
JDI_MIP on ThunderBoardSense2(Silicon-Labs)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
Go to the documentation of this file.
00001 /***************************************************************************//** 00002 * @file main.cpp 00003 ******************************************************************************* 00004 * @section License 00005 * <b>(C) Copyright 2017 Silicon Labs, http://www.silabs.com</b> 00006 ******************************************************************************* 00007 * 00008 * SPDX-License-Identifier: Apache-2.0 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00011 * not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00018 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 * 00022 ******************************************************************************/ 00023 00024 #include <string> 00025 #include "mbed.h" 00026 #include "BMP280/BMP280.h" 00027 #include "AMS_CCS811.h" 00028 #include "Si7021.h" 00029 #include "Si7210.h" 00030 #include "Si1133.h " 00031 #include "ICM20648.h " 00032 00033 #include "SPI_MIP16.h" 00034 #include "data.h" 00035 #include "HGP23x29.h" 00036 #include "HGP15x19.h" 00037 00038 00039 /* Turn on power supply to ENV sensor suite */ 00040 DigitalOut env_en(PF9, 1); 00041 /* Turn on power to CCS811 sensor */ 00042 DigitalOut ccs_en(PF14, 1); 00043 /* Set CCS_WAKE pin to output */ 00044 DigitalOut ccs_wake(PF15, 1); 00045 /* Turn on power to hall effect sensor */ 00046 DigitalOut hall_en(PB10, 1); 00047 /* Turn on power to IMU */ 00048 DigitalOut imu_en(PF8, 1); 00049 00050 I2C ccs_i2c(PB6, PB7); 00051 I2C hall_i2c(PB8, PB9); 00052 I2C env_i2c(PC4, PC5); 00053 00054 // MIP 00055 WatchDisp WD(PK0,PK2,PF7,PA5); //PTC12); // mosi,miso,clk,cs,disp,power(EXTMODE) 00056 DigitalOut _lcd_disp(PA8); // DISP 00057 DigitalOut _lcd_extmod(PF6); // EXTMODE L=SERIAL, H=EXTCOMIN Signal 00058 DigitalOut _lcd_extcom(PA9); // Togle 00059 00060 uint8_t ccolor_table[] = {Black,Gray,Blue ,LightBlue ,Green ,LightGreen ,Cyan ,LightCyan, 00061 Red ,pink,Magenta,LightMagenta,Yellow,LightYellow,LightGray,White}; 00062 DigitalOut _pk1(PK1,1); // QSPI Flash CS Disable 00063 00064 bool if16 = 0; 00065 bool ifMargeTXT = 0; 00066 int width =176; 00067 int height=176; 00068 /////////////////////////////////////// 00069 int disp_logo(void) 00070 { 00071 int RGB; 00072 uint8_t R8,G8,B8; 00073 uint8_t *cptr; 00074 //for(int i=0; i< 54 ; i++) fscanf(fp,"%c",DUMMY); // Discard Header 54bytes 00075 cptr = (uint8_t *)&Title_bmp[54]; 00076 for(int y=height-1; y>=0; y--) { 00077 for(int x=0; x< width; x++) { //24bit color B 8bit -> G 8bit -> R 8bit 00078 B8 = *cptr++; 00079 G8 = *cptr++; 00080 R8 = *cptr++; 00081 RGB = RGB8(R8,G8,B8); //6bit(8bit) MIP MASK 0000 1110 00082 WD.pixel(x,y,RGB); 00083 } 00084 //if( y!=0) // The last data column doesn't need padding 00085 //for(int x=(width*3)%4; (x%4 !=0); x++) fscanf(fp, "%c",DUMMY); // 4byte boundery for every column 00086 } 00087 } 00088 00089 int lcd_init(void) 00090 { 00091 int i; 00092 // Power On Sequece 00093 // _lcd_reset = 0; 00094 // _lcd_reset = 1; 00095 _lcd_extmod = 0; 00096 00097 WD.setWH(width,height); // Default X/Y-00 00098 bool if16 = 1; 00099 WD.set16col(if16); // 0= RGB, 1=RGBW 00100 WD.setmarge(1); 00101 WD.background(Cyan); 00102 WD.clsBUF(); 00103 _lcd_disp = 1; // 00104 //WD.fillrect(i*(width/16), height/2+1, (i+1)*(width/16)-1, height , ccolor_table[15-i]); 00105 //WD.writeDISP(); 00106 00107 //WD.set_font((unsigned char*) HGP23x29); 00108 //WD.locate( 0, 43);WD.foreground(Blue); WD.printf("Environmental"); 00109 //WD.locate( 0, 83);WD.foreground(Blue); WD.printf(" Monitor"); 00110 //WD.locate(120,140);WD.foreground(Red ); WD.printf("JDI"); 00111 disp_logo(); 00112 WD.writeDISP(); 00113 _lcd_extmod = 1; // 1=Pin,0=Serial 00114 WD.background(Cyan); 00115 return 0; 00116 /**/ 00117 } 00118 00119 /////////////////////////////////////////////// 00120 int main() { 00121 uint32_t eco2, tvoc; 00122 float pressure, temperature2; 00123 int32_t temperature; 00124 uint32_t humidity; 00125 float light, uv; 00126 float acc_x, acc_y, acc_z, gyr_x, gyr_y, gyr_z, temperature3; 00127 00128 bool lightsensor_en = true; 00129 bool gassensor_en = true; 00130 bool hallsensor_en = true; 00131 bool rhtsensor_en = true; 00132 bool pressuresensor_en = true; 00133 bool imu_en = false; 00134 00135 _pk1=1; 00136 lcd_init(); 00137 ccs_en = 0; wait_ms(100); 00138 ccs_en = 1; 00139 wait_ms(2000); 00140 00141 /* Initialize air quality sensor */ 00142 AMS_CCS811* gasSensor = new AMS_CCS811(&ccs_i2c, PF15); 00143 if(!gasSensor->init()) { 00144 printf("Failed CCS811 init\r\n"); 00145 gassensor_en = false; 00146 } else { 00147 if(!gasSensor->mode(AMS_CCS811::TEN_SECOND)) { 00148 printf("Failed to set CCS811 mode\r\n"); 00149 gassensor_en = false; 00150 } 00151 } 00152 00153 wait_ms(10); 00154 00155 /* Initialize barometer and RHT */ 00156 BMP280* pressureSensor = new BMP280(env_i2c); 00157 Si7021* rhtSensor = new Si7021(PC4, PC5); 00158 00159 printf("\r\n\r\nHello Thunderboard Sense 2...\r\n"); 00160 00161 /* Check if hall sensor is alive */ 00162 silabs::Si7210* hallSensor = new silabs::Si7210(&hall_i2c); 00163 uint8_t id; 00164 hallSensor->wakeup(); 00165 hallSensor->readRegister(SI72XX_HREVID, &id); 00166 printf("Hall ID: %d\r\n", id); 00167 00168 /* Initialize light sensor */ 00169 Si1133* lightSensor = new Si1133(PC4, PC5); 00170 00171 if(!lightSensor->open()) { 00172 printf("Something is wrong with Si1133, disabling...\n"); 00173 lightsensor_en = false; 00174 } else printf("Si1133 available\n\r"); 00175 00176 /* Initialize the IMU*/ 00177 printf("ICM20648 Start \n\r"); 00178 00179 ICM20648* imu = new ICM20648(PC0, PC1, PC2, PC3, PF12); 00180 printf(" ICM20648 OK\n\r"); 00181 00182 if (imu != 0){ 00183 if(!imu->open()) { 00184 printf("Something is wrong with ICM20648, disabling...\n"); 00185 imu_en = false; 00186 } else printf("ICM20648 available\n\r"); 00187 } 00188 WD.foreground(Red); 00189 WD.set_font((unsigned char*) HGP15x19); 00190 #define WD_OFFSET 10 00191 do { 00192 WD.clsBUF(); 00193 printf("----------------------------------------------\r\n"); 00194 /* Measure temperature and humidity */ 00195 if(rhtsensor_en) { 00196 rhtSensor->measure(); 00197 rhtSensor->measure(); 00198 temperature = rhtSensor->get_temperature(); 00199 humidity = rhtSensor->get_humidity(); 00200 00201 printf("temperature and humidity(Si7021):\r\n"); 00202 printf("temperature: %ld.%03ld degC\r\n", temperature/1000, abs(temperature%1000)); 00203 printf("humidity : %ld.%03ld %%\r\n", humidity/1000, humidity%1000); 00204 WD.locate( 2,0*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("Temp."); 00205 WD.locate(55,0*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%ld.%03ld degC", temperature/1000, abs(temperature%1000)); 00206 WD.locate( 2,1*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("Humi."); 00207 WD.locate(55,1*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%ld.%03ld %%", humidity/1000, humidity%1000); 00208 } 00209 00210 /* Measure barometric pressure */ 00211 if(pressuresensor_en) { 00212 temperature2 = pressureSensor->getTemperature(); 00213 pressure = pressureSensor->getPressure(); 00214 00215 printf("pressure(BMP280):\r\n"); 00216 printf("pressure : %.2f bar\r\n", pressure); 00217 printf("temperature: %.2f degC\r\n", temperature2); 00218 WD.locate( 2,2*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("Pres."); 00219 WD.locate(55,2*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%.2f bar\r\n", pressure); 00220 } 00221 00222 /* Measure air quality */ 00223 if(gassensor_en) { 00224 gasSensor->has_new_data(); 00225 eco2 = gasSensor->co2_read(); 00226 tvoc = gasSensor->tvoc_read(); 00227 00228 printf("air quality(CCS811):\r\n"); 00229 printf("CO2: %ld ppm\r\n", eco2); 00230 printf("VoC: %ld ppb\r\n", tvoc); 00231 WD.locate( 2,3*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("CO2"); 00232 WD.locate(55,3*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%ld ppm", eco2); 00233 WD.locate( 2,4*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("VoC"); 00234 WD.locate(55,4*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%ld ppb", tvoc); 00235 } 00236 00237 /* measure HALL */ 00238 if(hallsensor_en) { 00239 hallSensor->measureOnce(); 00240 printf("HALL(Si7210):\r\n"); 00241 printf("T: %d.%02d degC\r\n", hallSensor->getTemperature()/1000, abs(hallSensor->getTemperature()%1000)); 00242 printf("F: %i.%03d mT\r\n", (int)(hallSensor->getFieldStrength()/1000), abs(hallSensor->getFieldStrength() % 1000)); 00243 WD.locate( 2,5*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("Magn."); 00244 WD.locate(55,5*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%i.%03d mT\r\n", (int)(hallSensor->getFieldStrength()/1000), abs(hallSensor->getFieldStrength() % 1000)); 00245 } 00246 00247 /* measure light */ 00248 if(lightsensor_en) { 00249 lightSensor->get_light_and_uv(&light, &uv); 00250 00251 printf("Light(BMP280):\r\n"); 00252 printf("Light: %.2f lux\r\n", light); 00253 printf("UV : %.2f \r\n", uv); 00254 WD.locate( 2,6*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("Lumi."); 00255 WD.locate(55,6*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%.2f lux", light); 00256 WD.locate( 2,7*20+WD_OFFSET);WD.foreground(Blue ); WD.printf("UV"); 00257 WD.locate(55,7*20+WD_OFFSET);WD.foreground(Red ); WD.printf("%.2f", uv); 00258 } 00259 00260 /* measure imu */ 00261 if(imu_en) { 00262 imu->get_temperature(&temperature3); 00263 imu->get_accelerometer(&acc_x, &acc_y, &acc_z); 00264 imu->get_gyroscope(&gyr_x, &gyr_y, &gyr_z); 00265 00266 printf("ICM20648:\r\n"); 00267 printf("TEMP: %.2f degC\r\n", temperature3); 00268 printf("Acc : %.2f %.2f %.2f\r\n", acc_x, acc_y, acc_z); 00269 printf("Gyro: %.2f %.2f %.2f\r\n", gyr_x, gyr_y, gyr_z); 00270 WD.locate(2,8*20+WD_OFFSET); WD.printf("Acc : %.2f %.2f %.2f\r\n", acc_x, acc_y, acc_z); 00271 WD.locate(2,9*20+WD_OFFSET); WD.printf("Gyro: %.2f %.2f %.2f\r\n", gyr_x, gyr_y, gyr_z); 00272 } 00273 WD.writeDISP(); 00274 _lcd_extcom = !_lcd_extcom; 00275 wait(1); 00276 } while(1); 00277 }
Generated on Sat Jul 16 2022 02:12:35 by
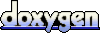