Library to make use of the DS1302 timekeeping IC. The functions are identical to those used by the mbed RTC's
Dependents: temp_humid_time_DS1302_LM35_DHT11 temp_humid_light_time_DS1302_LM35_DHT11_LDR DSKY DS1302_HelloWorld ... more
DS1302.h
00001 //The license: Its on the internet, have fun with it. 00002 00003 #ifndef DS1302_H 00004 #define DS1302_H 00005 00006 #include "mbed.h" 00007 00008 /** 00009 * Library to make use of the DS1302 timekeeping IC 00010 * 00011 * The library functions the same as the standard mbed time function, 00012 * only now you have to first make a DS1302 object and apply the 00013 * functions on the object. 00014 * 00015 * Example code: 00016 * @code 00017 * #define SCLK PTC5 00018 * #define IO PTC4 00019 * #define CE PTC3 00020 * 00021 * //Comment this line if the DS1302 is already running 00022 * #define INITIAL_RUN 00023 * 00024 * #include "mbed.h" 00025 * #include "DS1302.h" 00026 * 00027 * DS1302 clk(SCLK, IO, PTC3); 00028 * 00029 * int main() { 00030 * #ifdef INITIAL_RUN 00031 * clk.set_time(1256729737); 00032 * #endif 00033 * 00034 * char storedByte = clk.recallByte(0); 00035 * printf("Stored byte was %d, now increasing by one\r\n", storedByte); 00036 * clk.storeByte(0, storedByte + 1); 00037 * 00038 * while(1) { 00039 * time_t seconds = clk.time(NULL); 00040 * printf("Time as a basic string = %s\r", ctime(&seconds)); 00041 * wait(1); 00042 * } 00043 * } 00044 * @endcode 00045 * 00046 * See for example http://mbed.org/handbook/Time for general usage 00047 * of C time functions. 00048 * 00049 * Trickle charging is not supported 00050 **/ 00051 class DS1302 00052 { 00053 public: 00054 /** 00055 * Register map 00056 */ 00057 enum { 00058 Seconds = 0x80, 00059 Minutes = 0x82, 00060 Hours = 0x84, 00061 Dates = 0x86, 00062 Months = 0x88, 00063 Days = 0x8A, 00064 Years = 0x8C, 00065 WriteProtect = 0x8E, 00066 Charge = 0x90, 00067 ClockBurst = 0xBE, 00068 RAMBase = 0xC0 00069 }; 00070 00071 /** 00072 * Create a new DS1302 object 00073 * 00074 * @param SCLK the pin to which SCLK is connectd 00075 * @param IO the pin to which IO is connectd 00076 * @param CE the pin to which CE is connected (also called RST) 00077 */ 00078 DS1302(PinName SCLK, PinName IO, PinName CE); 00079 00080 /** Set the current time 00081 * 00082 * Initialises and sets the time of the DS1302 00083 * to the time represented by the number of seconds since January 1, 1970 00084 * (the UNIX timestamp). 00085 * 00086 * @param t Number of seconds since January 1, 1970 (the UNIX timestamp) 00087 * 00088 */ 00089 void set_time(time_t t); 00090 00091 /** Get the current time 00092 * 00093 * Use other functions to convert this value, see: http://mbed.org/handbook/Time 00094 * 00095 * @param t ignored, supply NULL 00096 * @return number of seconds since January 1, 1970 00097 */ 00098 time_t time(time_t *t = NULL); 00099 00100 /** 00101 * Store a byte in the battery-backed RAM 00102 * 00103 * @param address address where to store the data (0-30) 00104 * @param data the byte to store 00105 */ 00106 void storeByte(char address, char data); 00107 00108 /** 00109 * Recall a byte from the battery-backed RAM 00110 * 00111 * @param address address where to retrieve the data (0-30) 00112 * @return the stored data 00113 */ 00114 char recallByte(char address); 00115 00116 /** 00117 * Read a register 00118 * 00119 * Only required to for example manually set trickle charge register 00120 * 00121 * @param reg register to read 00122 * @return contents of the register 00123 */ 00124 char readReg(char reg); 00125 00126 /** 00127 * Write a register 00128 * 00129 * Only required to for example manually set trickle charge register 00130 * 00131 * @param reg register to write 00132 * @param val contents of the register to write 00133 */ 00134 void writeReg(char reg, char val); 00135 00136 protected: 00137 00138 void writeByte(char data); 00139 char readByte(void); 00140 00141 DigitalOut _SCLK; 00142 DigitalInOut _IO; 00143 DigitalOut _CE; 00144 bool writeProtect; 00145 00146 00147 }; 00148 00149 00150 00151 #endif
Generated on Tue Jul 12 2022 18:57:19 by
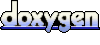