
JDI_MIP on ThunderBoardSense2(Silicon-Labs)
Embed:
(wiki syntax)
Show/hide line numbers
SPI_MIP16.h
00001 /* mbed WatchDISP Library 00002 * Copyright (c) 2014 T.okamoto 00003 * Version 0.1 (19, September, 2014) 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 #include "mbed.h" 00008 //#define FRAME_SIZE 9328 //1flame = 212dot X 88dot 1dot = 4bit 00009 #define FRAME_SIZE 27824 //1flame = 296dot X 188dot 1dot = 4bit 00010 00011 // RGBW color definitions /* R, G, B, W */ 00012 #define Black 0x00 /* 0 0 0 0 */ 00013 #define Gray 0x01 /* 0 0 0 1 */ 00014 #define Blue 0x02 /* 0 0 1 0 */ 00015 #define LightBlue 0x03 /* 0 0 1 1 */ 00016 #define Green 0x04 /* 0 1 0 0 */ 00017 #define LightGreen 0x05 /* 0 1 0 1 */ 00018 #define Cyan 0x06 /* 0 1 1 0 */ 00019 #define LightCyan 0x07 /* 0 1 1 1 */ 00020 #define Red 0x08 /* 1 0 0 0 */ 00021 #define pink 0x09 /* 1 0 0 1 */ 00022 #define Magenta 0x0a /* 1 0 1 0 */ 00023 #define LightMagenta 0x0b /* 1 0 1 1 */ 00024 #define Yellow 0x0c /* 1 1 0 0 */ 00025 #define LightYellow 0x0d /* 1 1 0 1 */ 00026 #define LightGray 0x0e /* 1 1 1 0 */ 00027 #define White 0x0f /* 1 1 1 1 */ 00028 00029 #define RGB8(r,g,b) (((r & 0x80) >>4) | ((g & 0x80)>>5) | ((b & 0x80)>>6) ) & 0x0E //6bit(8bit) MIP MASK 0000 1110 00030 00031 class WatchDisp : public Stream { 00032 public: 00033 WatchDisp(PinName mosi,PinName miso,PinName sclk,PinName cs); 00034 void writeDISP(void); 00035 void clsBUF(void); 00036 void locate(int x, int y); 00037 void foreground(uint8_t colour); 00038 void background(uint8_t colour); 00039 void command(char command); 00040 void setmarge(bool ifMarge); 00041 void set16col(char if16); 00042 void setWH(int width, int height); 00043 00044 void character(int x, int y, int c); 00045 void pixel(int x, int y, uint8_t color); 00046 void LayerCopy(void); 00047 void circle(int x0, int y0, int r, uint8_t color); 00048 void fillcircle(int x0, int y0, int r, uint8_t color); 00049 void hline(int x0, int x1, int y, uint8_t color); 00050 void vline(int x, int y0, int y1, uint8_t color); 00051 void line(int x0, int y0, int x1, int y1, uint8_t color); 00052 void rect(int x0, int y0, int x1, int y1, uint8_t color); 00053 void fillrect(int x0, int y0, int x1, int y1, uint8_t color); 00054 void Symbol(unsigned int x, unsigned int y, unsigned char *symbol); 00055 void set_font(unsigned char* f); 00056 unsigned char* font; 00057 00058 //#define LCD_SPI 00059 00060 protected: 00061 virtual int _putc(int value); 00062 virtual int _getc(); 00063 #ifdef LCD_SPI 00064 SPI _spi; 00065 #define _spi_write _spi.write 00066 #else 00067 DigitalOut _mosi; 00068 DigitalIn _miso; 00069 DigitalOut _sclk; 00070 void _spi_write(uint8_t command); 00071 #endif 00072 DigitalOut _cs; 00073 00074 char _foreground; 00075 char _background; 00076 bool _ifMarge; 00077 char _if16; 00078 00079 uint8_t _dispBUF[FRAME_SIZE]; 00080 uint8_t _LayerBUF[FRAME_SIZE]; 00081 int _height; 00082 int _width; 00083 unsigned int char_x; 00084 unsigned int char_y; 00085 00086 };
Generated on Sat Jul 16 2022 02:12:35 by
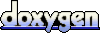