libuav original
Dependents: UAVCAN UAVCAN_Subscriber
wwdt_11xx.h
00001 /* 00002 * @brief LPC11xx WWDT chip driver 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2012 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __WWDT_11XX_H_ 00033 #define __WWDT_11XX_H_ 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif 00038 00039 /** @defgroup WWDT_11XX CHIP: LPC11xx Windowed Watchdog driver 00040 * @ingroup CHIP_11XX_Drivers 00041 * @{ 00042 */ 00043 00044 #if !defined(CHIP_LPC11CXX) || defined(CHIP_LPC1125) 00045 #define WATCHDOG_WINDOW_SUPPORT 00046 #endif 00047 00048 #if defined(CHIP_LPC11AXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11UXX) 00049 #define WATCHDOG_CLKSEL_SUPPORT 00050 #endif 00051 00052 /** 00053 * @brief Windowed Watchdog register block structure 00054 */ 00055 typedef struct { /*!< WWDT Structure */ 00056 __IO uint32_t MOD ; /*!< Watchdog mode register. This register contains the basic mode and status of the Watchdog Timer. */ 00057 __IO uint32_t TC ; /*!< Watchdog timer constant register. This register determines the time-out value. */ 00058 __O uint32_t FEED ; /*!< Watchdog feed sequence register. Writing 0xAA followed by 0x55 to this register reloads the Watchdog timer with the value contained in WDTC. */ 00059 __I uint32_t TV ; /*!< Watchdog timer value register. This register reads out the current value of the Watchdog timer. */ 00060 #ifdef WATCHDOG_CLKSEL_SUPPORT 00061 __IO uint32_t CLKSEL ; /*!< Watchdog clock select register. */ 00062 #else 00063 __I uint32_t RESERVED0; 00064 #endif 00065 #ifdef WATCHDOG_WINDOW_SUPPORT 00066 __IO uint32_t WARNINT ; /*!< Watchdog warning interrupt register. This register contains the Watchdog warning interrupt compare value. */ 00067 __IO uint32_t WINDOW ; /*!< Watchdog timer window register. This register contains the Watchdog window value. */ 00068 #endif 00069 } LPC_WWDT_T; 00070 00071 /** 00072 * @brief Watchdog Mode register definitions 00073 */ 00074 /** Watchdog Mode Bitmask */ 00075 #define WWDT_WDMOD_BITMASK ((uint32_t) 0x1F) 00076 /** WWDT interrupt enable bit */ 00077 #define WWDT_WDMOD_WDEN ((uint32_t) (1 << 0)) 00078 /** WWDT interrupt enable bit */ 00079 #define WWDT_WDMOD_WDRESET ((uint32_t) (1 << 1)) 00080 /** WWDT time out flag bit */ 00081 #define WWDT_WDMOD_WDTOF ((uint32_t) (1 << 2)) 00082 /** WDT Time Out flag bit */ 00083 #define WWDT_WDMOD_WDINT ((uint32_t) (1 << 3)) 00084 /** WWDT Protect flag bit */ 00085 #define WWDT_WDMOD_WDPROTECT ((uint32_t) (1 << 4)) 00086 00087 /** 00088 * @brief Initialize the Watchdog timer 00089 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00090 * @return None 00091 */ 00092 void Chip_WWDT_Init(LPC_WWDT_T *pWWDT); 00093 00094 /** 00095 * @brief Shutdown the Watchdog timer 00096 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00097 * @return None 00098 */ 00099 void Chip_WWDT_DeInit(LPC_WWDT_T *pWWDT); 00100 00101 /** 00102 * @brief Set WDT timeout constant value used for feed 00103 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00104 * @param timeout : WDT timeout in ticks, between WWDT_TICKS_MIN and WWDT_TICKS_MAX 00105 * @return none 00106 */ 00107 STATIC INLINE void Chip_WWDT_SetTimeOut(LPC_WWDT_T *pWWDT, uint32_t timeout) 00108 { 00109 pWWDT->TC = timeout; 00110 } 00111 00112 /** 00113 * @brief Feed watchdog timer 00114 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00115 * @return None 00116 * @note If this function isn't called, a watchdog timer warning will occur. 00117 * After the warning, a timeout will occur if a feed has happened. 00118 */ 00119 STATIC INLINE void Chip_WWDT_Feed(LPC_WWDT_T *pWWDT) 00120 { 00121 pWWDT->FEED = 0xAA; 00122 pWWDT->FEED = 0x55; 00123 } 00124 00125 #if defined(WATCHDOG_WINDOW_SUPPORT) 00126 /** 00127 * @brief Set WWDT warning interrupt 00128 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00129 * @param timeout : WDT warning in ticks, between 0 and 1023 00130 * @return None 00131 * @note This is the number of ticks after the watchdog interrupt that the 00132 * warning interrupt will be generated. 00133 */ 00134 STATIC INLINE void Chip_WWDT_SetWarning(LPC_WWDT_T *pWWDT, uint32_t timeout) 00135 { 00136 pWWDT->WARNINT = timeout; 00137 } 00138 00139 /** 00140 * @brief Set WWDT window time 00141 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00142 * @param timeout : WDT timeout in ticks, between WWDT_TICKS_MIN and WWDT_TICKS_MAX 00143 * @return None 00144 * @note The watchdog timer must be fed between the timeout from the Chip_WWDT_SetTimeOut() 00145 * function and this function, with this function defining the last tick before the 00146 * watchdog window interrupt occurs. 00147 */ 00148 STATIC INLINE void Chip_WWDT_SetWindow(LPC_WWDT_T *pWWDT, uint32_t timeout) 00149 { 00150 pWWDT->WINDOW = timeout; 00151 } 00152 00153 #endif 00154 00155 /** 00156 * @brief Enable watchdog timer options 00157 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00158 * @param options : An or'ed set of options of values 00159 * WWDT_WDMOD_WDEN, WWDT_WDMOD_WDRESET, and WWDT_WDMOD_WDPROTECT 00160 * @return None 00161 * @note You can enable more than one option at once (ie, WWDT_WDMOD_WDRESET | 00162 * WWDT_WDMOD_WDPROTECT), but use the WWDT_WDMOD_WDEN after all other options 00163 * are set (or unset) with no other options. If WWDT_WDMOD_LOCK is used, it cannot 00164 * be unset. 00165 */ 00166 STATIC INLINE void Chip_WWDT_SetOption(LPC_WWDT_T *pWWDT, uint32_t options) 00167 { 00168 pWWDT->MOD |= options; 00169 } 00170 00171 /** 00172 * @brief Disable/clear watchdog timer options 00173 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00174 * @param options : An or'ed set of options of values 00175 * WWDT_WDMOD_WDEN, WWDT_WDMOD_WDRESET, and WWDT_WDMOD_WDPROTECT 00176 * @return None 00177 * @note You can disable more than one option at once (ie, WWDT_WDMOD_WDRESET | 00178 * WWDT_WDMOD_WDTOF). 00179 */ 00180 STATIC INLINE void Chip_WWDT_UnsetOption(LPC_WWDT_T *pWWDT, uint32_t options) 00181 { 00182 pWWDT->MOD &= (~options) & WWDT_WDMOD_BITMASK; 00183 } 00184 00185 /** 00186 * @brief Enable WWDT activity 00187 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00188 * @return None 00189 */ 00190 STATIC INLINE void Chip_WWDT_Start(LPC_WWDT_T *pWWDT) 00191 { 00192 Chip_WWDT_SetOption(pWWDT, WWDT_WDMOD_WDEN); 00193 Chip_WWDT_Feed(pWWDT); 00194 } 00195 00196 /** 00197 * @brief Read WWDT status flag 00198 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00199 * @return Watchdog status, an Or'ed value of WWDT_WDMOD_* 00200 */ 00201 STATIC INLINE uint32_t Chip_WWDT_GetStatus(LPC_WWDT_T *pWWDT) 00202 { 00203 return pWWDT->MOD ; 00204 } 00205 00206 /** 00207 * @brief Clear WWDT interrupt status flags 00208 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00209 * @param status : Or'ed value of status flag(s) that you want to clear, should be: 00210 * - WWDT_WDMOD_WDTOF: Clear watchdog timeout flag 00211 * - WWDT_WDMOD_WDINT: Clear watchdog warning flag 00212 * @return None 00213 */ 00214 void Chip_WWDT_ClearStatusFlag(LPC_WWDT_T *pWWDT, uint32_t status); 00215 00216 /** 00217 * @brief Get the current value of WDT 00218 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00219 * @return current value of WDT 00220 */ 00221 STATIC INLINE uint32_t Chip_WWDT_GetCurrentCount(LPC_WWDT_T *pWWDT) 00222 { 00223 return pWWDT->TV ; 00224 } 00225 00226 #if defined(WATCHDOG_CLKSEL_SUPPORT) 00227 /** 00228 * @brief Watchdog Timer Clock Source Selection register definitions 00229 */ 00230 /** Clock source select bitmask */ 00231 #define WWDT_CLKSEL_BITMASK ((uint32_t) 0x10000003) 00232 /** Clock source select */ 00233 #define WWDT_CLKSEL_SOURCE(n) ((uint32_t) (n & 0x03)) 00234 /** Lock the clock source selection */ 00235 #define WWDT_CLKSEL_LOCK ((uint32_t) (1 << 31)) 00236 00237 /** 00238 * @brief Watchdog Clock Source definitions 00239 */ 00240 typedef enum { 00241 WWDT_CLKSRC_IRC = WWDT_CLKSEL_SOURCE(0), /*!< Internal RC oscillator */ 00242 WWDT_CLKSRC_WATCHDOG_WDOSC = WWDT_CLKSEL_SOURCE(1), /*!< Watchdog oscillator (WDOSC) */ 00243 } CHIP_WWDT_CLK_SRC_T; 00244 00245 /** 00246 * @brief Get the current value of WDT 00247 * @param pWWDT : The base of WatchDog Timer peripheral on the chip 00248 * @param wdtClkSrc : Selected watchdog clock source 00249 * @return Nothing 00250 */ 00251 STATIC INLINE void Chip_WWDT_SelClockSource(LPC_WWDT_T *pWWDT, CHIP_WWDT_CLK_SRC_T wdtClkSrc) 00252 { 00253 pWWDT->CLKSEL = wdtClkSrc & WWDT_CLKSEL_BITMASK; 00254 } 00255 00256 #endif 00257 00258 /** 00259 * @} 00260 */ 00261 00262 #ifdef __cplusplus 00263 } 00264 #endif 00265 00266 #endif /* __WWDT_11XX_H_ */
Generated on Tue Jul 12 2022 17:17:35 by
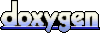