
Mar. 14. 2018
Dependencies: GraphicsFramework GR-PEACH_video LCD_shield_config AsciiFont R_BSP USBHost_custom
FrInterface.h
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #if !defined( _INTERFACE_H_ ) 00018 #define _INTERFACE_H_ 00019 00020 #include "STBFrTypedef.h" 00021 #include "STBCommonDef.h" 00022 #include "STBCommonType.h" 00023 #include "STBFrValidValue.h" 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 00030 ////////////////////////////////////////////////////////////////////////////////// 00031 ////////////////////////////////////////////////////////////////////////////////// 00032 /////////// Define ////////////// 00033 ////////////////////////////////////////////////////////////////////////////////// 00034 ////////////////////////////////////////////////////////////////////////////////// 00035 00036 00037 #define STB_FR_BACK_MAX 20 /* refer to past "STB_BACK_MAX" frames of results */ 00038 00039 #define STB_FR_TRA_CNT_MAX 35 00040 00041 #define STB_FR_INVALID_UID -999 00042 00043 #define STB_FR_DIR_MIN_UD_INI -15 00044 #define STB_FR_DIR_MIN_UD_MIN -90 00045 #define STB_FR_DIR_MIN_UD_MAX 90 00046 00047 #define STB_FR_DIR_MAX_UD_INI 20 00048 #define STB_FR_DIR_MAX_UD_MIN -90 00049 #define STB_FR_DIR_MAX_UD_MAX 90 00050 00051 #define STB_FR_DIR_MIN_LR_INI -30 00052 #define STB_FR_DIR_MIN_LR_MIN -90 00053 #define STB_FR_DIR_MIN_LR_MAX 90 00054 00055 #define STB_FR_DIR_MAX_LR_INI 30 00056 #define STB_FR_DIR_MAX_LR_MIN -90 00057 #define STB_FR_DIR_MAX_LR_MAX 90 00058 00059 #define STB_FR_DIR_THR_INI 300 00060 #define STB_FR_DIR_THR_MIN 0 00061 #define STB_FR_DIR_THR_MAX 1000 00062 00063 #define STB_FR_FRAME_CNT_INI 5 00064 #define STB_FR_FRAME_CNT_MIN 0 00065 #define STB_FR_FRAME_CNT_MAX 20 00066 00067 #define STB_FR_FRAME_RATIO_INI 60 00068 #define STB_FR_FRAME_RATIO_MIN 0 00069 #define STB_FR_FRAME_RATIO_MAX 100 00070 00071 ////////////////////////////////////////////////////////////////////////////////// 00072 ////////////////////////////////////////////////////////////////////////////////// 00073 /////////// Struct ////////////// 00074 ////////////////////////////////////////////////////////////////////////////////// 00075 ////////////////////////////////////////////////////////////////////////////////// 00076 typedef struct tagFRHANDLE { 00077 00078 STB_INT8 *frPtr ; 00079 00080 /* param */ 00081 STB_INT32 frCntMax ;//Maximum number of tracking people 00082 STB_INT32 frFaceDirUDMax ;//The face on top/down allowable range max. 00083 STB_INT32 frFaceDirUDMin ;//The face on top/down allowable range min. 00084 STB_INT32 frFaceDirLRMax ;//The face on left /right side allowable range max. 00085 STB_INT32 frFaceDirLRMin ;//The face on left /right side allowable range min. 00086 STB_INT32 frFaceDirThr ;//If the confidence of Face direction estimation doesn't exceed the reference value, the recognition result isn't trusted. 00087 STB_INT32 frFrameCount ; 00088 STB_INT32 frFrameRatio ; 00089 /* FR_Face */ 00090 STB_FR_DET frDet ;//Present data before the stabilization(input). 00091 STB_FR_DET *frDetRec ;//past data before the stabilization 00092 STB_FR_RES frRes ;//present data after the stabilization(output) 00093 00094 } *FRHANDLE; 00095 00096 00097 ////////////////////////////////////////////////////////////////////////////////// 00098 ////////////////////////////////////////////////////////////////////////////////// 00099 /////////// Func ////////////// 00100 ////////////////////////////////////////////////////////////////////////////////// 00101 ////////////////////////////////////////////////////////////////////////////////// 00102 FRHANDLE FrCreateHandle ( const STB_INT32 nTraCntMax ); 00103 STB_INT32 FrDeleteHandle ( FRHANDLE handle); 00104 STB_INT32 FrSetDetect ( FRHANDLE handle,const STB_FR_DET *stbPeDet); 00105 STB_INT32 FrExecute ( FRHANDLE handle); 00106 STB_INT32 FrClear ( FRHANDLE handle ); 00107 STB_INT32 FrGetResult ( FRHANDLE handle , STB_FR_RES* peResult); 00108 00109 STB_INT32 FrSetFaceDirMinMax( FRHANDLE handle , STB_INT32 nMinUDAngle , STB_INT32 nMaxUDAngle ,STB_INT32 nMinLRAngle , STB_INT32 nMaxLRAngle); 00110 STB_INT32 FrGetFaceDirMinMax( FRHANDLE handle , STB_INT32 *pnMinUDAngle , STB_INT32 *pnMaxUDAngle ,STB_INT32 *pnMinLRAngle, STB_INT32 *pnMaxLRAngle ); 00111 STB_INT32 FrSetFaceDirThreshold ( FRHANDLE handle , STB_INT32 threshold ); 00112 STB_INT32 FrGetFaceDirThreshold ( FRHANDLE handle , STB_INT32* threshold ); 00113 STB_INT32 FrSetFrameCount ( FRHANDLE handle , STB_INT32 nFrameCount ); 00114 STB_INT32 FrGetFrameCount ( FRHANDLE handle , STB_INT32* nFrameCount ); 00115 STB_INT32 FrSetMinRatio ( FRHANDLE handle , STB_INT32 nMinRatio ); 00116 STB_INT32 FrGetMinRatio ( FRHANDLE handle , STB_INT32* nMinRatio ); 00117 #ifdef __cplusplus 00118 } 00119 #endif 00120 00121 #endif
Generated on Wed Jul 13 2022 14:40:13 by
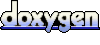