libuav original
Dependents: UAVCAN UAVCAN_Subscriber
uc_dynamic_memory.cpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include <uavcan/dynamic_memory.hpp> 00006 00007 namespace uavcan 00008 { 00009 /* 00010 * LimitedPoolAllocator 00011 */ 00012 void* LimitedPoolAllocator::allocate(std::size_t size) 00013 { 00014 if (used_blocks_ < max_blocks_) 00015 { 00016 used_blocks_++; 00017 return allocator_.allocate(size); 00018 } 00019 else 00020 { 00021 return UAVCAN_NULLPTR; 00022 } 00023 } 00024 00025 void LimitedPoolAllocator::deallocate(const void* ptr) 00026 { 00027 allocator_.deallocate(ptr); 00028 00029 UAVCAN_ASSERT(used_blocks_ > 0); 00030 if (used_blocks_ > 0) 00031 { 00032 used_blocks_--; 00033 } 00034 } 00035 00036 uint16_t LimitedPoolAllocator::getBlockCapacity() const 00037 { 00038 return min(max_blocks_, allocator_.getBlockCapacity()); 00039 } 00040 00041 }
Generated on Tue Jul 12 2022 17:17:35 by
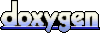