fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
port_api.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "port_api.h" 00017 #include "pinmap.h" 00018 #include "gpio_api.h" 00019 00020 PinName port_pin(PortName port, int pin_n) { 00021 return (PinName)((port << PORT_SHIFT) | (pin_n << 2)); 00022 } 00023 00024 void port_init(port_t *obj, PortName port, int mask, PinDirection dir) { 00025 obj->port = port; 00026 obj->mask = mask; 00027 00028 FGPIO_Type *reg = (FGPIO_Type *)(FPTA_BASE + port * 0x40); 00029 00030 obj->reg_out = ®->PDOR; 00031 obj->reg_in = ®->PDIR; 00032 obj->reg_dir = ®->PDDR; 00033 00034 uint32_t i; 00035 // The function is set per pin: reuse gpio logic 00036 for (i=0; i<32; i++) { 00037 if (obj->mask & (1<<i)) { 00038 gpio_set(port_pin(obj->port, i)); 00039 } 00040 } 00041 00042 port_dir(obj, dir); 00043 } 00044 00045 void port_mode(port_t *obj, PinMode mode) { 00046 uint32_t i; 00047 // The mode is set per pin: reuse pinmap logic 00048 for (i=0; i<32; i++) { 00049 if (obj->mask & (1<<i)) { 00050 pin_mode(port_pin(obj->port, i), mode); 00051 } 00052 } 00053 } 00054 00055 void port_dir(port_t *obj, PinDirection dir) { 00056 switch (dir) { 00057 case PIN_INPUT : *obj->reg_dir &= ~obj->mask; break; 00058 case PIN_OUTPUT: *obj->reg_dir |= obj->mask; break; 00059 } 00060 } 00061 00062 void port_write(port_t *obj, int value) { 00063 *obj->reg_out = (*obj->reg_in & ~obj->mask) | (value & obj->mask); 00064 } 00065 00066 int port_read(port_t *obj) { 00067 return (*obj->reg_in & obj->mask); 00068 }
Generated on Tue Jul 12 2022 13:47:01 by
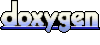