
The last version programs
Dependencies: mbed TrapezoidControl Pulse QEI
RS485.cpp
00001 #include "RS485.h" 00002 #include "mbed.h" 00003 #include "ActuatorHub/ActuatorHub.h" 00004 #include "LineHub/LineHub.h" 00005 #include "../../LED/LED.h" 00006 #include "../../System/Using.h" 00007 00008 namespace RS485 { 00009 00010 DigitalOut selectBitT(SELECTBIT_T_PIN); 00011 // DigitalOut selectBitR(SELECTBIT_R_PIN); 00012 Serial RS485Uart(RS485UART_TX, RS485UART_RX); 00013 Serial RS485Line(RS485LINE_TX, RS485LINE_RX); 00014 00015 bool readFase = 0; 00016 char buffer[RS485_BUFFER_LINE] = {0}; 00017 00018 void Transmit(); 00019 void Recieve(); 00020 00021 void RS485::Initialize() { 00022 selectBitT = 1; //送信固定 00023 // selectBitR = 0; //受信固定 00024 RS485Uart.baud(38400); 00025 RS485Uart.attach(Transmit, Serial::TxIrq); //送信割り込み 00026 RS485Line.baud(9600); 00027 RS485Line.attach(Recieve, Serial::RxIrq); //受信割り込み 00028 } 00029 00030 void Transmit() { 00031 // __disable_irq(); 00032 static uint8_t count = 0; 00033 RS485Uart.putc(RS485SendBuffer.GetData()); 00034 if(count >= 200) { 00035 #ifdef USE_MOTOR 00036 LED_DEBUG2 = !LED_DEBUG2; 00037 #endif 00038 00039 count = 0; 00040 } else count++; 00041 __enable_irq(); 00042 } 00043 00044 void Recieve() { 00045 __disable_irq(); 00046 static uint8_t time = 0; 00047 static uint8_t count = 0; 00048 char data = RS485Line.getc(); 00049 if (data == 'S') { 00050 readFase = true; 00051 time = 0; 00052 } else if(data == 'F') { 00053 readFase = false; 00054 for(int i = 0; i < 8; i++) { 00055 lineData[i] = buffer[i]; 00056 } 00057 time = 0; 00058 } else if(readFase) { 00059 buffer[time] = data; 00060 time++; 00061 } else { 00062 readFase = false; 00063 time = 0; 00064 } 00065 if(count >= 200) { 00066 LED_DEBUG1 = !LED_DEBUG1; 00067 count = 0; 00068 } else count++; 00069 __enable_irq(); 00070 } 00071 }
Generated on Fri Jul 15 2022 03:24:23 by
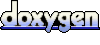