libuav original
Dependents: UAVCAN UAVCAN_Subscriber
type_util.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_MARSHAL_TYPE_UTIL_HPP_INCLUDED 00006 #define UAVCAN_MARSHAL_TYPE_UTIL_HPP_INCLUDED 00007 00008 #include <uavcan/build_config.hpp> 00009 #include <uavcan/util/templates.hpp> 00010 #include <uavcan/util/comparison.hpp> 00011 00012 namespace uavcan 00013 { 00014 /** 00015 * Read the specs to learn more about cast modes. 00016 */ 00017 enum CastMode { CastModeSaturate, CastModeTruncate }; 00018 00019 /** 00020 * Read the specs to learn more about tail array optimizations. 00021 */ 00022 enum TailArrayOptimizationMode { TailArrayOptDisabled, TailArrayOptEnabled }; 00023 00024 /** 00025 * Compile-time: Returns the number of bits needed to represent an integer value. 00026 */ 00027 template <unsigned long Num> 00028 struct IntegerBitLen 00029 { 00030 enum { Result = 1 + IntegerBitLen<(Num >> 1)>::Result }; 00031 }; 00032 template <> 00033 struct IntegerBitLen<0> 00034 { 00035 enum { Result = 0 }; 00036 }; 00037 00038 /** 00039 * Compile-time: Returns the number of bytes needed to contain the given number of bits. Assumes 1 byte == 8 bit. 00040 */ 00041 template <unsigned long BitLen> 00042 struct BitLenToByteLen 00043 { 00044 enum { Result = (BitLen + 7) / 8 }; 00045 }; 00046 00047 /** 00048 * Compile-time: Helper for integer and float specialization classes. Returns the platform-specific storage type. 00049 */ 00050 template <typename T, typename Enable = void> 00051 struct StorageType 00052 { 00053 typedef T Type; 00054 }; 00055 template <typename T> 00056 struct StorageType<T, typename EnableIfType<typename T::StorageType>::Type> 00057 { 00058 typedef typename T::StorageType Type; 00059 }; 00060 00061 /** 00062 * Compile-time: Whether T is a primitive type on this platform. 00063 */ 00064 template <typename T> 00065 class IsPrimitiveType 00066 { 00067 typedef char Yes; 00068 struct No { Yes dummy[8]; }; 00069 00070 template <typename U> 00071 static typename EnableIf<U::IsPrimitive, Yes>::Type test(int); 00072 00073 template <typename> 00074 static No test(...); 00075 00076 public: 00077 enum { Result = sizeof(test<T>(0)) == sizeof(Yes) }; 00078 }; 00079 00080 /** 00081 * Streams a given value into YAML string. Please see the specializations. 00082 */ 00083 template <typename T> 00084 class UAVCAN_EXPORT YamlStreamer; 00085 00086 } 00087 00088 #endif // UAVCAN_MARSHAL_TYPE_UTIL_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:35 by
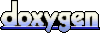