
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "spilcd.h" 00003 #include "kfont8.h" 00004 00005 #define countof(x) ( sizeof(x) / sizeof(x[0]) ) 00006 00007 SPILCD lcd(p21, p22, p23, p11, p12, p13); 00008 00009 int csrx = 0; 00010 int csry = 0; 00011 int offsety = 0; 00012 bool kstate = false; 00013 unsigned char kbuf; 00014 00015 void locate(int x, int y){ 00016 csrx = x; 00017 csry = y; 00018 if(csrx < 0) csrx = 0; 00019 if(csry < 0) csry = 0; 00020 csrx &= 31; 00021 csry &= 7; 00022 lcd.moveto(csrx << 3, (offsety + csry) & 7); 00023 }; 00024 00025 void scroll(void){ 00026 int i; 00027 offsety = (offsety + 1) & 7; 00028 lcd.cmd(0x40 | offsety << 3); 00029 lcd.moveto(0, (offsety + 7) & 7); 00030 for(i = 0; i < 128; i++) lcd.write(0); 00031 } 00032 00033 void newline(void){ 00034 csrx = 0; 00035 if(++csry == 8){ 00036 scroll(); 00037 csry = 7; 00038 } 00039 locate(csrx, csry); 00040 } 00041 00042 // draw 4x8 font 00043 void drawfont4(unsigned char c){ 00044 const unsigned char *p = &font4[c << 2]; 00045 lcd.write(p[0]); 00046 lcd.write(p[1]); 00047 lcd.write(p[2]); 00048 lcd.write(p[3]); 00049 // cursor control 00050 if(++csrx == 32) newline(); 00051 } 00052 00053 const unsigned char *findface(unsigned short c){ 00054 const unsigned char *p = NULL; 00055 int i, sum; 00056 for(sum = i = 0; i < countof(font8table); i++){ 00057 if(font8table[i].start <= c && c <= font8table[i].end){ 00058 p = &font8[(sum + c - font8table[i].start) << 3]; 00059 break; 00060 } 00061 sum += font8table[i].end - font8table[i].start + 1; 00062 } 00063 return p; 00064 } 00065 00066 // draw 8x8 font 00067 void drawkanji(unsigned short c){ 00068 const unsigned char *p = findface(c); 00069 if(p == NULL) return; 00070 00071 if(csrx >= 31) newline(); 00072 lcd.write(p[0]); 00073 lcd.write(p[1]); 00074 lcd.write(p[2]); 00075 lcd.write(p[3]); 00076 lcd.write(p[4]); 00077 lcd.write(p[5]); 00078 lcd.write(p[6]); 00079 lcd.write(p[7]); 00080 csrx += 2; 00081 if(csrx == 32) newline(); 00082 } 00083 00084 void drawc(unsigned char c){ 00085 if(kstate){ // 2nd byte of shift-jis 00086 kstate = false; 00087 drawkanji(kbuf << 8 | c); 00088 } else if((0x81 <= c && c <= 0x9f) || (0xe0 <= c && c <= 0xfc)){ // 1st byte of shift-jis 00089 kstate = true; 00090 kbuf = c; 00091 } else { // 4x8font 00092 drawfont4(c); 00093 } 00094 } 00095 00096 void draws(const unsigned char *s){ 00097 unsigned char c; 00098 while((c = *s++) != '\0') drawc(c); 00099 } 00100 00101 Serial sio(USBTX, USBRX); 00102 00103 int main() { 00104 unsigned char c; 00105 00106 sio.baud(115200); 00107 while(1){ 00108 c = sio.getc(); 00109 if(c == 0x0d){ 00110 newline(); 00111 } else { 00112 drawc(c); 00113 } 00114 } 00115 }
Generated on Tue Jul 12 2022 20:11:35 by
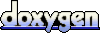