
Embed:
(wiki syntax)
Show/hide line numbers
spilcd.h
00001 // AD-12684-SPI control class 00002 // version 0.1 00003 // created by Sim (http://mbed.org/users/Sim/) 00004 // 00005 // About AD-12864-SPI, see http://www.aitendo.co.jp/product/1622 . 00006 00007 #ifndef __SPILCD_H__ 00008 #define __SPILCD_H__ 00009 00010 #include "mbed.h" 00011 00012 class SPILCD { 00013 private: 00014 DigitalOut cs, rst, a0; 00015 SPI spi; 00016 00017 // boot up sequence 00018 void init(){ 00019 spi.format(8,0); // nazo 00020 spi.frequency(20000000); // modify later 00021 00022 // reset 00023 wait_ms(10); 00024 rst = 0; 00025 wait_us(1); 00026 rst = 1; 00027 wait_us(1); 00028 00029 // initialize sequence 00030 cmd(0xa2); // (11) LCD Bias Set ... 1/9 bias (see 2.4.11) 00031 cmd(0xa0); // (8) ADC Select ... normal (see 2.4.8) 00032 cmd(0xc8); // (15) Common Output Mode Select ... Reverse (see 2.4.15) 00033 cmd(0x24); // (17) V5 Volatge Regulator Internal Resister Ratio Set (see 2.4.17) 00034 cmd(0x81); // (18) set electronic volume mode (see 2.4.18) 00035 cmd(0x1e); // electronic volume data 00-3f ... control your own value 00036 cmd(0x2f); // (16) power control set (see 2.4.16) 00037 cmd(0x40); // (2) Display Start Line Set ... 0 (see 2.4.2) 00038 cmd(0xe0); // (6) Write Mode Set 00039 cmd(0xaf); // (1) display on (see 2.4.1) 00040 } 00041 00042 public: 00043 00044 // constructor 00045 SPILCD(PinName cs_pin, PinName rst_pin, PinName a0_pin, PinName mosi_pin, PinName miso_pin, PinName sclk_pin) 00046 : cs(cs_pin), rst(rst_pin), a0(a0_pin), spi(mosi_pin, miso_pin, sclk_pin) { 00047 00048 init(); 00049 cls(); 00050 } 00051 00052 // wipe all screen 00053 void cls(void){ 00054 int x, y; 00055 for(y = 0; y < 8; y++){ 00056 moveto(0, y); 00057 for(x = 0; x < 128; x++) write(0x00); 00058 } 00059 moveto(0, 0); 00060 } 00061 00062 // move position to (x, 8 * y) 00063 void moveto(int x, int y){ 00064 cmd(0xb0 | (y & 0x0f)); // Page Address Set (see 2.4.3) 00065 cmd(0x10 | (x >> 4 & 0x0f)); // Column Address Set (see 2.4.4) 00066 cmd(x & 0x0f); 00067 } 00068 00069 // write command 00070 void cmd(unsigned char c){ 00071 cs = a0 = 0; 00072 spi.write(c); 00073 cs = 1; 00074 } 00075 00076 // write data 00077 void write(unsigned char c){ 00078 cs = 0; 00079 a0 = 1; 00080 spi.write(c); 00081 cs = 1; 00082 } 00083 }; 00084 00085 #endif
Generated on Tue Jul 12 2022 20:11:35 by
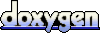