
The last version programs
Dependencies: mbed TrapezoidControl Pulse QEI
Process.h
00001 #ifndef PROCESS_H_ 00002 #define PROCESS_H_ 00003 00004 #include "mbed.h" 00005 00006 #define BUZZER_PIN PB_3 00007 #define EMG_0 LimitSw::IsPressed(0) 00008 #define EMG_1 LimitSw::IsPressed(1) 00009 00010 void SystemProcess(); 00011 00012 /* ---------- motor ---------- */ 00013 00014 #define TIRE_FL 0 00015 #define TIRE_BL 1 00016 #define TIRE_BR 2 00017 #define TIRE_FR 3 00018 #define LIFT_LB 4 00019 #define LIFT_RB 6 00020 #define LIFT_U 5 00021 00022 /* ---------- motor ---------- */ 00023 00024 /* ---------- limitswitch ---------- */ 00025 00026 #define LSW_LB 3 // 上下1段目 左 リミット 00027 #define LSW_RB 8 // 上下1段目 右 リミット 00028 #define LSW_UU 2 // 上下2段目 上 リミット 00029 #define LSW_UB 16 // 上下2段目 下 リミット 00030 #define TOW_1L 4 // タオル1 左 リミット 00031 #define TOW_1R 5 // タオル1 右 リミット 00032 #define TOW_2L 6 // タオル2 左 リミット 00033 #define TOW_2R 7 // タオル2 右 リミット 00034 #define LEFTlim 0 00035 #define RIGHTlim 1 00036 00037 #define SETTING_SW 10 // セッティングタイム用 00038 #define QF_SW 9 // 予選・決勝 切り替え 00039 #define UNFOLD_ZYOUGE_SW 11 // タオルセット用の展開 00040 //#define TOWEL1_SW 11 // タオル1 切り替え 00041 #define REDBLUE_SW 12 // 赤青 切り替え 00042 #define SHEETS_SW 13 // シーツのみモード 00043 #define UNFOLD_TOWL_SW 14 00044 #define START_SW 15 // スタートボタン 00045 00046 /* ---------- limitswitch ---------- */ 00047 00048 /* ---------- linetrace ---------- */ 00049 00050 #define LINE_FRONT 0 00051 #define LINE_BACK 2 00052 #define LINE_LEFT 3 00053 #define LINE_RIGHT 4 00054 #define LINE_TOW_1 5 00055 #define LINE_TOW_2 6 00056 00057 /* ---------- linetrace ---------- */ 00058 00059 /* ---------- solenoid ---------- */ 00060 00061 #define TOWEL0 3 00062 // タオル横 展開 00063 #define TOWEL1 0 // タオル1 00064 #define TOWEL2 1 // タオル2 00065 #define CLOTHESPIN 2 // シーツの洗濯ばさみ 00066 00067 /* ---------- solenoid ---------- */ 00068 00069 #define ECHO_0 PC_3 00070 #define ECHO_1 PC_1 00071 00072 #define TRIG_0 PC_0 00073 #define TRIG_1 PB_0 00074 00075 #define SELECT_1 PB_8//LS18 00076 #define SELECT_2 PC_9//LS19 00077 #define SELECT_3 PC_8//LS20 00078 00079 00080 typedef union 00081 { 00082 struct 00083 { 00084 unsigned int blue : 8; 00085 unsigned int green : 8; 00086 unsigned int red : 8; 00087 unsigned int : 8; 00088 }; 00089 uint32_t code; 00090 } TapeLedData; 00091 00092 enum TapeLED_Mode 00093 { 00094 EMS, 00095 Normal, 00096 Launch, 00097 }; 00098 00099 enum TapeLED_Color 00100 { 00101 Black = 0x000000, 00102 // Red = 0xff0000, 00103 Green = 0x008000, 00104 Blue = 0x0000ff, 00105 White = 0xffffff, 00106 // Orange = 0xffa500, 00107 // Yellow = 0xffff00, 00108 // Purple = 0x800080, 00109 // Cyan = 0x00ffff, 00110 // Magenta = 0xff00ff, 00111 // Lime = 0x00ff00 00112 00113 00114 Dimgray = 0x696969, 00115 Gray = 0x808080, 00116 DarkGray = 0xa9a9a9, 00117 Silver = 0xc0c0c0, 00118 LightGray = 0xd3d3d3, 00119 Gainsboro = 0xdcdcdc, 00120 Whitesmoke = 0xf5f5f5, 00121 Snow = 0xfffafa, 00122 Ghostwhite = 0xf8f8ff, 00123 Floralwhite = 0xfffaf0, 00124 Linen = 0xfaf0e6, 00125 Antiquewhite = 0xfaebd7, 00126 Papayawhip = 0xffefd5, 00127 Blanchedalmond = 0xffebcd, 00128 Bisque = 0xffe4c4, 00129 Moccasin = 0xffe4b5, 00130 Navajowhite = 0xffdead, 00131 Peachpuff = 0xffdab9, 00132 Mistyrose = 0xffe4e1, 00133 Lavenderblush = 0xfff0f5, 00134 Seashell = 0xfff5ee, 00135 Oldlace = 0xfdf5e6, 00136 Ivory = 0xfffff0, 00137 Honeydew = 0xf0fff0, 00138 Mintcream = 0xf5fffa, 00139 Azure = 0xf0ffff, 00140 Aliceblue = 0xf0f8ff, 00141 Lavender = 0xe6e6fa, 00142 Lightsteelblue = 0xb0c4de, 00143 Lightslategray = 0x778899, 00144 Slategray = 0x708090, 00145 Steelblue = 0x4682b4, 00146 Royalblue = 0x4169e1, 00147 Midnightblue = 0x191970, 00148 Navy = 0x000080, 00149 Darkblue = 0x00008b, 00150 Mediumblue = 0x0000cd, 00151 Dodgerblue = 0x1e90ff, 00152 Cornflowerblue = 0x6495ed, 00153 Deepskyblue = 0x00bfff, 00154 Lightskyblue = 0x87cefa, 00155 Skyblue = 0x87ceeb, 00156 Lightblue = 0xadd8e6, 00157 Powderblue = 0xb0e0e6, 00158 Paleturquoise = 0xafeeee, 00159 // Lightcyan = 0xe0ffff, 00160 Cyan = 0x00ffff, 00161 // Aqua = 0x00ffff, 00162 Turquoise = 0x40e0d0, 00163 Mediumturquoise = 0x48d1cc, 00164 Darkturquoise = 0x00ced1, 00165 Lightseagreen = 0x20b2aa, 00166 Cabetblue = 0x5f9ea0, 00167 Darkcyan = 0x008b8b, 00168 Teal = 0x008080, 00169 Darkslategray = 0x2f4f4f, 00170 Darkgreen = 0x006400, 00171 Forestgreen = 0x228b22, 00172 Seagreen = 0x2e8b57, 00173 Mediumseagreen = 0x3cb371, 00174 Mediumaquamarine = 0x66cdaa, 00175 Darkseagreen = 0x8fbc8f, 00176 Aquamarine = 0x7fffd4, 00177 Palegreen = 0x98fb98, 00178 Lightgreen = 0x90ee90, 00179 Springgreen = 0x00ff7f, 00180 Mediumspringgreen = 0x00fa9a, 00181 Lawngreen = 0x7cfc00, 00182 Chartreuse = 0x7fff00, 00183 Greenyellow = 0xadff2f, 00184 Lime = 0x00ff00, 00185 Limegreen = 0x32cd32, 00186 Yellowgreen = 0x9acd32, 00187 Darkolivegreen = 0x556b2f, 00188 Olivedrab = 0x6b8e23, 00189 Olive = 0x808000, 00190 Darkkhaki = 0xbdb76b, 00191 Palegoldenrod = 0xeee8aa, 00192 Cornsilk = 0xfff8dc, 00193 Beige = 0xf5f5dc, 00194 Lightyellow = 0xffffe0, 00195 Lightgoldenrodyellow = 0xfafad2, 00196 Lemonchiffon = 0xfffacd, 00197 Wheat = 0xf5deb3, 00198 Burlywood = 0xdeb887, 00199 Tan = 0xd2b48c, 00200 Khaki = 0xf0e68c, 00201 Yellow = 0xffff00, 00202 Gold = 0xffd700, 00203 // Orange = 0xffa500, 00204 Orange = 0xff2500, 00205 Sandybrown = 0xf4a460, 00206 Darkorange = 0xff8c00, 00207 Goldenrod = 0xdaa520, 00208 Peru = 0xcd853f, 00209 Darkgoldenrod = 0xb8860d, 00210 Chocolate = 0xd2691e, 00211 Sienna = 0xa0522d, 00212 Saddlebrown = 0x8b4513, 00213 Marron = 0x800000, 00214 Darkred = 0x8b0000, 00215 Brown = 0xa52a2a, 00216 Firebrick = 0xb22222, 00217 Indeanred = 0xcd5c5c, 00218 Rosybrown = 0xbc8f8f, 00219 Darksalmon = 0xe9967a, 00220 Lightcoral = 0xf08080, 00221 Salmon = 0xfa8072, 00222 Lightsalmon = 0xffa07a, 00223 Coral = 0xff7f50, 00224 Tomato = 0xff6347, 00225 Orangered = 0xff4500, 00226 Red = 0xff0000, 00227 Crimson = 0xdc143c, 00228 Mediumvioletred = 0xc71585, 00229 Deeppink = 0xff1493, 00230 Hotpink = 0xff69b4, 00231 Palevioletred = 0xdb7093, 00232 Pink = 0xffc0cb, 00233 Lightpink = 0xffb6c1, 00234 Thistle = 0xd8bfd8, 00235 Magenta = 0xff00ff, 00236 // Fuchsia = 0xff00ff, 00237 Violet = 0xee82ee, 00238 Plum = 0xdda0dd, 00239 Orchid = 0xda70d6, 00240 Mediumorchid = 0xba55d3, 00241 Darkorchid = 0x9932cc, 00242 Darkviolet = 0x9400d3, 00243 Darkmagenta = 0x8b008b, 00244 Purple = 0x800080, 00245 Indigo = 0x4b0082, 00246 Darkslateblue = 0x483d8b, 00247 Blueviolet = 0x8a2be2, 00248 Mediumpurple = 0x9370db, 00249 Slateblue = 0x6a5acd, 00250 Mediumslateblue = 0x7b68ee 00251 }; 00252 00253 extern TapeLedData sendLedData; 00254 00255 #endif
Generated on Fri Jul 15 2022 03:24:23 by
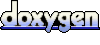