read_sp_version( (unsigned char*)&buffer ) ) 00100 { 00101 printf(" CC3000 Firmware Version : %u.%u \r\n", buffer[0], buffer[1]); 00102 } 00103 else 00104 { 00105 printf(" CC3000 Read nvmem failed!"); 00106 } 00107 00108 status = wifi._wlan.ioctl_statusget(); 00109 if (( status > -1 ) && ( status < 4 )) 00110 { 00111 printf(" Wifi Status : %s\r\n", WIFI_STATUS[status]); 00112 } 00113 else 00114 { 00115 printf(" Wifi Status : %d\r\n", status); 00116 } 00117 00118 if ( wifi.is_dhcp_configured() ) 00119 { 00120 wifi.get_ip_config(&ipinfo2); 00121 printf(" Connected to : %s \r\n", ipinfo2.uaSSID); 00122 printf(" IP : %d.%d.%d.%d \r\n", ipinfo2.aucIP[3], ipinfo2.aucIP[2], ipinfo2.aucIP[1], ipinfo2.aucIP[0]); 00123 printf(" Gateway : %d.%d.%d.%d \r\n", ipinfo2.aucDefaultGateway[3], ipinfo2.aucDefaultGateway[2], ipinfo2.aucDefaultGateway[1], ipinfo2.aucDefaultGateway[0]); 00124 printf(" Subnet : %d.%d.%d.%d \r\n", ipinfo2.aucSubnetMask[3], ipinfo2.aucSubnetMask[2], ipinfo2.aucSubnetMask[1], ipinfo2.aucSubnetMask[0]); 00125 printf(" DNS : %d.%d.%d.%d \r\n", ipinfo2.aucDNSServer[3], ipinfo2.aucDNSServer[2], ipinfo2.aucDNSServer[1], ipinfo2.aucDNSServer[0]); 00126 00127 printf(" Cached IP : %s \r\n", wifi.getIPAddress()); 00128 printf(" Cached Gateway : %s \r\n", wifi.getGateway()); 00129 printf(" Cached Subnet : %s \r\n", wifi.getNetworkMask()); 00130 00131 } 00132 else 00133 { 00134 printf(" Not connected \r\n"); 00135 } 00136 00137 // This doesn't work for the wifi dip 00138 printf(" FTC %i\r\n",cc_user_info.FTC); 00139 printf(" PP_version %i.%i\r\n",cc_user_info.PP_version[0], cc_user_info.PP_version[1]); 00140 printf(" SERV_PACK %i.%i\r\n",cc_user_info.SERV_PACK[0], cc_user_info.SERV_PACK[1]); 00141 printf(" DRV_VER %i.%i.%i\r\n",cc_user_info.DRV_VER[0], cc_user_info.DRV_VER[1], cc_user_info.DRV_VER[2]); 00142 printf(" FW_VER %i.%i.%i\r\n",cc_user_info.FW_VER[0], cc_user_info.FW_VER[1], cc_user_info.FW_VER[2]); 00143 } 00144 00145 /** 00146 * \brief Connect to SSID with a timeout 00147 * \param ssid Name of SSID 00148 * \param key Password 00149 * \param sec_mode Security mode 00150 * \return none 00151 */ 00152 void connect_to_ssid(char *ssid, char *key, unsigned char sec_mode) { 00153 printf("Connecting to SSID: %s. Timeout is 10s.\n",ssid); 00154 if (wifi.connect_to_AP((uint8_t *)ssid, (uint8_t *)key, sec_mode) == true) { 00155 printf(" Connected\r\n"); 00156 } else { 00157 printf(" Connection timed-out (error). Please restart.\r\n"); 00158 while(1); 00159 } 00160 } 00161 00162 /** 00163 * \brief Connect to SSID without security 00164 * \param ssid Name of SSID 00165 * \return none 00166 */ 00167 void connect_to_ssid(uint8_t *ssid) { 00168 wifi.connect_open((uint8_t *)ssid); 00169 } 00170 00171 /** 00172 * \brief First time configuration 00173 * \param none 00174 * \return none 00175 */ 00176 void do_FTC(void) 00177 { 00178 /* 00179 printf("Running First Time Configuration\r\n"); 00180 wifi.start_smart_config(smartconfigkey); 00181 while (wifi.is_dhcp_configured() == false) { 00182 wait_ms(500); 00183 printf("Waiting for dhcp to be set.\r\n"); 00184 } 00185 cc_user_info.FTC = 1; 00186 wifi.set_user_file_info((uint8_t *)&user_info, sizeof(user_info)); 00187 wifi._wlan.stop(); 00188 printf("FTC finished.\r\n"); 00189 */ 00190 }
Generated on Wed Jul 13 2022 02:13:28 by
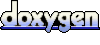