
JDI_MIP on ThunderBoardSense2(Silicon-Labs)
Embed:
(wiki syntax)
Show/hide line numbers
AMS_ENS210.h
00001 /** 00002 * @author Marcus Lee 00003 * 00004 * @section DESCRIPTION 00005 * A library for the AMS ENS210 tempuratire and relative humidy sensor. 00006 * 00007 */ 00008 00009 00010 #ifndef AMS_ENS210_H 00011 #define AMS_ENS210_H 00012 00013 #include "mbed.h" 00014 00015 00016 /* Library defaults */ 00017 #define CONFIG_TEMP_OP_MODE 0 // single shot 00018 #define CONFIG_HUMID_OP_MODE 0 // single shot 00019 #define CONFIG_POWER_MODE 1 // low power 00020 00021 /* Library Constants */ 00022 #define ENS210_SLAVE_ADDR_RAW 0x43 00023 #define ENS210_SLAVE_ADDR ENS210_SLAVE_ADDR_RAW << 1 // 0x86 00024 #define ENS210_SLAVE_ADDR_W ENS210_SLAVE_ADDR 00025 #define ENS210_SLAVE_ADDR_R ENS210_SLAVE_ADDR | 1 // 0x87 00026 00027 #define SYS_CONFIG 0x10 00028 #define SYS_STATUS 0x11 00029 00030 #define SENS_OP_MODE 0x21 00031 #define SENS_START 0x22 00032 #define SENS_STOP 0x23 00033 #define SENS_STATUS 0x24 00034 #define SENS_TEMP 0x30 00035 #define SENS_HUMID 0x33 00036 00037 /** The AMS ENS210 class 00038 */ 00039 class AMS_ENS210 { 00040 00041 public: 00042 /** Create an AMS_ENS210 instance 00043 * 00044 * @param i2c The I2C interface to use for communication 00045 */ 00046 AMS_ENS210(I2C * i2c); 00047 00048 /** Create an AMS_ENS210 instance 00049 * 00050 * @param i2c The I2C interface to use for communication 00051 * @param temp_continuous Set tempurature operation mode, true for continuous and false for single shot 00052 * @param humid_continuous Set humidity operation mode, true for continuous and false for single shot 00053 */ 00054 AMS_ENS210(I2C * i2c, bool temp_continuous, bool humid_continuous); 00055 00056 /** Create an AMS_ENS210 instance 00057 * 00058 * @param i2c The I2C interface to use for communication 00059 * @param temp_continuous Set tempurature operation mode, true for continuous and false for single shot 00060 * @param humid_continuous Set humidity operation mode, true for continuous and false for single shot 00061 * @param low_power Set power mode, true for low power/standby and false for active 00062 */ 00063 AMS_ENS210(I2C * i2c, bool temp_continuous, bool humid_continuous, bool low_power); 00064 00065 /** Destroy the AMS_ENS210 instance 00066 */ 00067 ~AMS_ENS210(); 00068 00069 /** Initalise the sensor 00070 * 00071 * @return Intalisation success 00072 */ 00073 bool init(); 00074 00075 /** Software reset the sensor 00076 * 00077 * @return Reset success 00078 */ 00079 bool reset(); 00080 00081 /** Set the power mode 00082 * 00083 * @param low_power True for low power/standby and false for active 00084 * 00085 * @return Write success 00086 */ 00087 bool low_power_mode(bool low_power); 00088 00089 /** Get the current power mode 00090 * 00091 * @return The power mode, true for low power, false for active 00092 */ 00093 bool low_power_mode(); 00094 00095 /** Get whether the sensor is in the active state or not 00096 * 00097 * @return The active state, true for active, false for inactive 00098 */ 00099 bool is_active(); 00100 00101 /** Set the tempurature operation mode 00102 * 00103 * @param single_shot True for continuous and false for single shot 00104 * 00105 * @return Write success 00106 */ 00107 bool temp_continuous_mode(bool continuous); 00108 00109 /** Get the current tempurature operation mode 00110 * 00111 * @return Write success 00112 */ 00113 bool temp_continuous_mode(); 00114 00115 /** Set the humidity operation mode 00116 * 00117 * @param single_shot True for continuous and false for single shot 00118 * 00119 * @return Write success 00120 */ 00121 bool humid_continuous_mode(bool continuous); 00122 00123 /** Get the current humidity operation mode 00124 * 00125 * @return Write success 00126 */ 00127 bool humid_continuous_mode(); 00128 00129 /** Set the I2C interface 00130 * 00131 * @param i2c The I2C interface 00132 */ 00133 void i2c_interface(I2C * i2c); 00134 00135 /** Get the I2C interface 00136 * 00137 * @return The I2C interface 00138 */ 00139 I2C* i2c_interface(); 00140 00141 /** Start measurement collection 00142 * 00143 * @param temp Start the tempurature sensor 00144 * @param humid Start the humidity sensor 00145 * 00146 * @return Write success 00147 */ 00148 bool start(bool temp = true, bool humid = true); 00149 00150 /** Stop measurement collection 00151 * 00152 * @param temp Stop the tempurature sensor 00153 * @param humid Stop the humidity sensor 00154 * 00155 * @return Write success 00156 */ 00157 bool stop(bool temp = true, bool humid = true); 00158 00159 /** Get whether the sensor is measuring tempurature data 00160 * 00161 * @return Sensor activity, true for measureing, false for idle 00162 */ 00163 bool temp_is_measuring(); 00164 00165 /** Get whether the sensor is measuring humidity data 00166 * 00167 * @return Sensor activity, true for measureing, false for idle 00168 */ 00169 bool humid_is_measuring(); 00170 00171 /** Get whether the sensor has collected tempurature data 00172 * 00173 * @return Has data 00174 */ 00175 bool temp_has_data(); 00176 00177 /** Get whether the sensor has collected humidity data 00178 * 00179 * @return Has data 00180 */ 00181 bool humid_has_data(); 00182 00183 /** Get the most recent tempurature measurement, must call temp_has_data() first when in single shot mode otherwise the same data will be returned 00184 * 00185 * @return Most recent tempurature measurement as a 16 bits unsigned value in 1/64 Kelvin 00186 */ 00187 uint16_t temp_read(); 00188 00189 /** Get the most recent humidty measurement, must call humid_has_data() first when in single shot mode otherwise the same data will be returned 00190 * 00191 * @return Most recent humidity measurement as a 16 bits unsigned value in 1/64 Kelvin 00192 */ 00193 uint16_t humid_read(); 00194 00195 private: 00196 I2C * _i2c; 00197 bool _temp_mode; 00198 bool _humid_mode; 00199 bool _power_mode; 00200 bool _reset; 00201 uint16_t temp_reading; 00202 uint16_t humid_reading; 00203 00204 bool write_config(bool system = true, bool sensor = true); 00205 const char * read_config(bool system = true, bool sensor = true); 00206 int i2c_read(char reg_addr, char* output, int len); 00207 int i2c_write(char reg_addr, char* input, int len); 00208 00209 00210 00211 }; 00212 00213 #endif /* AMS_ENS210_H */
Generated on Sat Jul 16 2022 02:12:35 by
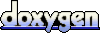