
This is used for sending Data to receiving mDot
Dependencies: libmDot-dev-mbed5-deprecated sd-driver ISL29011
Fork of mdot-examples by
itoa.cpp
00001 #include <string.h> 00002 #include "itoa.h" 00003 using namespace std; 00004 00005 // Implementation of itoa() 00006 char* itoa(int num, char* str, int base) 00007 { 00008 int i = 0; 00009 bool isNegative = false; 00010 00011 /* Handle 0 explicitely, otherwise empty string is printed for 0 */ 00012 if (num == 0) 00013 { 00014 str[i++] = '0'; 00015 str[i] = '\0'; 00016 return str; 00017 } 00018 00019 // In standard itoa(), negative numbers are handled only with 00020 // base 10. Otherwise numbers are considered unsigned. 00021 if (num < 0 && base == 10) 00022 { 00023 isNegative = true; 00024 num = -num; 00025 } 00026 00027 // Process individual digits 00028 while (num != 0) 00029 { 00030 int rem = num % base; 00031 str[i++] = (rem > 9)? (rem-10) + 'a' : rem + '0'; 00032 num = num/base; 00033 } 00034 00035 // If number is negative, append '-' 00036 if (isNegative) 00037 str[i++] = '-'; 00038 00039 str[i] = '\0'; // Append string terminator 00040 00041 // Reverse the string 00042 reverse(str); 00043 00044 return str; 00045 } 00046 00047 /* 00048 ** reverse string in place 00049 */ 00050 void reverse(char *s){ 00051 char *j; 00052 int c; 00053 00054 j = s + strlen(s) - 1; 00055 while(s < j) { 00056 c = *s; 00057 *s++ = *j; 00058 *j-- = c; 00059 } 00060 }
Generated on Tue Jul 12 2022 18:56:55 by
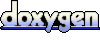