Allows easy usage of the LPC1768 RTC interrupts
Dependents: Mini_DK_clk MakerBotServer GT-ACQUAPLUS_Consumo GT-ACQUAPLUS_Eficiencia ... more
RTC.h
00001 #ifndef RTC_H 00002 #define RTC_H 00003 00004 #include "mbed.h" 00005 00006 /** 00007 * Library to get access to the interrupt functionality of the LPC1768's RTC. 00008 * 00009 * This class is completely static: which means you don't have to create an RTC object, 00010 * there is always one object automatically created when you include this class. Since 00011 * there is only one RTC, more than one would make no sense. 00012 * 00013 * @code 00014 * #include "mbed.h" 00015 * #include "RTC.h" 00016 * 00017 * DigitalOut led(LED1); 00018 * 00019 * void ledFunction( void ) 00020 * { 00021 * led = 1; 00022 * RTC::detach(RTC::Second); 00023 * } 00024 * 00025 * void displayFunction( void ) 00026 * { 00027 * time_t seconds = time(NULL); 00028 * printf("%s", ctime(&seconds)); 00029 * } 00030 * 00031 * void alarmFunction( void ) 00032 * { 00033 * error("Not most useful alarm function"); 00034 * } 00035 * 00036 * int main() 00037 * { 00038 * set_time(1256729737); // Set time to Wed, 28 Oct 2009 11:35:37 00039 * 00040 * tm t = RTC::getDefaultTM(); 00041 * t.tm_sec = 5; 00042 * t.tm_min = 36; 00043 * 00044 * RTC::alarm(&alarmFunction, t); 00045 * RTC::attach(&displayFunction, RTC::Second); 00046 * RTC::attach(&ledFunction, RTC::Minute); 00047 * 00048 * while(1); 00049 * } 00050 * @endcode 00051 **/ 00052 class RTC { 00053 public: 00054 /** 00055 * Available time units for interrupts 00056 * 00057 * RTC::Second, RTC::Minute, RTC::Hour, 00058 * RTC::Day, RTC::Month, RTC::Year 00059 */ 00060 enum TimeUnit {Second, Minute, Hour, 00061 Day, Month, Year}; 00062 00063 /** 00064 * Call a function when the specified time unit increases 00065 * 00066 * You can attach one function for each TimeUnit. When several are 00067 * attached the smalles TimeUnit is called first. 00068 * 00069 * Member functions of a class can be attached the normal way (similar to for example Ticker). 00070 * 00071 * @param function - the function to call 00072 * @param interval - the TimeUnit which specifies the interval 00073 */ 00074 static void attach(void (*function)(void), TimeUnit interval); 00075 template<typename T> 00076 void attach(T *object, void (T::*member)(void), TimeUnit interval); 00077 00078 /** 00079 * Detach an interrupt function 00080 * 00081 * @param interval - the TimeUnit of the interrupt to detach 00082 */ 00083 static void detach(TimeUnit interval); 00084 00085 /** 00086 * Call a function when a specified time is reached 00087 * 00088 * Only one alarm is possible. Make fields of the tm structure -1 for don't care. 00089 * 00090 * Member functions of a class can be attached the normal way (similar to for example Ticker). 00091 * 00092 * @param function - the function to call 00093 * @param alarmTime - tm structure which specifies when to activate the alarm 00094 */ 00095 static void alarm(void (*function)(void), tm alarmTime); 00096 template<typename T> 00097 void alarm(T *object, void (T::*member)(void), tm alarmTime); 00098 00099 /** 00100 * Disable the alarm 00101 */ 00102 static void alarmOff( void ); 00103 00104 /** 00105 * Returns a default tm structure where each field is initialized 00106 * to -1, so it is ignored by the alarm function. 00107 * 00108 * Available fields: http://www.cplusplus.com/reference/ctime/tm/ 00109 * Except tm_isdst all of them can be used for the alarm 00110 * 00111 * @param return - tm structure initialized to -1 00112 */ 00113 static tm getDefaultTM( void ); 00114 00115 00116 00117 private: 00118 static void IRQHandler( void ); 00119 00120 static FunctionPointer attachCB[6]; 00121 static FunctionPointer alarmCB; 00122 00123 //If someone knows a nicer way to do this, please tell me 00124 static bool initialRun; 00125 static void _attach(TimeUnit interval); 00126 static void _alarm(tm alarmTime); 00127 00128 00129 }; 00130 00131 #endif
Generated on Wed Jul 13 2022 05:23:28 by
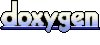