
Mar. 14. 2018
Dependencies: GraphicsFramework GR-PEACH_video LCD_shield_config AsciiFont R_BSP USBHost_custom
TrInterface.h
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #if !defined( _INTERFACE_H_ ) 00018 #define _INTERFACE_H_ 00019 #include "STBTrTypedef.h" 00020 #include "STBCommonDef.h" 00021 #include "STBCommonType.h" 00022 #include "STBTrValidValue.h" 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 00029 ////////////////////////////////////////////////////////////////////////////////// 00030 ////////////////////////////////////////////////////////////////////////////////// 00031 /////////// Define ////////////// 00032 ////////////////////////////////////////////////////////////////////////////////// 00033 ////////////////////////////////////////////////////////////////////////////////// 00034 00035 /* refer to past "STB_BACK_MAX-1" frames of results */ 00036 #define STB_TR_BACK_MAX 2 00037 00038 #define STB_TR_DET_CNT_MAX 35 00039 #define STB_TR_TRA_CNT_MAX 35 00040 00041 //If the face isn't find out during tracking, set until how many frames can look for it. 00042 //In the case of tracking failed with a specified number of frames consecutively, end of tracking as the face lost. 00043 #define STB_TR_INI_RETRY 2 00044 #define STB_TR_MIN_RETRY 0 00045 #define STB_TR_MAX_RETRY 300 00046 00047 //Specifies settings % 00048 //For example, about the percentage of detected position change, setting the value to 30(<- initialize value) 00049 //in the case of position change under 30 percentage from the previous frame, output detected position of the previous frame 00050 //When it exceeds 30%, the detection position coordinate is output as it is. 00051 #define STB_TR_INI_STEADINESS_POS 30 00052 #define STB_TR_MIN_STEADINESS_POS 0 00053 #define STB_TR_MAX_STEADINESS_POS 100 00054 00055 //Specifies settings % 00056 //In the case of the percentage of detection size change setting to 30(<- initialize value) 00057 //in the case of size change under 30 percentage from the previous frame, output detected size of the previous frame 00058 //When it exceeds 30%, the detection size is output as it is. 00059 #define STB_TR_INI_STEADINESS_SIZE 30 00060 #define STB_TR_MIN_STEADINESS_SIZE 0 00061 #define STB_TR_MAX_STEADINESS_SIZE 100 00062 00063 00064 ////////////////////////////////////////////////////////////////////////////////// 00065 ////////////////////////////////////////////////////////////////////////////////// 00066 /////////// Struct ////////////// 00067 ////////////////////////////////////////////////////////////////////////////////// 00068 ////////////////////////////////////////////////////////////////////////////////// 00069 00070 00071 typedef struct{ 00072 STB_INT32 cnt ; 00073 STB_INT32 *nDetID ; /*previous detected result ID*/ 00074 STB_INT32 *nTraID ; /*Tracking ID*/ 00075 STB_INT32 *posX ; /* Center x-coordinate */ 00076 STB_INT32 *posY ; /* Center y-coordinate */ 00077 STB_INT32 *size ; /* Size */ 00078 STB_INT32 *conf ; /* Degree of confidence */ 00079 STB_INT32 *retryN ; /*Continuous retry count*/ 00080 }ROI_SYS; 00081 00082 00083 /*---------------------------------------------------------------------------*/ 00084 typedef struct tagPEHANDLE { 00085 STB_INT8 *trPtr ; 00086 STB_INT32 detCntMax ;//Maximum of detected people 00087 STB_INT32 traCntMax ;//Maximum number of tracking people 00088 STB_INT32 retryCnt ;//Retry count 00089 STB_INT32 stedPos ;//stabilization parameter(position) 00090 STB_INT32 stedSize ;//stabilization parameter(size) 00091 STB_INT32 fcCntAcc ;//Number of faces (cumulative) 00092 STB_INT32 bdCntAcc ;//a number of human bodies(cumulative) 00093 STB_TR_DET *stbTrDet ;//Present data before the stabilization(input). 00094 ROI_SYS *fcRec ;//past data 00095 ROI_SYS *bdRec ;//past data 00096 STB_TR_RES_FACES *resFaces ;//present data after the stabilization(output) 00097 STB_TR_RES_BODYS *resBodys ;//present data after the stabilization(output) 00098 STB_INT32 *wIdPreCur ; 00099 STB_INT32 *wIdCurPre ; 00100 STB_INT32 *wDstTbl ; 00101 STBExecFlg *execFlg ; 00102 ROI_SYS *wRoi ; 00103 } *TRHANDLE; 00104 00105 ////////////////////////////////////////////////////////////////////////////////// 00106 ////////////////////////////////////////////////////////////////////////////////// 00107 /////////// Func ////////////// 00108 ////////////////////////////////////////////////////////////////////////////////// 00109 ////////////////////////////////////////////////////////////////////////////////// 00110 00111 TRHANDLE TrCreateHandle ( const STBExecFlg* execFlg ,const STB_INT32 nDetCntMax, const STB_INT32 nTraCntMax ); 00112 STB_INT32 TrDeleteHandle ( TRHANDLE handle); 00113 STB_INT32 TrSetDetect ( TRHANDLE handle , const STB_TR_DET *stbTrDet); 00114 STB_INT32 TrExecute ( TRHANDLE handle); 00115 STB_INT32 TrClear ( TRHANDLE handle); 00116 STB_INT32 TrGetResult ( TRHANDLE handle , STB_TR_RES_FACES* fcResult,STB_TR_RES_BODYS* bdResult); 00117 STB_INT32 TrSetRetryCount ( TRHANDLE handle , STB_INT32 nRetryCount ); 00118 STB_INT32 TrGetRetryCount ( TRHANDLE handle , STB_INT32* nRetryCount ); 00119 STB_INT32 TrSetStedinessParam ( TRHANDLE handle , STB_INT32 nStedinessPos , STB_INT32 nStedinessSize ); 00120 STB_INT32 TrGetStedinessParam ( TRHANDLE handle , STB_INT32* nStedinessPos , STB_INT32* nStedinessSize ); 00121 00122 00123 00124 #ifdef __cplusplus 00125 } 00126 #endif 00127 00128 #endif
Generated on Tue Jul 12 2022 16:00:45 by
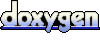