Inherit from SoftSerial and use software buffers for TX and RX. This allows the SoftSerial to operate in a IRQ driven mode. Overrides most (but not all) stdio functions as SoftSerial did
Dependencies: Buffer SoftSerial
Dependents: 2014_Ensoul_Capstone F103RB_tcp_rtu_modbus_copy_v1_0 SDP_Testing Nucleo_SFM ... more
Fork of BufferedSerial by
BufferedSoftSerial.h
00001 00002 /** 00003 * @file BufferedSoftSerial.h 00004 * @brief Software Buffer - Extends mbed Serial functionallity adding irq driven TX and RX 00005 * @author sam grove 00006 * @version 1.0 00007 * @see 00008 * 00009 * Copyright (c) 2013 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); 00012 * you may not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, 00019 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 */ 00023 00024 #ifndef BUFFEREDSOFTSERIAL_H 00025 #define BUFFEREDSOFTSERIAL_H 00026 00027 #include "mbed.h" 00028 #include "Buffer.h" 00029 #include "SoftSerial.h" 00030 00031 /** A serial port (UART) for communication with other serial devices 00032 * 00033 * Can be used for Full Duplex communication, or Simplex by specifying 00034 * one pin as NC (Not Connected) 00035 * 00036 * This uses software serial emulation, regular serial pins are alot better, 00037 * however if you don't have spare ones, you can use this. It is advicable 00038 * to put the serial connection with highest speed to hardware serial. 00039 * 00040 * If you lack RAM memory you can also use SoftSerial without this buffer around it. 00041 * In that case it is fully blocking. 00042 * 00043 * Example: 00044 * @code 00045 * #include "mbed.h" 00046 * #include "BufferedSoftSerial.h" 00047 * 00048 * SoftSerial block(USBTX, USBRX); 00049 * BufferedSoftSerial buf(USBTX, USBRX); 00050 * 00051 * int main() 00052 * { 00053 * while(1) { 00054 * Timer s; 00055 * 00056 * s.start(); 00057 * buf.printf("Hello World - buffered\r\n"); 00058 * int buffered_time = s.read_us(); 00059 * wait(0.1f); // give time for the buffer to empty 00060 * 00061 * s.reset(); 00062 * block.printf("Hello World - blocking\r\n"); 00063 * int polled_time = s.read_us(); 00064 * s.stop(); 00065 * wait(0.1f); // give time for the buffer to empty 00066 * 00067 * buf.printf("printf buffered took %d us\r\n", buffered_time); 00068 * buf.printf("printf blocking took %d us\r\n", polled_time); 00069 * wait(5); 00070 * } 00071 * } 00072 * @endcode 00073 */ 00074 00075 /** 00076 * @class BufferedSerial 00077 * @brief Software buffers and interrupt driven tx and rx for SoftSerial 00078 */ 00079 class BufferedSoftSerial : public SoftSerial 00080 { 00081 private: 00082 Buffer <char> _rxbuf; 00083 Buffer <char> _txbuf; 00084 00085 void rxIrq(void); 00086 void txIrq(void); 00087 void prime(void); 00088 00089 public: 00090 /** Create a BufferedSoftSerial port, connected to the specified transmit and receive pins 00091 * @param tx Transmit pin 00092 * @param rx Receive pin 00093 * @note Either tx or rx may be specified as NC if unused 00094 */ 00095 BufferedSoftSerial(PinName tx, PinName rx, const char* name=NULL); 00096 00097 /** Check on how many bytes are in the rx buffer 00098 * @return 1 if something exists, 0 otherwise 00099 */ 00100 virtual int readable(void); 00101 00102 /** Check to see if the tx buffer has room 00103 * @return 1 always has room and can overwrite previous content if too small / slow 00104 */ 00105 virtual int writeable(void); 00106 00107 /** Get a single byte from the BufferedSoftSerial Port. 00108 * Should check readable() before calling this. 00109 * @return A byte that came in on the BufferedSoftSerial Port 00110 */ 00111 virtual int getc(void); 00112 00113 /** Write a single byte to the BufferedSoftSerial Port. 00114 * @param c The byte to write to the BufferedSoftSerial Port 00115 * @return The byte that was written to the BufferedSoftSerial Port Buffer 00116 */ 00117 virtual int putc(int c); 00118 00119 /** Write a string to the BufferedSoftSerial Port. Must be NULL terminated 00120 * @param s The string to write to the Serial Port 00121 * @return The number of bytes written to the Serial Port Buffer 00122 */ 00123 virtual int puts(const char *s); 00124 00125 /** Write a formatted string to the BufferedSoftSerial Port. 00126 * @param format The string + format specifiers to write to the BufferedSoftSerial Port 00127 * @return The number of bytes written to the Serial Port Buffer 00128 */ 00129 virtual int printf(const char* format, ...); 00130 00131 /** Write data to the BufferedSoftSerial Port 00132 * @param s A pointer to data to send 00133 * @param length The amount of data being pointed to 00134 * @return The number of bytes written to the Serial Port Buffer 00135 */ 00136 virtual ssize_t write(const void *s, std::size_t length); 00137 }; 00138 00139 #endif
Generated on Wed Jul 13 2022 04:41:52 by
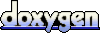