
test
Dependencies: mbed GR-PEACH_video mbed-rtos LCD_shield_config
main.cpp
00001 #include "mbed.h" 00002 #include "DisplayBace.h" 00003 #include "rtos.h" 00004 00005 #define VIDEO_CVBS (0) /* Analog Video Signal */ 00006 #define VIDEO_CMOS_CAMERA (1) /* Digital Video Signal */ 00007 #define VIDEO_YCBCR422 (0) 00008 #define VIDEO_RGB888 (1) 00009 #define VIDEO_RGB565 (2) 00010 00011 /**** User Selection *********/ 00012 /** Camera setting **/ 00013 #define VIDEO_INPUT_METHOD (VIDEO_CMOS_CAMERA) /* Select VIDEO_CVBS or VIDEO_CMOS_CAMERA */ 00014 #define VIDEO_INPUT_FORMAT (VIDEO_YCBCR422) /* Select VIDEO_YCBCR422 or VIDEO_RGB888 or VIDEO_RGB565 */ 00015 #define USE_VIDEO_CH (0) /* Select 0 or 1 If selecting VIDEO_CMOS_CAMERA, should be 0.) */ 00016 #define VIDEO_PAL (0) /* Select 0(NTSC) or 1(PAL) If selecting VIDEO_CVBS, this parameter is not referenced.) */ 00017 /** LCD setting **/ 00018 #define LCD_TYPE (0) /* Select 0(4.3inch) or 1(7.1inch) */ 00019 /*****************************/ 00020 00021 /** LCD shield config **/ 00022 #if (LCD_TYPE == 0) 00023 #include "LCD_shield_config_4_3inch.h" 00024 #else 00025 #include "LCD_shield_config_7_1inch.h" 00026 #endif 00027 00028 /** Video and Grapics (GRAPHICS_LAYER_0) parameter **/ 00029 /* video input */ 00030 #if USE_VIDEO_CH == (0) 00031 #define VIDEO_INPUT_CH (DisplayBase::VIDEO_INPUT_CHANNEL_0) 00032 #define VIDEO_INT_TYPE (DisplayBase::INT_TYPE_S0_VFIELD) 00033 #else 00034 #define VIDEO_INPUT_CH (DisplayBase::VIDEO_INPUT_CHANNEL_1) 00035 #define VIDEO_INT_TYPE (DisplayBase::INT_TYPE_S1_VFIELD) 00036 #endif 00037 00038 /* NTSC or PAL */ 00039 #if VIDEO_PAL == 0 00040 #define COL_SYS (DisplayBase::COL_SYS_NTSC_358) 00041 #else 00042 #define COL_SYS (DisplayBase::COL_SYS_PAL_443) 00043 #endif 00044 00045 /* Video input and LCD layer 0 output */ 00046 #if VIDEO_INPUT_FORMAT == VIDEO_YCBCR422 00047 #define VIDEO_FORMAT (DisplayBase::VIDEO_FORMAT_YCBCR422) 00048 #define GRAPHICS_FORMAT (DisplayBase::GRAPHICS_FORMAT_YCBCR422) 00049 #define WR_RD_WRSWA (DisplayBase::WR_RD_WRSWA_NON) 00050 #elif VIDEO_INPUT_FORMAT == VIDEO_RGB565 00051 #define VIDEO_FORMAT (DisplayBase::VIDEO_FORMAT_RGB565) 00052 #define GRAPHICS_FORMAT (DisplayBase::GRAPHICS_FORMAT_RGB565) 00053 #define WR_RD_WRSWA (DisplayBase::WR_RD_WRSWA_32_16BIT) 00054 #else 00055 #define VIDEO_FORMAT (DisplayBase::VIDEO_FORMAT_RGB888) 00056 #define GRAPHICS_FORMAT (DisplayBase::GRAPHICS_FORMAT_RGB888) 00057 #define WR_RD_WRSWA (DisplayBase::WR_RD_WRSWA_32BIT) 00058 #endif 00059 00060 /* The size of the video input is adjusted to the LCD size. */ 00061 #define VIDEO_PIXEL_HW LCD_PIXEL_WIDTH 00062 #define VIDEO_PIXEL_VW LCD_PIXEL_HEIGHT 00063 00064 /*! Frame buffer stride: Frame buffer stride should be set to a multiple of 32 or 128 00065 in accordance with the frame buffer burst transfer mode. */ 00066 /* FRAME BUFFER Parameter GRAPHICS_LAYER_0 */ 00067 #define FRAME_BUFFER_NUM (3u) 00068 #if ( VIDEO_INPUT_FORMAT == VIDEO_YCBCR422 || VIDEO_INPUT_FORMAT == VIDEO_RGB565 ) 00069 #define FRAME_BUFFER_BYTE_PER_PIXEL (2u) 00070 #else 00071 #define FRAME_BUFFER_BYTE_PER_PIXEL (4u) 00072 #endif 00073 #define FRAME_BUFFER_STRIDE (((LCD_PIXEL_WIDTH * FRAME_BUFFER_BYTE_PER_PIXEL) + 31u) & ~31u) 00074 00075 /* TOUCH BUFFER Parameter GRAPHICS_LAYER_1 */ 00076 #define TOUCH_BUFFER_BYTE_PER_PIXEL (2u) 00077 #define TOUCH_BUFFER_STRIDE (((LCD_PIXEL_WIDTH * TOUCH_BUFFER_BYTE_PER_PIXEL) + 31u) & ~31u) 00078 00079 /* Touch panel parameter */ 00080 #define TOUCH_NUM (2u) 00081 #define DROW_POINT (5) 00082 00083 static DisplayBase Display; 00084 static DigitalOut lcd_pwon(P7_15); 00085 static DigitalOut lcd_blon(P8_1); 00086 static PwmOut lcd_cntrst(P8_15); 00087 static Serial pc(USBTX, USBRX); 00088 static Semaphore sem_touch_int(0); 00089 static TouckKey_LCD_shield touch(P4_0, P2_13, I2C_SDA, I2C_SCL); 00090 static Thread * p_VideoLcdTask = NULL; 00091 static DigitalOut led_blue(LED_BLUE); 00092 00093 #if defined(__ICCARM__) 00094 /* 32 bytes aligned */ 00095 #pragma data_alignment=32 00096 static uint8_t user_frame_buffer0[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00097 static uint8_t user_frame_buffer1[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00098 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00099 static uint8_t user_frame_buffer_touch[TOUCH_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00100 #pragma data_alignment=4 00101 #else 00102 /* 32 bytes aligned */ 00103 static uint8_t user_frame_buffer0[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); 00104 static uint8_t user_frame_buffer1[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); 00105 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); 00106 static uint8_t user_frame_buffer_touch[TOUCH_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); 00107 #endif 00108 static uint8_t * FrameBufferTbl[FRAME_BUFFER_NUM] = {user_frame_buffer0, user_frame_buffer1, user_frame_buffer2}; 00109 static volatile int32_t vfield_count = 0; 00110 static int write_buff_num = 0; 00111 static int read_buff_num = 0; 00112 static bool graphics_init_end = false; 00113 00114 /****** cache control ******/ 00115 static void dcache_clean(void * p_buf, uint32_t size) { 00116 uint32_t start_addr = (uint32_t)p_buf & 0xFFFFFFE0; 00117 uint32_t end_addr = (uint32_t)p_buf + size; 00118 uint32_t addr; 00119 00120 /* Data cache clean */ 00121 for (addr = start_addr; addr < end_addr; addr += 0x20) { 00122 __v7_clean_dcache_mva((void *)addr); 00123 } 00124 } 00125 00126 /****** LCD ******/ 00127 #if(0) /* When needing LCD Vsync interrupt, please make it effective. */ 00128 static void IntCallbackFunc_LoVsync(DisplayBase::int_type_t int_type) { 00129 /* Interrupt callback function for Vsync interruption */ 00130 } 00131 #endif 00132 00133 static void Init_LCD_Display(void) { 00134 DisplayBase::graphics_error_t error; 00135 DisplayBase::lcd_config_t lcd_config; 00136 PinName lvds_pin[8] = { 00137 /* data pin */ 00138 P5_7, P5_6, P5_5, P5_4, P5_3, P5_2, P5_1, P5_0 00139 }; 00140 00141 lcd_pwon = 0; 00142 lcd_blon = 0; 00143 Thread::wait(100); 00144 lcd_pwon = 1; 00145 lcd_blon = 1; 00146 00147 Display.Graphics_Lvds_Port_Init(lvds_pin, 8); 00148 00149 /* Graphics initialization process */ 00150 lcd_config = LcdCfgTbl_LCD_shield; 00151 error = Display.Graphics_init(&lcd_config); 00152 if (error != DisplayBase::GRAPHICS_OK) { 00153 printf("Line %d, error %d\n", __LINE__, error); 00154 mbed_die(); 00155 } 00156 graphics_init_end = true; 00157 00158 #if(0) /* When needing LCD Vsync interrupt, please make it effective. */ 00159 /* Interrupt callback function setting (Vsync signal output from scaler 0) */ 00160 error = Display.Graphics_Irq_Handler_Set(DisplayBase::INT_TYPE_S0_LO_VSYNC, 0, IntCallbackFunc_LoVsync); 00161 if (error != DisplayBase::GRAPHICS_OK) { 00162 printf("Line %d, error %d\n", __LINE__, error); 00163 mbed_die(); 00164 } 00165 #endif 00166 } 00167 00168 static void Start_LCD_Display(uint8_t * p_buf) { 00169 DisplayBase::rect_t rect; 00170 00171 rect.vs = 0; 00172 rect.vw = LCD_PIXEL_HEIGHT; 00173 rect.hs = 0; 00174 rect.hw = LCD_PIXEL_WIDTH; 00175 Display.Graphics_Read_Setting( 00176 DisplayBase::GRAPHICS_LAYER_0, 00177 (void *)p_buf, 00178 FRAME_BUFFER_STRIDE, 00179 GRAPHICS_FORMAT, 00180 WR_RD_WRSWA, 00181 &rect 00182 ); 00183 Display.Graphics_Start(DisplayBase::GRAPHICS_LAYER_0); 00184 } 00185 00186 /****** Video ******/ 00187 #if(0) /* When needing video Vsync interrupt, please make it effective. */ 00188 static void IntCallbackFunc_ViVsync(DisplayBase::int_type_t int_type) { 00189 /* Interrupt callback function for Vsync interruption */ 00190 } 00191 #endif 00192 00193 static void IntCallbackFunc_Vfield(DisplayBase::int_type_t int_type) { 00194 /* Interrupt callback function */ 00195 if (vfield_count == 0) { 00196 vfield_count = 1; 00197 } else { 00198 vfield_count = 0; 00199 if (p_VideoLcdTask != NULL) { 00200 p_VideoLcdTask->signal_set(1); 00201 } 00202 } 00203 } 00204 00205 static void Init_Video(void) { 00206 DisplayBase::graphics_error_t error; 00207 00208 /* Graphics initialization process */ 00209 if (graphics_init_end == false) { 00210 /* When not initializing LCD, this processing is needed. */ 00211 error = Display.Graphics_init(NULL); 00212 if (error != DisplayBase::GRAPHICS_OK) { 00213 printf("Line %d, error %d\n", __LINE__, error); 00214 mbed_die(); 00215 } 00216 graphics_init_end = true; 00217 } 00218 00219 #if VIDEO_INPUT_METHOD == VIDEO_CVBS 00220 error = Display.Graphics_Video_init( DisplayBase::INPUT_SEL_VDEC, NULL); 00221 if( error != DisplayBase::GRAPHICS_OK ) { 00222 printf("Line %d, error %d\n", __LINE__, error); 00223 mbed_die(); 00224 } 00225 #elif VIDEO_INPUT_METHOD == VIDEO_CMOS_CAMERA 00226 DisplayBase::video_ext_in_config_t ext_in_config; 00227 PinName cmos_camera_pin[11] = { 00228 /* data pin */ 00229 P2_7, P2_6, P2_5, P2_4, P2_3, P2_2, P2_1, P2_0, 00230 /* control pin */ 00231 P10_0, /* DV0_CLK */ 00232 P1_0, /* DV0_Vsync */ 00233 P1_1 /* DV0_Hsync */ 00234 }; 00235 00236 /* MT9V111 camera input config */ 00237 ext_in_config.inp_format = DisplayBase::VIDEO_EXTIN_FORMAT_BT601; /* BT601 8bit YCbCr format */ 00238 ext_in_config.inp_pxd_edge = DisplayBase::EDGE_RISING; /* Clock edge select for capturing data */ 00239 ext_in_config.inp_vs_edge = DisplayBase::EDGE_RISING; /* Clock edge select for capturing Vsync signals */ 00240 ext_in_config.inp_hs_edge = DisplayBase::EDGE_RISING; /* Clock edge select for capturing Hsync signals */ 00241 ext_in_config.inp_endian_on = DisplayBase::OFF; /* External input bit endian change on/off */ 00242 ext_in_config.inp_swap_on = DisplayBase::OFF; /* External input B/R signal swap on/off */ 00243 ext_in_config.inp_vs_inv = DisplayBase::SIG_POL_NOT_INVERTED; /* External input DV_VSYNC inversion control */ 00244 ext_in_config.inp_hs_inv = DisplayBase::SIG_POL_INVERTED; /* External input DV_HSYNC inversion control */ 00245 ext_in_config.inp_f525_625 = DisplayBase::EXTIN_LINE_525; /* Number of lines for BT.656 external input */ 00246 ext_in_config.inp_h_pos = DisplayBase::EXTIN_H_POS_CRYCBY; /* Y/Cb/Y/Cr data string start timing to Hsync reference */ 00247 ext_in_config.cap_vs_pos = 6; /* Capture start position from Vsync */ 00248 ext_in_config.cap_hs_pos = 150; /* Capture start position form Hsync */ 00249 #if (LCD_TYPE == 0) 00250 /* The same screen ratio as the screen ratio of the LCD. */ 00251 ext_in_config.cap_width = 640; /* Capture width */ 00252 ext_in_config.cap_height = 363; /* Capture height Max 468[line] 00253 Due to CMOS(MT9V111) output signal timing and VDC5 specification */ 00254 #else 00255 ext_in_config.cap_width = 640; /* Capture width */ 00256 ext_in_config.cap_height = 468; /* Capture height Max 468[line] 00257 Due to CMOS(MT9V111) output signal timing and VDC5 specification */ 00258 #endif 00259 error = Display.Graphics_Video_init( DisplayBase::INPUT_SEL_EXT, &ext_in_config); 00260 if( error != DisplayBase::GRAPHICS_OK ) { 00261 printf("Line %d, error %d\n", __LINE__, error); 00262 mbed_die(); 00263 } 00264 00265 /* Camera input port setting */ 00266 error = Display.Graphics_Dvinput_Port_Init(cmos_camera_pin, 11); 00267 if( error != DisplayBase::GRAPHICS_OK ) { 00268 printf("Line %d, error %d\n", __LINE__, error); 00269 mbed_die(); 00270 } 00271 #endif 00272 00273 #if(0) /* When needing video Vsync interrupt, please make it effective. */ 00274 /* Interrupt callback function setting (Vsync signal input to scaler 0) */ 00275 error = Display.Graphics_Irq_Handler_Set(DisplayBase::INT_TYPE_S0_VI_VSYNC, 0, IntCallbackFunc_ViVsync); 00276 if (error != DisplayBase::GRAPHICS_OK) { 00277 printf("Line %d, error %d\n", __LINE__, error); 00278 mbed_die(); 00279 } 00280 #endif 00281 00282 /* Interrupt callback function setting (Field end signal for recording function in scaler 0) */ 00283 error = Display.Graphics_Irq_Handler_Set(VIDEO_INT_TYPE, 0, IntCallbackFunc_Vfield); 00284 if (error != DisplayBase::GRAPHICS_OK) { 00285 printf("Line %d, error %d\n", __LINE__, error); 00286 mbed_die(); 00287 } 00288 } 00289 00290 static void Start_Video(uint8_t * p_buf) { 00291 DisplayBase::graphics_error_t error; 00292 00293 /* Video capture setting (progressive form fixed) */ 00294 error = Display.Video_Write_Setting( 00295 VIDEO_INPUT_CH, 00296 COL_SYS, 00297 p_buf, 00298 FRAME_BUFFER_STRIDE, 00299 VIDEO_FORMAT, 00300 WR_RD_WRSWA, 00301 VIDEO_PIXEL_VW, 00302 VIDEO_PIXEL_HW 00303 ); 00304 if (error != DisplayBase::GRAPHICS_OK) { 00305 printf("Line %d, error %d\n", __LINE__, error); 00306 mbed_die(); 00307 } 00308 00309 /* Video write process start */ 00310 error = Display.Video_Start(VIDEO_INPUT_CH); 00311 if (error != DisplayBase::GRAPHICS_OK) { 00312 printf("Line %d, error %d\n", __LINE__, error); 00313 mbed_die(); 00314 } 00315 00316 /* Video write process stop */ 00317 error = Display.Video_Stop(VIDEO_INPUT_CH); 00318 if (error != DisplayBase::GRAPHICS_OK) { 00319 printf("Line %d, error %d\n", __LINE__, error); 00320 mbed_die(); 00321 } 00322 00323 /* Video write process start */ 00324 error = Display.Video_Start(VIDEO_INPUT_CH); 00325 if (error != DisplayBase::GRAPHICS_OK) { 00326 printf("Line %d, error %d\n", __LINE__, error); 00327 mbed_die(); 00328 } 00329 } 00330 00331 /****** Video input is output to LCD ******/ 00332 static void video_lcd_task(void const *) { 00333 DisplayBase::graphics_error_t error; 00334 int wk_num; 00335 int i; 00336 00337 /* Initialization memory */ 00338 for (i = 0; i < FRAME_BUFFER_NUM; i++) { 00339 memset(FrameBufferTbl[i], 0, (FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT)); 00340 dcache_clean(FrameBufferTbl[i], (FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT)); 00341 } 00342 00343 /* Start of Video */ 00344 Start_Video(FrameBufferTbl[write_buff_num]); 00345 00346 /* Wait for first video drawing */ 00347 Thread::signal_wait(1); 00348 write_buff_num++; 00349 if (write_buff_num >= FRAME_BUFFER_NUM) { 00350 write_buff_num = 0; 00351 } 00352 error = Display.Video_Write_Change(VIDEO_INPUT_CH, FrameBufferTbl[write_buff_num], FRAME_BUFFER_STRIDE); 00353 if (error != DisplayBase::GRAPHICS_OK) { 00354 printf("Line %d, error %d\n", __LINE__, error); 00355 mbed_die(); 00356 } 00357 00358 /* Start of LCD */ 00359 Start_LCD_Display(FrameBufferTbl[read_buff_num]); 00360 00361 /* Backlight on */ 00362 Thread::wait(200); 00363 lcd_cntrst.write(1.0); 00364 00365 while (1) { 00366 Thread::signal_wait(1); 00367 wk_num = write_buff_num + 1; 00368 if (wk_num >= FRAME_BUFFER_NUM) { 00369 wk_num = 0; 00370 } 00371 /* If the next buffer is empty, it's changed. */ 00372 if (wk_num != read_buff_num) { 00373 read_buff_num = write_buff_num; 00374 write_buff_num = wk_num; 00375 /* Change video buffer */ 00376 error = Display.Video_Write_Change(VIDEO_INPUT_CH, FrameBufferTbl[write_buff_num], FRAME_BUFFER_STRIDE); 00377 if (error != DisplayBase::GRAPHICS_OK) { 00378 printf("Line %d, error %d\n", __LINE__, error); 00379 mbed_die(); 00380 } 00381 /* Change LCD buffer */ 00382 Display.Graphics_Read_Change(DisplayBase::GRAPHICS_LAYER_0, (void *)FrameBufferTbl[read_buff_num]); 00383 } 00384 } 00385 } 00386 00387 /****** Touch panel ******/ 00388 static void drow_touch_pos(uint8_t * p_buf, int id, int x, int y) { 00389 int idx_base; 00390 int wk_idx; 00391 int i; 00392 int j; 00393 uint8_t coller_pix[TOUCH_BUFFER_BYTE_PER_PIXEL]; /* ARGB4444 */ 00394 00395 /* A coordinate in the upper left is calculated from a central coordinate. */ 00396 if ((x - (DROW_POINT / 2)) >= 0) { 00397 x -= (DROW_POINT / 2); 00398 } 00399 if (x > (LCD_PIXEL_WIDTH - DROW_POINT)) { 00400 x = (LCD_PIXEL_WIDTH - DROW_POINT); 00401 } 00402 if ((y - (DROW_POINT / 2)) >= 0) { 00403 y -= (DROW_POINT / 2); 00404 } 00405 if (y > (LCD_PIXEL_HEIGHT - DROW_POINT)) { 00406 y = (LCD_PIXEL_HEIGHT - DROW_POINT); 00407 } 00408 idx_base = (x + (LCD_PIXEL_WIDTH * y)) * TOUCH_BUFFER_BYTE_PER_PIXEL; 00409 00410 /* Select color */ 00411 if (id == 0) { 00412 /* Blue */ 00413 coller_pix[0] = 0x0F; /* 4:Green 4:Blue */ 00414 coller_pix[1] = 0xF0; /* 4:Alpha 4:Red */ 00415 } else { 00416 /* Pink */ 00417 coller_pix[0] = 0x07; /* 4:Green 4:Blue */ 00418 coller_pix[1] = 0xFF; /* 4:Alpha 4:Red */ 00419 } 00420 00421 /* Drawing */ 00422 for (i = 0; i < DROW_POINT; i++) { 00423 wk_idx = idx_base + (LCD_PIXEL_WIDTH * TOUCH_BUFFER_BYTE_PER_PIXEL * i); 00424 for (j = 0; j < DROW_POINT; j++) { 00425 p_buf[wk_idx++] = coller_pix[0]; 00426 p_buf[wk_idx++] = coller_pix[1]; 00427 } 00428 } 00429 } 00430 00431 static void drow_touch_keyonoff(uint8_t * p_buf, int id, bool onoff) { 00432 int idx_base; 00433 int wk_idx; 00434 int i; 00435 int j; 00436 uint8_t coller_pix[TOUCH_BUFFER_BYTE_PER_PIXEL]; /* ARGB4444 */ 00437 00438 /* Display position */ 00439 if (id == 0) { 00440 idx_base = 0; 00441 } else { 00442 idx_base = DROW_POINT * TOUCH_BUFFER_BYTE_PER_PIXEL; 00443 } 00444 00445 /* Select color */ 00446 if (onoff == false) { 00447 /* Transparency */ 00448 coller_pix[0] = 0x00; /* 4:Green 4:Blue */ 00449 coller_pix[1] = 0x00; /* 4:Alpha 4:Red */ 00450 } else { 00451 /* White */ 00452 coller_pix[0] = 0xff; /* 4:Green 4:Blue */ 00453 coller_pix[1] = 0xff; /* 4:Alpha 4:Red */ 00454 } 00455 00456 /* Drawing */ 00457 for (i = 0; i < DROW_POINT; i++) { 00458 wk_idx = idx_base + (LCD_PIXEL_WIDTH * TOUCH_BUFFER_BYTE_PER_PIXEL * i); 00459 for (j = 0; j < DROW_POINT; j++) { 00460 p_buf[wk_idx++] = coller_pix[0]; 00461 p_buf[wk_idx++] = coller_pix[1]; 00462 } 00463 } 00464 } 00465 00466 static void touch_int_callback(void) { 00467 sem_touch_int.release(); 00468 } 00469 00470 static void touch_task(void const *) { 00471 DisplayBase::rect_t rect; 00472 TouchKey::touch_pos_t touch_pos[TOUCH_NUM]; 00473 int touch_num = 0; 00474 int touch_num_last = 0; 00475 int i; 00476 00477 /* The layer by which the touch panel location is drawn */ 00478 memset(user_frame_buffer_touch, 0, sizeof(user_frame_buffer_touch)); 00479 dcache_clean(user_frame_buffer_touch, sizeof(user_frame_buffer_touch)); 00480 rect.vs = 0; 00481 rect.vw = LCD_PIXEL_HEIGHT; 00482 rect.hs = 0; 00483 rect.hw = LCD_PIXEL_WIDTH; 00484 Display.Graphics_Read_Setting( 00485 DisplayBase::GRAPHICS_LAYER_1, 00486 (void *)user_frame_buffer_touch, 00487 TOUCH_BUFFER_STRIDE, 00488 DisplayBase::GRAPHICS_FORMAT_ARGB4444, 00489 DisplayBase::WR_RD_WRSWA_32_16BIT, 00490 &rect 00491 ); 00492 Display.Graphics_Start(DisplayBase::GRAPHICS_LAYER_1); 00493 00494 /* Callback setting */ 00495 touch.SetCallback(&touch_int_callback); 00496 00497 /* Reset touch IC */ 00498 touch.Reset(); 00499 00500 while (1) { 00501 /* Wait touch event */ 00502 sem_touch_int.wait(); 00503 00504 /* Get touch coordinates */ 00505 touch_num = touch.GetCoordinates(TOUCH_NUM, touch_pos); 00506 00507 /* When it's a new touch, touch frame buffer is initialized */ 00508 if ((touch_num != 0) && (touch_num_last == 0)) { 00509 memset(user_frame_buffer_touch, 0, sizeof(user_frame_buffer_touch)); 00510 } 00511 touch_num_last = touch_num; 00512 00513 /* Drawing of a touch coordinate */ 00514 for (i = 0; i < TOUCH_NUM; i ++) { 00515 printf("{valid=%d,x=%d,y=%d} ", touch_pos[i].valid, touch_pos[i].x, touch_pos[i].y); 00516 drow_touch_keyonoff(user_frame_buffer_touch, i, touch_pos[i].valid); 00517 if (touch_pos[i].valid) { 00518 drow_touch_pos(user_frame_buffer_touch, i, touch_pos[i].x, touch_pos[i].y); 00519 } 00520 } 00521 printf("\n"); 00522 00523 /* Data cache clean */ 00524 dcache_clean(user_frame_buffer_touch, sizeof(user_frame_buffer_touch)); 00525 } 00526 } 00527 00528 /****** main ******/ 00529 int main(void) { 00530 /* Change the baud rate of the printf() */ 00531 pc.baud(921600); 00532 00533 /* Initialization of LCD */ 00534 Init_LCD_Display(); /* When using LCD, please call before than Init_Video(). */ 00535 00536 /* Initialization of Video */ 00537 Init_Video(); 00538 00539 /* Start Video and Lcd processing */ 00540 p_VideoLcdTask = new Thread(video_lcd_task, NULL, osPriorityAboveNormal, 1024 * 4); 00541 00542 /* Start touch panel processing */ 00543 Thread touchTask(touch_task, NULL, osPriorityNormal, 1024 * 4); 00544 00545 while (1) { 00546 led_blue = !led_blue; 00547 Thread::wait(1000); 00548 } 00549 }
Generated on Fri Jul 15 2022 16:47:24 by
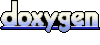