This is library for mcp320x. But operation check is not finished only "mcp3204".
Dependents: Nucleo_mcp3204test
MCP320x.cpp
00001 #include "MCP320x.h" 00002 00003 namespace MCP320x_SPI { 00004 00005 unsigned char CMCP320x_SPI::SPIModuleRefCounter = 0; 00006 00007 CMCP320x_SPI::CMCP320x_SPI(const PinName p_mosi, const PinName p_miso, const PinName p_sclk, const PinName p_cs, const Mcp320xFamilly p_familly, const unsigned int p_frequency) : _internalId("") { 00008 CMCP320x_SPI::SPIModuleRefCounter += 1; 00009 if (CMCP320x_SPI::SPIModuleRefCounter > 1) { 00010 //FIXME Check that SPI settings are identical. Otherwise it should failed 00011 return; 00012 } 00013 00014 _familly = p_familly; 00015 switch (_familly) { 00016 case _3201: 00017 _channelsNum = 1; 00018 break; 00019 case _3204: 00020 _channelsNum = 4; 00021 break; 00022 default: // _3208 00023 _channelsNum = 8; 00024 } // End of 'switch' statement 00025 _settings = 0x02; // SGL/DIFF bit set to 1 = See DS21298E-page 19 TABLE 5-1: CONFIGURATION BITS FOR THE MCP3204/TABLE 5-2: CONFIGURATION BITS FOR THE MCP3208 00026 _spiInstance = new SPI(p_mosi, p_miso, p_sclk); 00027 _spiInstance->frequency(p_frequency); // Set the frequency of the SPI interface 00028 _spiInstance->format(8, 3); 00029 00030 if (p_cs != NC) { 00031 _cs = new DigitalOut(p_cs); 00032 _cs->write(1); // Disable chip 00033 } else { 00034 _cs = NULL; // Not used 00035 } 00036 00037 } 00038 00039 CMCP320x_SPI::~CMCP320x_SPI() { 00040 // Release I2C instance 00041 CMCP320x_SPI::SPIModuleRefCounter -= 1; 00042 if (CMCP320x_SPI::SPIModuleRefCounter == 0) { 00043 delete _spiInstance; 00044 _spiInstance = NULL; 00045 } 00046 // Release _reset if required 00047 if (_cs != NULL) { 00048 _cs->write(1); 00049 delete _cs; 00050 } 00051 } 00052 00053 float CMCP320x_SPI::Read(const Mcp320xChannels p_channels) { 00054 00055 // Read a sample 00056 _sample.value = 0x00; 00057 switch (_familly) { 00058 case _3204: 00059 // No break; 00060 case _3208: 00061 Read_320x(p_channels); 00062 break; 00063 default: // _3201 00064 Read_3201(); 00065 break; 00066 } // End of 'switch' statement 00067 //_sample.value >>= 1; // Adjust composite integer for 12 valid bits 00068 _sample.value &= 0x0FFF; // Mask out upper nibble of integer 00069 00070 return _sample.value; 00071 } 00072 00073 void CMCP320x_SPI::SetConfig(const bool p_settings) { 00074 00075 if (_settings) { 00076 _settings = 0x02; 00077 } else { 00078 _settings = 0x00; 00079 } 00080 } 00081 00082 bool CMCP320x_SPI::Shutdown(const bool p_shutdown) { 00083 // Sanity check 00084 if (_cs == NULL) { 00085 return false; 00086 } 00087 00088 _cs->write(p_shutdown == false ? 0 : 1); 00089 00090 return true; 00091 } 00092 00093 void CMCP320x_SPI::Read_3201() { 00094 if (_cs != NULL) { 00095 _cs->write(0); 00096 wait_us(1); 00097 } 00098 _sample.bytes[1] = _spiInstance->write(0); 00099 _sample.bytes[0] = _spiInstance->write(0); 00100 if (_cs != NULL) { 00101 _cs->write(1); 00102 } 00103 } 00104 00105 void CMCP320x_SPI::Read_320x(const Mcp320xChannels p_channels) { 00106 00107 unsigned char _channels = (unsigned char)p_channels % _channelsNum; 00108 // Set start bit 00109 unsigned char mask = 0x04 | _settings; // Start bit set to 1 - See DS21298E-page 19 Clause 5.0 SERIAL COMMUNICATIONS 00110 // Set channel address 00111 unsigned char cmd0; 00112 unsigned char cmd1; 00113 if (_familly == _3204) { 00114 cmd0 = mask; 00115 cmd1 = _channels << 6; // MCP3204 has 4 channels in single-ended mode 00116 } else { // MCP3208 00117 cmd0 = mask | ((_channels & 0x04) >> 2); // Extract D2 bit - See DS21298E-page 19 Clause 5.0 SERIAL COMMUNICATIONS 00118 cmd1 = _channels << 6; // MCP3204 has 8 channels in single-ended mode 00119 } 00120 if (_cs != NULL) { 00121 _cs->write(0); 00122 wait_us(1); 00123 } 00124 _spiInstance->write(cmd0); // Don't care of the result - See DS21298E-page 21 Clause 6.1 Using the MCP3204/3208 with Microcontroller (MCU) SPI Ports 00125 _sample.bytes[1] = _spiInstance->write(cmd1); // DS21298E-page 21 See FIGURE 6-1: SPI Communication using 8-bit segments (Mode 0,0: SCLK idles low) 00126 _sample.bytes[0] = _spiInstance->write(0); 00127 if (_cs != NULL) { 00128 _cs->write(1); 00129 } 00130 00131 } 00132 00133 } // End of namespace MCP320x_SPI
Generated on Wed Jul 13 2022 12:32:09 by
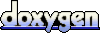