
Mar. 14. 2018
Dependencies: GraphicsFramework GR-PEACH_video LCD_shield_config AsciiFont R_BSP USBHost_custom
PeInterface.c
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #include "PeInterface.h" 00018 #include "STBPeAPI.h" 00019 00020 /*Value range check*/ 00021 #define ISVALID_RANGE( val , min , max ) ( ( (min) <= (val) ) && ( (val) <= (max) ) ) 00022 00023 /*--------------------------------------------------------------------- 00024 ---------------------------------------------------------------------*/ 00025 /*error check*/ 00026 /*--------------------------------------------------------------------- 00027 ---------------------------------------------------------------------*/ 00028 static STB_INT32 PeIsValidValue( 00029 const STB_INT32 nValue , 00030 const STB_INT32 nLimitMin , 00031 const STB_INT32 nLimitMax ) 00032 { 00033 STB_INT32 nRet; 00034 for( nRet = STB_ERR_INVALIDPARAM; nRet != STB_NORMAL; nRet = STB_NORMAL ){ 00035 if( ! ISVALID_RANGE( nValue , nLimitMin , nLimitMax ) ){ break; } 00036 } 00037 return nRet; 00038 } 00039 /*--------------------------------------------------------------------- 00040 ---------------------------------------------------------------------*/ 00041 static STB_INT32 PeIsValidPointer( const VOID* pPointer ) 00042 { 00043 STB_INT32 nRet; 00044 for( nRet = STB_ERR_INVALIDPARAM; nRet != STB_NORMAL; nRet = STB_NORMAL ){ 00045 if( NULL == pPointer ){ break; } 00046 } 00047 return nRet; 00048 } 00049 00050 /*------------------------------------------------------------------------------------------------------------------*/ 00051 /* PeCalcPeSize */ 00052 /*------------------------------------------------------------------------------------------------------------------*/ 00053 STB_UINT32 PeCalcPeSize ( STB_UINT32 nTraCntMax ) 00054 { 00055 STB_UINT32 retVal ; 00056 00057 retVal = 0 ; 00058 00059 retVal += 100 ;///Margin : alignment 00060 00061 00062 00063 retVal += sizeof( FACE_DET ) * nTraCntMax ; // peDet.fcDet 00064 retVal += sizeof( STB_PE_DET ) * STB_PE_BACK_MAX ; // peDetRec 00065 retVal += sizeof( FACE_DET ) * nTraCntMax * STB_PE_BACK_MAX; // handle->peDetRec[t].fcDet 00066 retVal += sizeof( STB_PE_FACE ) * nTraCntMax ; // peRes.peFace 00067 retVal += sizeof( STBExecFlg ) ; // execFlg 00068 00069 return retVal; 00070 } 00071 /*------------------------------------------------------------------------------------------------------------------*/ 00072 /* PeSharePeSize */ 00073 /*------------------------------------------------------------------------------------------------------------------*/ 00074 void PeSharePeSize ( PEHANDLE handle ) 00075 { 00076 00077 STB_UINT32 t; 00078 STB_INT8 *stbPtr = handle->pePtr ; 00079 STB_UINT32 nTraCntMax = handle->peCntMax; 00080 00081 handle->peDet.fcDet = ( FACE_DET* ) stbPtr; stbPtr += ( sizeof( FACE_DET ) * nTraCntMax ); 00082 handle->peDetRec = ( STB_PE_DET* ) stbPtr; stbPtr += ( sizeof( STB_PE_DET ) * STB_PE_BACK_MAX); 00083 for( t = 0 ; t < STB_PE_BACK_MAX ; t++ ) 00084 { 00085 handle->peDetRec[t].fcDet = ( FACE_DET* ) stbPtr; stbPtr += ( sizeof( FACE_DET ) * nTraCntMax ); 00086 } 00087 handle->peRes.peFace = ( STB_PE_FACE* ) stbPtr; stbPtr += ( sizeof( STB_PE_FACE) * nTraCntMax ); 00088 handle->execFlg = ( STBExecFlg* ) stbPtr; stbPtr += ( sizeof( STBExecFlg ) ); 00089 00090 } 00091 /*--------------------------------------------------------------------- 00092 ---------------------------------------------------------------------*/ 00093 /*Create handle*/ 00094 PEHANDLE PeCreateHandle( const STBExecFlg* execFlg ,const STB_INT32 nTraCntMax ){ 00095 00096 PEHANDLE handle; 00097 STB_INT32 t , i ,j; 00098 STB_INT32 tmpVal ; 00099 STB_INT32 nRet ; 00100 00101 nRet = PeIsValidPointer(execFlg); 00102 if(nRet != STB_NORMAL) 00103 { 00104 return NULL; 00105 } 00106 00107 if( nTraCntMax < 1 || STB_PE_TRA_CNT_MAX < nTraCntMax ) 00108 { 00109 return NULL; 00110 } 00111 00112 00113 00114 /*do handle's Malloc here*/ 00115 handle = ( PEHANDLE )malloc( sizeof(*handle) ); 00116 if(handle == NULL) 00117 { 00118 return NULL; 00119 } 00120 00121 /*initial value---------------------------------------------------------------------*/ 00122 handle->peFaceDirUDMin = STB_PE_DIR_MIN_UD_INI;//The face on top/down allowable range min. 00123 handle->peFaceDirUDMax = STB_PE_DIR_MAX_UD_INI;//The face on top/down allowable range max. 00124 handle->peFaceDirLRMin = STB_PE_DIR_MIN_LR_INI;//The face on left /right side allowable range min. 00125 handle->peFaceDirLRMax = STB_PE_DIR_MAX_LR_INI;//The face on left /right side allowable range max. 00126 handle->peFaceDirThr = STB_PE_DIR_THR_INI ;//If the confidence of Face direction estimation doesn't exceed the reference value, the recognition result isn't trusted. 00127 handle->peFrameCount = STB_PE_FRAME_CNT_INI ; 00128 handle->peCntMax = nTraCntMax ;//Maximum number of tracking people 00129 handle->pePtr = NULL; 00130 handle->peDet.num = 0; 00131 handle->peDet.fcDet = NULL; 00132 handle->peDetRec = NULL; 00133 handle->peRes.peCnt = 0; 00134 handle->peRes.peFace = NULL; 00135 handle->execFlg = NULL; 00136 00137 tmpVal = PeCalcPeSize ( nTraCntMax ); /*calculate necessary amount in the Pe handle*/ 00138 handle->pePtr = NULL; 00139 handle->pePtr = ( STB_INT8 * )malloc( tmpVal ); /*keeping necessary amount in the Pe handle*/ 00140 if( handle->pePtr == NULL ) 00141 { 00142 free ( handle->pePtr ); 00143 free ( handle ); 00144 return NULL; 00145 } 00146 00147 /*Malloc-area is allocated to things that need Malloc in TR handle*/ 00148 PeSharePeSize ( handle ); 00149 00150 for( t = 0 ; t < STB_PE_BACK_MAX ; t++ ) 00151 { 00152 handle->peDetRec [ t ].num = 0; 00153 for( i = 0 ; i < handle->peCntMax ; i++ ) 00154 { 00155 handle->peDetRec[t].fcDet[i].nDetID = STB_STATUS_NO_DATA ; 00156 handle->peDetRec[t].fcDet[i].nTraID = STB_STATUS_NO_DATA ; 00157 handle->peDetRec[t].fcDet[i].dirDetRoll = STB_STATUS_NO_DATA ; 00158 handle->peDetRec[t].fcDet[i].dirDetPitch = STB_STATUS_NO_DATA ; 00159 handle->peDetRec[t].fcDet[i].dirDetYaw = STB_STATUS_NO_DATA ; 00160 handle->peDetRec[t].fcDet[i].dirDetConf = STB_STATUS_NO_DATA ; 00161 handle->peDetRec[t].fcDet[i].ageDetVal = STB_STATUS_NO_DATA ; 00162 handle->peDetRec[t].fcDet[i].ageStatus = STB_STATUS_NO_DATA ; 00163 handle->peDetRec[t].fcDet[i].ageDetConf = STB_STATUS_NO_DATA ; 00164 handle->peDetRec[t].fcDet[i].genDetVal = STB_STATUS_NO_DATA ; 00165 handle->peDetRec[t].fcDet[i].genStatus = STB_STATUS_NO_DATA ; 00166 handle->peDetRec[t].fcDet[i].genDetConf = STB_STATUS_NO_DATA ; 00167 handle->peDetRec[t].fcDet[i].gazDetLR = STB_STATUS_NO_DATA ; 00168 handle->peDetRec[t].fcDet[i].gazDetUD = STB_STATUS_NO_DATA ; 00169 handle->peDetRec[t].fcDet[i].bliDetL = STB_STATUS_NO_DATA ; 00170 handle->peDetRec[t].fcDet[i].bliDetR = STB_STATUS_NO_DATA ; 00171 00172 handle->peDetRec[t].fcDet[i].expDetConf = STB_STATUS_NO_DATA ; 00173 for( j = 0 ; j < STB_EX_MAX ; j++) 00174 { 00175 handle->peDetRec[t].fcDet[i].expDetVal[ j ] = STB_STATUS_NO_DATA; 00176 } 00177 } 00178 } 00179 00180 handle->execFlg->pet = execFlg->pet ; 00181 handle->execFlg->hand = execFlg->hand ; 00182 handle->execFlg->bodyTr = execFlg->bodyTr ; 00183 handle->execFlg->faceTr = execFlg->faceTr ; 00184 handle->execFlg->gen = execFlg->gen ; 00185 handle->execFlg->age = execFlg->age ; 00186 handle->execFlg->fr = execFlg->fr ; 00187 handle->execFlg->exp = execFlg->exp ; 00188 handle->execFlg->gaz = execFlg->gaz ; 00189 handle->execFlg->dir = execFlg->dir ; 00190 handle->execFlg->bli = execFlg->bli ; 00191 00192 00193 return handle; 00194 } 00195 00196 /*--------------------------------------------------------------------- 00197 ---------------------------------------------------------------------*/ 00198 /*Delete handle*/ 00199 STB_INT32 PeDeleteHandle(PEHANDLE handle){ 00200 STB_INT32 nRet; 00201 /*NULL check*/ 00202 nRet = PeIsValidPointer(handle); 00203 if(nRet != STB_NORMAL){ 00204 return STB_ERR_NOHANDLE; 00205 } 00206 00207 free ( handle->pePtr ); 00208 free ( handle ); 00209 00210 return STB_NORMAL; 00211 } 00212 00213 /*--------------------------------------------------------------------- 00214 ---------------------------------------------------------------------*/ 00215 /*Set the result*/ 00216 STB_INT32 PeSetDetect(PEHANDLE handle,const STB_PE_DET *stbPeDet){ 00217 00218 STB_INT32 nRet; 00219 STB_INT32 i,j; 00220 00221 /*NULL check*/ 00222 nRet = PeIsValidPointer(handle); 00223 if(nRet != STB_NORMAL){ 00224 return STB_ERR_NOHANDLE; 00225 } 00226 00227 nRet = PeIsValidPointer(stbPeDet); 00228 if(nRet != STB_NORMAL){ 00229 return nRet; 00230 } 00231 00232 /*Input value check*/ 00233 nRet = STB_PeIsValidValue ( stbPeDet ,handle->execFlg ); 00234 if(nRet != STB_TRUE) 00235 { 00236 return STB_ERR_INVALIDPARAM; 00237 } 00238 00239 00240 /*Set the received result to the handle*/ 00241 /* Face */ 00242 if( stbPeDet->num > handle->peCntMax ) 00243 { 00244 return STB_ERR_PROCESSCONDITION; 00245 } 00246 00247 00248 handle->peDet.num = stbPeDet->num; 00249 for( i = 0 ; i < handle->peDet.num ; i++ ) 00250 { 00251 handle->peDet.fcDet[i].nDetID = stbPeDet->fcDet[i].nDetID ; 00252 handle->peDet.fcDet[i].nTraID = stbPeDet->fcDet[i].nTraID ; 00253 if( handle->execFlg->gen == STB_TRUE ) 00254 { 00255 handle->peDet.fcDet[i].genDetVal = stbPeDet->fcDet[i].genDetVal ; 00256 handle->peDet.fcDet[i].genDetConf = stbPeDet->fcDet[i].genDetConf ; 00257 handle->peDet.fcDet[i].genStatus = STB_STATUS_NO_DATA ; 00258 } 00259 if( handle->execFlg->age == STB_TRUE ) 00260 { 00261 handle->peDet.fcDet[i].ageDetVal = stbPeDet->fcDet[i].ageDetVal ; 00262 handle->peDet.fcDet[i].ageDetConf = stbPeDet->fcDet[i].ageDetConf ; 00263 handle->peDet.fcDet[i].ageStatus = STB_STATUS_NO_DATA ; 00264 } 00265 if( handle->execFlg->exp == STB_TRUE ) 00266 { 00267 handle->peDet.fcDet[i].expDetConf = stbPeDet->fcDet[i].expDetConf ; 00268 for( j = 0 ; j < STB_EX_MAX ; j++) 00269 { 00270 handle->peDet.fcDet[i].expDetVal[ j ] = stbPeDet->fcDet[i].expDetVal[ j ]; 00271 } 00272 } 00273 if( handle->execFlg->gaz == STB_TRUE ) 00274 { 00275 handle->peDet.fcDet[i].gazDetLR = stbPeDet->fcDet[i].gazDetLR ; 00276 handle->peDet.fcDet[i].gazDetUD = stbPeDet->fcDet[i].gazDetUD ; 00277 } 00278 //if( handle->execFlg->dir == STB_TRUE )// dir is obligation. 00279 //{ 00280 handle->peDet.fcDet[i].dirDetRoll = stbPeDet->fcDet[i].dirDetRoll ; 00281 handle->peDet.fcDet[i].dirDetPitch = stbPeDet->fcDet[i].dirDetPitch ; 00282 handle->peDet.fcDet[i].dirDetYaw = stbPeDet->fcDet[i].dirDetYaw ; 00283 handle->peDet.fcDet[i].dirDetConf = stbPeDet->fcDet[i].dirDetConf ; 00284 //} 00285 if( handle->execFlg->bli == STB_TRUE ) 00286 { 00287 handle->peDet.fcDet[i].bliDetL = stbPeDet->fcDet[i].bliDetL ; 00288 handle->peDet.fcDet[i].bliDetR = stbPeDet->fcDet[i].bliDetR ; 00289 } 00290 } 00291 00292 00293 return STB_NORMAL; 00294 } 00295 /*--------------------------------------------------------------------- 00296 ---------------------------------------------------------------------*/ 00297 /*Main process execution*/ 00298 STB_INT32 PeExecute(PEHANDLE handle){ 00299 STB_INT32 nRet; 00300 /*NULL check*/ 00301 nRet = PeIsValidPointer(handle); 00302 if(nRet != STB_NORMAL){ 00303 return STB_ERR_NOHANDLE; 00304 } 00305 00306 /*Main processing here*/ 00307 nRet = StbPeExec ( handle ); 00308 00309 00310 return STB_NORMAL; 00311 } 00312 /*--------------------------------------------------------------------- 00313 ---------------------------------------------------------------------*/ 00314 /*Get-Function of results*/ 00315 STB_INT32 PeGetResult( PEHANDLE handle, STB_PE_RES* peResult){ 00316 00317 STB_INT32 nRet; 00318 STB_INT32 i; 00319 00320 /*NULL check*/ 00321 nRet = PeIsValidPointer(handle); 00322 if(nRet != STB_NORMAL){ 00323 return STB_ERR_NOHANDLE; 00324 } 00325 00326 nRet = PeIsValidPointer(peResult); 00327 if(nRet != STB_NORMAL){ 00328 return nRet; 00329 } 00330 00331 /*Get result from handle*/ 00332 peResult->peCnt = handle->peRes.peCnt ; 00333 for( i = 0 ; i < peResult->peCnt ; i++ ) 00334 { 00335 peResult->peFace[i].nTraID = handle->peRes.peFace[i].nTraID ; 00336 peResult->peFace[i].gen.status = handle->peRes.peFace[i].gen.status ; 00337 peResult->peFace[i].gen.value = handle->peRes.peFace[i].gen.value ; 00338 peResult->peFace[i].gen.conf = handle->peRes.peFace[i].gen.conf ; 00339 peResult->peFace[i].age.status = handle->peRes.peFace[i].age.status ; 00340 peResult->peFace[i].age.value = handle->peRes.peFace[i].age.value ; 00341 peResult->peFace[i].age.conf = handle->peRes.peFace[i].age.conf ; 00342 peResult->peFace[i].exp.status = handle->peRes.peFace[i].exp.status ; 00343 peResult->peFace[i].exp.value = handle->peRes.peFace[i].exp.value ; 00344 peResult->peFace[i].exp.conf = handle->peRes.peFace[i].exp.conf ; 00345 peResult->peFace[i].gaz.status = handle->peRes.peFace[i].gaz.status ; 00346 peResult->peFace[i].gaz.LR = handle->peRes.peFace[i].gaz.LR ; 00347 peResult->peFace[i].gaz.UD = handle->peRes.peFace[i].gaz.UD ; 00348 peResult->peFace[i].gaz.conf = handle->peRes.peFace[i].gaz.conf ; 00349 peResult->peFace[i].dir.status = handle->peRes.peFace[i].dir.status ; 00350 peResult->peFace[i].dir.pitch = handle->peRes.peFace[i].dir.pitch ; 00351 peResult->peFace[i].dir.roll = handle->peRes.peFace[i].dir.roll ; 00352 peResult->peFace[i].dir.yaw = handle->peRes.peFace[i].dir.yaw ; 00353 peResult->peFace[i].dir.conf = handle->peRes.peFace[i].dir.conf ; 00354 peResult->peFace[i].bli.status = handle->peRes.peFace[i].bli.status ; 00355 peResult->peFace[i].bli.ratioL = handle->peRes.peFace[i].bli.ratioL ; 00356 peResult->peFace[i].bli.ratioR = handle->peRes.peFace[i].bli.ratioR ; 00357 } 00358 00359 00360 00361 return STB_NORMAL; 00362 } 00363 /*--------------------------------------------------------------------- 00364 ---------------------------------------------------------------------*/ 00365 STB_INT32 PeSetFaceDirMinMax(PEHANDLE handle , STB_INT32 nMinUDAngle , STB_INT32 nMaxUDAngle ,STB_INT32 nMinLRAngle , STB_INT32 nMaxLRAngle ) 00366 { 00367 00368 STB_INT32 nRet; 00369 nRet = PeIsValidPointer(handle); 00370 if(nRet != STB_NORMAL){ 00371 return STB_ERR_NOHANDLE; 00372 } 00373 00374 if( nMinUDAngle < STB_PE_DIR_MIN_UD_MIN || STB_PE_DIR_MIN_UD_MAX < nMinUDAngle) 00375 { 00376 return STB_ERR_INVALIDPARAM; 00377 } 00378 if( nMaxUDAngle < STB_PE_DIR_MAX_UD_MIN || STB_PE_DIR_MAX_UD_MAX < nMaxUDAngle) 00379 { 00380 return STB_ERR_INVALIDPARAM; 00381 } 00382 if( nMaxUDAngle < nMinUDAngle) 00383 { 00384 return STB_ERR_INVALIDPARAM; 00385 } 00386 00387 00388 if( nMinLRAngle < STB_PE_DIR_MIN_LR_MIN || STB_PE_DIR_MIN_LR_MAX < nMinLRAngle) 00389 { 00390 return STB_ERR_INVALIDPARAM; 00391 } 00392 if( nMaxLRAngle < STB_PE_DIR_MAX_LR_MIN || STB_PE_DIR_MAX_LR_MAX < nMaxLRAngle) 00393 { 00394 return STB_ERR_INVALIDPARAM; 00395 } 00396 if( nMaxLRAngle < nMinLRAngle) 00397 { 00398 return STB_ERR_INVALIDPARAM; 00399 } 00400 00401 handle->peFaceDirUDMin = nMinUDAngle; 00402 handle->peFaceDirUDMax = nMaxUDAngle; 00403 handle->peFaceDirLRMin = nMinLRAngle; 00404 handle->peFaceDirLRMax = nMaxLRAngle; 00405 00406 00407 return STB_NORMAL; 00408 } 00409 /*--------------------------------------------------------------------- 00410 ---------------------------------------------------------------------*/ 00411 STB_INT32 PeGetFaceDirMinMax(PEHANDLE handle ,STB_INT32 *pnMinUDAngle, STB_INT32 *pnMaxUDAngle ,STB_INT32 *pnMinLRAngle, STB_INT32 *pnMaxLRAngle ) 00412 { 00413 STB_INT32 nRet; 00414 nRet = PeIsValidPointer(handle); 00415 if(nRet != STB_NORMAL){ 00416 return STB_ERR_NOHANDLE; 00417 } 00418 nRet = PeIsValidPointer(pnMinUDAngle); 00419 if(nRet != STB_NORMAL){ 00420 return STB_ERR_INVALIDPARAM; 00421 } 00422 nRet = PeIsValidPointer(pnMaxUDAngle); 00423 if(nRet != STB_NORMAL){ 00424 return STB_ERR_INVALIDPARAM; 00425 } 00426 nRet = PeIsValidPointer(pnMinLRAngle); 00427 if(nRet != STB_NORMAL){ 00428 return STB_ERR_INVALIDPARAM; 00429 } 00430 nRet = PeIsValidPointer(pnMaxLRAngle); 00431 if(nRet != STB_NORMAL){ 00432 return STB_ERR_INVALIDPARAM; 00433 } 00434 00435 *pnMinUDAngle = handle->peFaceDirUDMin ; 00436 *pnMaxUDAngle = handle->peFaceDirUDMax ; 00437 00438 *pnMinLRAngle = handle->peFaceDirLRMin ; 00439 *pnMaxLRAngle = handle->peFaceDirLRMax ; 00440 00441 return STB_NORMAL; 00442 } 00443 /*--------------------------------------------------------------------- 00444 ---------------------------------------------------------------------*/ 00445 STB_INT32 PeClear ( PEHANDLE handle ) 00446 { 00447 //clear processing 00448 STB_INT32 t , i ,j; 00449 STB_INT32 nRet; 00450 00451 /*NULL check*/ 00452 nRet = PeIsValidPointer(handle); 00453 if(nRet != STB_NORMAL){ 00454 return STB_ERR_NOHANDLE; 00455 } 00456 00457 for( t = 0 ; t < STB_PE_BACK_MAX ; t++ ) 00458 { 00459 handle->peDetRec [ t ].num = 0; 00460 for( i = 0 ; i < handle->peCntMax ; i++ ) 00461 { 00462 handle->peDetRec[t].fcDet[i].nDetID = STB_STATUS_NO_DATA ; 00463 handle->peDetRec[t].fcDet[i].nTraID = STB_STATUS_NO_DATA ; 00464 if( handle->execFlg->dir == STB_TRUE ) 00465 { 00466 handle->peDetRec[t].fcDet[i].dirDetRoll = STB_STATUS_NO_DATA ; 00467 handle->peDetRec[t].fcDet[i].dirDetPitch = STB_STATUS_NO_DATA ; 00468 handle->peDetRec[t].fcDet[i].dirDetYaw = STB_STATUS_NO_DATA ; 00469 handle->peDetRec[t].fcDet[i].dirDetConf = STB_STATUS_NO_DATA ; 00470 } 00471 if( handle->execFlg->age == STB_TRUE ) 00472 { 00473 handle->peDetRec[t].fcDet[i].ageDetVal = STB_STATUS_NO_DATA ; 00474 handle->peDetRec[t].fcDet[i].ageStatus = STB_STATUS_NO_DATA ; 00475 handle->peDetRec[t].fcDet[i].ageDetConf = STB_STATUS_NO_DATA ; 00476 } 00477 if( handle->execFlg->gen == STB_TRUE ) 00478 { 00479 handle->peDetRec[t].fcDet[i].genDetVal = STB_STATUS_NO_DATA ; 00480 handle->peDetRec[t].fcDet[i].genStatus = STB_STATUS_NO_DATA ; 00481 handle->peDetRec[t].fcDet[i].genDetConf = STB_STATUS_NO_DATA ; 00482 } 00483 if( handle->execFlg->gaz == STB_TRUE ) 00484 { 00485 handle->peDetRec[t].fcDet[i].gazDetLR = STB_STATUS_NO_DATA ; 00486 handle->peDetRec[t].fcDet[i].gazDetUD = STB_STATUS_NO_DATA ; 00487 } 00488 if( handle->execFlg->bli == STB_TRUE ) 00489 { 00490 handle->peDetRec[t].fcDet[i].bliDetL = STB_STATUS_NO_DATA ; 00491 handle->peDetRec[t].fcDet[i].bliDetR = STB_STATUS_NO_DATA ; 00492 } 00493 if( handle->execFlg->exp == STB_TRUE ) 00494 { 00495 handle->peDetRec[t].fcDet[i].expDetConf = STB_STATUS_NO_DATA ; 00496 for( j = 0 ; j < STB_EX_MAX ; j++) 00497 { 00498 handle->peDetRec[t].fcDet[i].expDetVal[j] = STB_STATUS_NO_DATA ; 00499 } 00500 } 00501 } 00502 } 00503 00504 return STB_NORMAL; 00505 } 00506 /*--------------------------------------------------------------------- 00507 ---------------------------------------------------------------------*/ 00508 STB_INT32 PeSetFaceDirThreshold(PEHANDLE handle , STB_INT32 threshold ) 00509 { 00510 STB_INT32 nRet; 00511 nRet = PeIsValidPointer(handle); 00512 if(nRet != STB_NORMAL){ 00513 return STB_ERR_NOHANDLE; 00514 } 00515 if( threshold < STB_PE_DIR_THR_MIN || STB_PE_DIR_THR_MAX < threshold ){ 00516 return STB_ERR_INVALIDPARAM; 00517 } 00518 00519 handle->peFaceDirThr = threshold; 00520 return STB_NORMAL; 00521 } 00522 /*--------------------------------------------------------------------- 00523 ---------------------------------------------------------------------*/ 00524 STB_INT32 PeGetFaceDirThreshold(PEHANDLE handle , STB_INT32* threshold ) 00525 { 00526 STB_INT32 nRet; 00527 nRet = PeIsValidPointer(handle); 00528 if(nRet != STB_NORMAL){ 00529 return STB_ERR_NOHANDLE; 00530 } 00531 nRet = PeIsValidPointer(threshold); 00532 if(nRet != STB_NORMAL){ 00533 return STB_ERR_INVALIDPARAM; 00534 } 00535 00536 *threshold = handle->peFaceDirThr ; 00537 return STB_NORMAL; 00538 } 00539 /*--------------------------------------------------------------------- 00540 ---------------------------------------------------------------------*/ 00541 STB_INT32 PeSetFrameCount(PEHANDLE handle , STB_INT32 nFrameCount ) 00542 { 00543 00544 STB_INT32 nRet; 00545 nRet = PeIsValidPointer(handle); 00546 if(nRet != STB_NORMAL){ 00547 return STB_ERR_NOHANDLE; 00548 } 00549 00550 if( nFrameCount < 1 || nFrameCount > STB_PE_BACK_MAX ) 00551 { 00552 return STB_ERR_INVALIDPARAM; 00553 } 00554 00555 handle->peFrameCount = nFrameCount; 00556 return STB_NORMAL; 00557 } 00558 /*--------------------------------------------------------------------- 00559 ---------------------------------------------------------------------*/ 00560 STB_INT32 PeGetFrameCount(PEHANDLE handle , STB_INT32* nFrameCount ) 00561 { 00562 STB_INT32 nRet; 00563 nRet = PeIsValidPointer(handle); 00564 if(nRet != STB_NORMAL){ 00565 return STB_ERR_NOHANDLE; 00566 } 00567 nRet = PeIsValidPointer(nFrameCount); 00568 if(nRet != STB_NORMAL){ 00569 return STB_ERR_INVALIDPARAM; 00570 } 00571 00572 *nFrameCount = handle->peFrameCount ; 00573 return STB_NORMAL; 00574 }
Generated on Wed Jul 13 2022 14:40:13 by
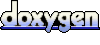