fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
core_cm0.c
00001 /**************************************************************************//** 00002 * @file core_cm0.c 00003 * @brief CMSIS Cortex-M0 Core Peripheral Access Layer Source File 00004 * @version V2.00 00005 * @date 10. September 2010 00006 * 00007 * @note 00008 * Copyright (C) 2009-2010 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #include <stdint.h> 00025 00026 /* define compiler specific symbols */ 00027 #if defined ( __CC_ARM ) 00028 #define __ASM __asm /*!< asm keyword for ARM Compiler */ 00029 #define __INLINE __inline /*!< inline keyword for ARM Compiler */ 00030 00031 #elif defined ( __ICCARM__ ) 00032 #define __ASM __asm /*!< asm keyword for IAR Compiler */ 00033 #define __INLINE inline /*!< inline keyword for IAR Compiler. Only avaiable in High optimization mode! */ 00034 00035 #elif defined ( __GNUC__ ) 00036 #define __ASM __asm /*!< asm keyword for GNU Compiler */ 00037 #define __INLINE inline /*!< inline keyword for GNU Compiler */ 00038 00039 #elif defined ( __TASKING__ ) 00040 #define __ASM __asm /*!< asm keyword for TASKING Compiler */ 00041 #define __INLINE inline /*!< inline keyword for TASKING Compiler */ 00042 00043 #endif 00044 00045 00046 /* ########################## Core Instruction Access ######################### */ 00047 00048 #if defined ( __CC_ARM ) /*------------------ RealView Compiler ----------------*/ 00049 00050 /** \brief Reverse byte order (16 bit) 00051 00052 This function reverses the byte order in two unsigned short values. 00053 00054 \param [in] value Value to reverse 00055 \return Reversed value 00056 */ 00057 #if (__ARMCC_VERSION < 400677) 00058 __ASM uint32_t __REV16(uint32_t value) 00059 { 00060 rev16 r0, r0 00061 bx lr 00062 } 00063 #endif /* __ARMCC_VERSION */ 00064 00065 00066 /** \brief Reverse byte order in signed short value 00067 00068 This function reverses the byte order in a signed short value with sign extension to integer. 00069 00070 \param [in] value Value to reverse 00071 \return Reversed value 00072 */ 00073 #if (__ARMCC_VERSION < 400677) 00074 __ASM int32_t __REVSH(int32_t value) 00075 { 00076 revsh r0, r0 00077 bx lr 00078 } 00079 #endif /* __ARMCC_VERSION */ 00080 00081 00082 /** \brief Remove the exclusive lock 00083 00084 This function removes the exclusive lock which is created by LDREX. 00085 00086 */ 00087 #if (__ARMCC_VERSION < 400000) 00088 __ASM void __CLREX(void) 00089 { 00090 clrex 00091 } 00092 #endif /* __ARMCC_VERSION */ 00093 00094 00095 #elif (defined (__ICCARM__)) /*---------------- ICC Compiler ---------------------*/ 00096 /* obsolete */ 00097 #elif (defined (__GNUC__)) /*------------------ GNU Compiler ---------------------*/ 00098 /* obsolete */ 00099 #elif (defined (__TASKING__)) /*--------------- TASKING Compiler -----------------*/ 00100 /* obsolete */ 00101 #endif 00102 00103 00104 /* ########################### Core Function Access ########################### */ 00105 00106 #if defined ( __CC_ARM ) /*------------------ RealView Compiler ----------------*/ 00107 00108 /** \brief Get Control Register 00109 00110 This function returns the content of the Control Register. 00111 00112 \return Control Register value 00113 */ 00114 #if (__ARMCC_VERSION < 400000) 00115 __ASM uint32_t __get_CONTROL(void) 00116 { 00117 mrs r0, control 00118 bx lr 00119 } 00120 #endif /* __ARMCC_VERSION */ 00121 00122 00123 /** \brief Set Control Register 00124 00125 This function writes the given value to the Control Register. 00126 00127 \param [in] control Control Register value to set 00128 */ 00129 #if (__ARMCC_VERSION < 400000) 00130 __ASM void __set_CONTROL(uint32_t control) 00131 { 00132 msr control, r0 00133 bx lr 00134 } 00135 #endif /* __ARMCC_VERSION */ 00136 00137 00138 /** \brief Get ISPR Register 00139 00140 This function returns the content of the ISPR Register. 00141 00142 \return ISPR Register value 00143 */ 00144 #if (__ARMCC_VERSION < 400000) 00145 __ASM uint32_t __get_IPSR(void) 00146 { 00147 mrs r0, ipsr 00148 bx lr 00149 } 00150 #endif /* __ARMCC_VERSION */ 00151 00152 00153 /** \brief Get APSR Register 00154 00155 This function returns the content of the APSR Register. 00156 00157 \return APSR Register value 00158 */ 00159 #if (__ARMCC_VERSION < 400000) 00160 __ASM uint32_t __get_APSR(void) 00161 { 00162 mrs r0, apsr 00163 bx lr 00164 } 00165 #endif /* __ARMCC_VERSION */ 00166 00167 00168 /** \brief Get xPSR Register 00169 00170 This function returns the content of the xPSR Register. 00171 00172 \return xPSR Register value 00173 */ 00174 #if (__ARMCC_VERSION < 400000) 00175 __ASM uint32_t __get_xPSR(void) 00176 { 00177 mrs r0, xpsr 00178 bx lr 00179 } 00180 #endif /* __ARMCC_VERSION */ 00181 00182 00183 /** \brief Get Process Stack Pointer 00184 00185 This function returns the current value of the Process Stack Pointer (PSP). 00186 00187 \return PSP Register value 00188 */ 00189 #if (__ARMCC_VERSION < 400000) 00190 __ASM uint32_t __get_PSP(void) 00191 { 00192 mrs r0, psp 00193 bx lr 00194 } 00195 #endif /* __ARMCC_VERSION */ 00196 00197 00198 /** \brief Set Process Stack Pointer 00199 00200 This function assigns the given value to the Process Stack Pointer (PSP). 00201 00202 \param [in] topOfProcStack Process Stack Pointer value to set 00203 */ 00204 #if (__ARMCC_VERSION < 400000) 00205 __ASM void __set_PSP(uint32_t topOfProcStack) 00206 { 00207 msr psp, r0 00208 bx lr 00209 } 00210 #endif /* __ARMCC_VERSION */ 00211 00212 00213 /** \brief Get Main Stack Pointer 00214 00215 This function returns the current value of the Main Stack Pointer (MSP). 00216 00217 \return MSP Register value 00218 */ 00219 #if (__ARMCC_VERSION < 400000) 00220 __ASM uint32_t __get_MSP(void) 00221 { 00222 mrs r0, msp 00223 bx lr 00224 } 00225 #endif /* __ARMCC_VERSION */ 00226 00227 00228 /** \brief Set Main Stack Pointer 00229 00230 This function assigns the given value to the Main Stack Pointer (MSP). 00231 00232 \param [in] topOfMainStack Main Stack Pointer value to set 00233 */ 00234 #if (__ARMCC_VERSION < 400000) 00235 __ASM void __set_MSP(uint32_t mainStackPointer) 00236 { 00237 msr msp, r0 00238 bx lr 00239 } 00240 #endif /* __ARMCC_VERSION */ 00241 00242 00243 /** \brief Get Priority Mask 00244 00245 This function returns the current state of the priority mask bit from the Priority Mask Register. 00246 00247 \return Priority Mask value 00248 */ 00249 #if (__ARMCC_VERSION < 400000) 00250 __ASM uint32_t __get_PRIMASK(void) 00251 { 00252 mrs r0, primask 00253 bx lr 00254 } 00255 #endif /* __ARMCC_VERSION */ 00256 00257 00258 /** \brief Set Priority Mask 00259 00260 This function assigns the given value to the Priority Mask Register. 00261 00262 \param [in] priMask Priority Mask 00263 */ 00264 #if (__ARMCC_VERSION < 400000) 00265 __ASM void __set_PRIMASK(uint32_t priMask) 00266 { 00267 msr primask, r0 00268 bx lr 00269 } 00270 #endif /* __ARMCC_VERSION */ 00271 00272 00273 #elif (defined (__ICCARM__)) /*---------------- ICC Compiler ---------------------*/ 00274 /* obsolete */ 00275 #elif (defined (__GNUC__)) /*------------------ GNU Compiler ---------------------*/ 00276 /* obsolete */ 00277 #elif (defined (__TASKING__)) /*--------------- TASKING Compiler -----------------*/ 00278 /* obsolete */ 00279 #endif
Generated on Tue Jul 12 2022 13:47:00 by
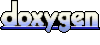