
Mar. 14. 2018
Dependencies: GraphicsFramework GR-PEACH_video LCD_shield_config AsciiFont R_BSP USBHost_custom
STBTypedefOutput.h
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 00018 #ifndef STBTYPEDEF_H__ 00019 #define STBTYPEDEF_H__ 00020 00021 #ifndef VOID 00022 #define VOID void 00023 #endif 00024 00025 typedef signed char STB_INT8 ; /*8-bit signed integer*/ 00026 typedef unsigned char STB_UINT8 ; /*8-bit unsigned integer*/ 00027 typedef signed short STB_INT16 ; /*16-bit signed integer*/ 00028 typedef unsigned short STB_UINT16 ; /*16-bit unsigned integer*/ 00029 typedef int STB_INT32 ; /*32 bit signed integer*/ 00030 typedef unsigned int STB_UINT32 ; /*32 bit unsigned integer*/ 00031 typedef float STB_FLOAT32 ; /*32-bit floating point number*/ 00032 typedef double STB_FLOAT64 ; /*64-bit floating point number*/ 00033 00034 00035 typedef enum { 00036 STB_STATUS_NO_DATA = -1, /*No data : No data for the relevant person*/ 00037 STB_STATUS_CALCULATING = 0, /* during stabilization : a number of data for relevant people aren't enough(a number of frames that relevant people are taken) */ 00038 STB_STATUS_COMPLETE = 1, /*stabilization done : the frames which done stabilization*/ 00039 STB_STATUS_FIXED = 2, /*stabilization fixed : already stabilization done, the results is fixed*/ 00040 } STB_STATUS;/*Status of stabilization*/ 00041 00042 #define STB_CONF_NO_DATA -1 /*No confidence(No data or in the case of stabilization time)*/ 00043 00044 /* Expression */ 00045 typedef enum { 00046 STB_EX_UNKNOWN = -1, 00047 STB_EX_NEUTRAL = 0, 00048 STB_EX_HAPPINESS, 00049 STB_EX_SURPRISE, 00050 STB_EX_ANGER, 00051 STB_EX_SADNESS, 00052 STB_EX_MAX 00053 }STB_EXPRESSION; 00054 00055 /*General purpose stabilization result structure*/ 00056 typedef struct { 00057 STB_STATUS status;/* Stabilization status */ 00058 STB_INT32 conf; /* Stabilization confidence */ 00059 STB_INT32 value; 00060 } STB_RES; 00061 00062 /*result of Gaze estimation*/ 00063 typedef struct { 00064 STB_STATUS status;/* Stabilization status */ 00065 STB_INT32 conf; /* Stabilization confidence */ 00066 STB_INT32 UD; 00067 STB_INT32 LR; 00068 } STB_GAZE; 00069 00070 /*Face direction result*/ 00071 typedef struct { 00072 STB_STATUS status;/* Stabilization status */ 00073 STB_INT32 conf; /* Stabilization confidence */ 00074 STB_INT32 yaw; 00075 STB_INT32 pitch; 00076 STB_INT32 roll; 00077 } STB_DIR; 00078 00079 /*result of Blink estimation*/ 00080 typedef struct { 00081 STB_STATUS status;/* Stabilization status */ 00082 STB_INT32 ratioL; 00083 STB_INT32 ratioR; 00084 } STB_BLINK; 00085 00086 /*Detection position structure*/ 00087 typedef struct { 00088 STB_UINT32 x; 00089 STB_UINT32 y; 00090 } STB_POS; 00091 00092 /*Face stabilization result structure*/ 00093 typedef struct { 00094 STB_INT32 nDetectID; 00095 STB_INT32 nTrackingID; 00096 STB_POS center; 00097 STB_UINT32 nSize; 00098 STB_INT32 conf; 00099 STB_DIR direction; 00100 STB_RES age; 00101 STB_RES gender; 00102 STB_GAZE gaze; 00103 STB_BLINK blink; 00104 STB_RES expression; 00105 STB_RES recognition; 00106 } STB_FACE; 00107 00108 /*Human body result structure*/ 00109 typedef struct { 00110 STB_INT32 nDetectID; 00111 STB_INT32 nTrackingID; 00112 STB_POS center; 00113 STB_UINT32 nSize; 00114 STB_INT32 conf; 00115 } STB_BODY; 00116 00117 00118 00119 #endif /* STBTYPEDEF_H__ */
Generated on Tue Jul 12 2022 16:00:45 by
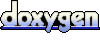