
The last version programs
Dependencies: mbed TrapezoidControl Pulse QEI
RingBuffer.h
00001 /* 00002 * RingBuffer.h 00003 * 00004 * Created: 2016/08/10 12:15:08 00005 * Author: masuk 00006 */ 00007 00008 00009 #ifndef RINGBUFFER_H_ 00010 #define RINGBUFFER_H_ 00011 00012 #include <stdint.h> 00013 00014 namespace RINGBUFFER 00015 { 00016 //循環型バッファ 使用するバッファの配列と大きさを指定してください 00017 class RingBuffer 00018 { 00019 struct 00020 { 00021 char *data; 00022 int size; 00023 uint8_t top; 00024 uint8_t bottom; 00025 uint8_t length; 00026 bool fullup; 00027 }Buffer; 00028 00029 public: 00030 RingBuffer(char *bufPtr, int size); 00031 00032 //バッファにデータを追加 00033 void PutData(char data, bool ASCIItoNum = false); 00034 void PutData(char *data, int length); 00035 void PutData(const char *str); 00036 00037 //バッファからデータを1byte読み出し 00038 char GetData(); 00039 00040 //バッファが飽和しているか確認 00041 bool IsFullup(); 00042 //バッファにデータが存在するか確認 00043 bool InAnyData(); 00044 }; 00045 } 00046 00047 00048 00049 #endif /* RINGBUFFER_H_ */
Generated on Fri Jul 15 2022 03:24:23 by
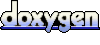