Replacement for regular GPIO (DigitalIn, DigitalOut, DigitalInOut) classes which has superior speed.
Dependents: Eavesdropper BitstreamGenerator SimpleDecimationFilter 11U68_MP3Player with TFTLCD ... more
FastIO_EFM32.h
00001 #if defined(TARGET_EFM32) 00002 00003 #include "mbed.h" 00004 #include "pinmap.h" 00005 #include "em_cmu.h" 00006 00007 typedef struct { 00008 uint32_t mask; 00009 uint8_t input_mode; 00010 uint8_t output_mode; 00011 } fastio_vars; 00012 00013 #define PIN_INDEX (pin & 0xF) 00014 #define PINMASK (1 << PIN_INDEX) 00015 #define PORT_INDEX (pin >> 4) 00016 00017 //Mode_reg is either high or low, depending on first 8 bit or second 8-bit of a port 00018 #define MODE_REG (*((&GPIO->P[PORT_INDEX].MODEL) + PIN_INDEX / 8)) 00019 #define MODE_SHIFT ((PIN_INDEX * 4) % 32) 00020 00021 #define INIT_PIN this->container.mask = PINMASK; this->container.input_mode = PullDefault; this->container.output_mode = PushPull; CMU_ClockEnable(cmuClock_HFPER, true); CMU_ClockEnable(cmuClock_GPIO, true) 00022 #define DESTROY_PIN 00023 00024 #define SET_DIR_INPUT uint32_t temp = MODE_REG & ~(0xF << MODE_SHIFT); MODE_REG = temp + ((this->container.input_mode & 0xF) << MODE_SHIFT); if (this->container.input_mode > 0x10) WRITE_PIN_SET; else WRITE_PIN_CLR 00025 #define SET_DIR_OUTPUT uint32_t temp = MODE_REG & ~(0xF << MODE_SHIFT); MODE_REG = temp + (container.output_mode << MODE_SHIFT) 00026 #define SET_MODE(pull) if ((pull <= 3) || (pull > 0x10)) {this->container.input_mode = pull; SET_DIR_INPUT; } else {this->container.output_mode = pull; SET_DIR_OUTPUT;} 00027 00028 #define WRITE_PIN_SET GPIO->P[PORT_INDEX].DOUTSET = PINMASK 00029 #define WRITE_PIN_CLR GPIO->P[PORT_INDEX].DOUTCLR = PINMASK 00030 00031 #define READ_PIN ((GPIO->P[PORT_INDEX].DIN & this->container.mask) ? 1 : 0) 00032 00033 #endif
Generated on Tue Jul 12 2022 19:39:08 by
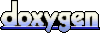