
Simple clock program for LPC1768 Mini-DK
Embed:
(wiki syntax)
Show/hide line numbers
Touch.h
00001 00002 #ifndef MBED_Touch_H 00003 #define MBED_Touch_H 00004 00005 #include "SPI_TFT.h" 00006 #include "mbed.h" 00007 00008 typedef struct 00009 { 00010 int x; 00011 int y; 00012 } Coordinate; 00013 00014 typedef struct 00015 { 00016 int An, 00017 Bn, 00018 Cn, 00019 Dn, 00020 En, 00021 Fn, 00022 Divider ; 00023 } Matrix; 00024 00025 class TouchScreenADS7843 : public SPI_TFT 00026 { 00027 public: 00028 // (To be modified) C code below works on KEIL compiler but NOT on LPCXpresso/mbed 00029 // extern Coordinate ScreenSample[3]; 00030 // extern Coordinate DisplaySample[3]; 00031 // extern Matrix matrix ; 00032 // extern Coordinate display ; 00033 00034 TouchScreenADS7843(PinName tp_mosi,PinName tp_miso,PinName tp_sclk,PinName tp_cs,PinName tp_irq,PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset,const char* name ="TFT"); 00035 00036 void TP_Init(void); 00037 void TP_GetAdXY(int *x,int *y); 00038 void TP_DrawPoint(unsigned int Xpos,unsigned int Ypos,unsigned int color); 00039 // Coordinate Read_Ads7846(void); 00040 unsigned char Read_Ads7846(Coordinate * screenPtr); 00041 void TouchPanel_Calibrate(Matrix * matrixPtr); 00042 unsigned char getDisplayPoint(Coordinate * displayPtr,Coordinate * screenPtr,Matrix * matrixPtr ); 00043 00044 SPI _tp_spi; 00045 DigitalOut _tp_cs; 00046 DigitalIn _tp_irq; 00047 00048 protected: 00049 00050 #define SPI_RD_DELAY 1 00051 #define CHX 0xd0 // 12 bit mode 00052 #define CHY 0x90 00053 00054 int Read_XY(unsigned char XY); 00055 void DrawCross(unsigned int Xpos,unsigned int Ypos); 00056 unsigned char setCalibrationMatrix( Coordinate * displayPtr,Coordinate * screenPtr,Matrix * matrixPtr); 00057 00058 }; 00059 #endif
Generated on Tue Jul 12 2022 17:26:32 by
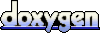