Library for Si114x optical sensors. Currently intended for the Si1146, although it will work also for the Si1145, and without full functionality for the Si1147
Dependents: Si114x_HelloWorld Hello-Uzuki-sensor-shield
Si114x.h
00001 #ifndef SI114X_H 00002 #define SI114X_H 00003 00004 00005 #include "mbed.h" 00006 /* 00007 * Class to interface with Si114x sensors 00008 * 00009 * Currently only intended for Si1146. Should work also on the Si1145, 00010 * but the extra LED of the Si1147 is not supported. Everyone is welcome 00011 * to add this support and submit a pull request. 00012 * 00013 * Aditionally only synchronous mode is for now supported (no async I2C API is used 00014 * and the sensor is not placed in automatic measurement mode: Each request starts a new 00015 * measurement. Also for this and other issues: Everyone is welcome to submit 00016 * pull requests 00017 * 00018 * For now this is just returning raw values, no scaling is done by the library code 00019 */ 00020 class Si114x { 00021 public: 00022 /* Constructor to create new Si114x instance 00023 * 00024 * @param sda SDA pin of the I2C interface 00025 * @param scl SCL pin of the I2C interface 00026 */ 00027 Si114x(PinName sda, PinName scl); 00028 00029 /* Get the measure visible light intensity 00030 * 00031 * @return unsigned short (uint16_t) representing visible light intensity 00032 */ 00033 unsigned short getVisibleLight(void); 00034 00035 /* Get the measure IR light intensity 00036 * 00037 * @return unsigned short (uint16_t) representing IR light intensity 00038 */ 00039 unsigned short getIRLight(void); 00040 00041 /* Get the measure UV intensity 00042 * 00043 * The retval is supposed to be 10 times the UV index. It will be modified 00044 * to return a float representing the UV index once it doesn't claim 00045 * the UV index is 50 outside. 00046 * 00047 * @return unsigned short (uint16_t) representing UV intensity 00048 */ 00049 unsigned short getUVIndex(void); 00050 00051 /* Perform a proximity measurement 00052 * 00053 * This enabled LEDs (set using setPSLEDs) and performs a 00054 * reflection measurement. It can be used to obtain (relative) 00055 * proximity, but also to measure for example heartbeat, as in 00056 * the HelloWorld program. 00057 * 00058 * Currently the code does not substract ambient light (todo) 00059 * 00060 * @param pschannel channel to use for the measurement (1-2) 00061 * @return unsigned short (uint16_t) representing the reflection 00062 */ 00063 unsigned short getProximity(char pschannel); 00064 00065 /* Set the LEDs for a given proximity measurement 00066 * 00067 * The Si1146 has two channels to do measurements with and can control 2 LEDs. 00068 * This function is used to set which LEDs are used for which channel. 00069 * Multiple LEDs can be active at the same time. By default the 00070 * constructor sets LED1 to be active with channel 1 and LED2 to 00071 * be active with channel 2. 00072 * 00073 * @param pschannel channel to set active LEDs for (1-2) 00074 * @param ledmask LEDs to be active for the set channel (bit 0 is LED1, bit1 is LED2) 00075 */ 00076 void setPSLEDs(char pschannel, char ledmask); 00077 00078 /* Verify I2C communication can occur with the sensor 00079 * 00080 * @return true when the sensor is found 00081 */ 00082 bool verifyConnection(void); 00083 00084 protected: 00085 char read_byte(const char reg); 00086 unsigned short read_word(const char reg); 00087 void write_byte(const char reg, const char byte); 00088 void param_set(const char param, const char value); 00089 char param_query(const char param); 00090 void write_cmd(const char cmd); 00091 00092 I2C i2c; 00093 00094 bool continious; 00095 }; 00096 00097 #endif
Generated on Mon Jul 18 2022 19:16:33 by
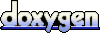