libuav original
Dependents: UAVCAN UAVCAN_Subscriber
transfer.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_TRANSPORT_TRANSFER_HPP_INCLUDED 00006 #define UAVCAN_TRANSPORT_TRANSFER_HPP_INCLUDED 00007 00008 #include <cassert> 00009 #include <uavcan/build_config.hpp> 00010 #include <uavcan/util/templates.hpp> 00011 #include <uavcan/std.hpp> 00012 00013 namespace uavcan 00014 { 00015 00016 static const unsigned GuaranteedPayloadLenPerFrame = 7; ///< Guaranteed for all transfers, all CAN standards 00017 00018 enum TransferType 00019 { 00020 TransferTypeServiceResponse = 0, 00021 TransferTypeServiceRequest = 1, 00022 TransferTypeMessageBroadcast = 2 00023 }; 00024 00025 static const uint8_t NumTransferTypes = 3; 00026 00027 00028 class UAVCAN_EXPORT TransferPriority 00029 { 00030 uint8_t value_; 00031 00032 public: 00033 static const uint8_t BitLen = 5U; 00034 static const uint8_t NumericallyMax = (1U << BitLen) - 1; 00035 static const uint8_t NumericallyMin = 0; 00036 00037 /// This priority is used by default 00038 static const TransferPriority Default; 00039 static const TransferPriority MiddleLower; 00040 static const TransferPriority OneHigherThanLowest; 00041 static const TransferPriority OneLowerThanHighest; 00042 static const TransferPriority Lowest; 00043 00044 TransferPriority() : value_(0xFF) { } 00045 00046 TransferPriority(uint8_t value) // Implicit 00047 : value_(value) 00048 { 00049 UAVCAN_ASSERT(isValid()); 00050 } 00051 00052 template <uint8_t Percent> 00053 static TransferPriority fromPercent() 00054 { 00055 StaticAssert<(Percent <= 100)>::check(); 00056 enum { Result = ((100U - Percent) * NumericallyMax) / 100U }; 00057 StaticAssert<(Result <= NumericallyMax)>::check(); 00058 StaticAssert<(Result >= NumericallyMin)>::check(); 00059 return TransferPriority(Result); 00060 } 00061 00062 uint8_t get() const { return value_; } 00063 00064 bool isValid() const { return value_ < (1U << BitLen); } 00065 00066 bool operator!=(TransferPriority rhs) const { return value_ != rhs.value_; } 00067 bool operator==(TransferPriority rhs) const { return value_ == rhs.value_; } 00068 }; 00069 00070 00071 class UAVCAN_EXPORT TransferID 00072 { 00073 uint8_t value_; 00074 00075 public: 00076 static const uint8_t BitLen = 5U; 00077 static const uint8_t Max = (1U << BitLen) - 1U; 00078 static const uint8_t Half = (1U << BitLen) / 2U; 00079 00080 TransferID() 00081 : value_(0) 00082 { } 00083 00084 TransferID(uint8_t value) // implicit 00085 : value_(value) 00086 { 00087 value_ &= Max; 00088 UAVCAN_ASSERT(value == value_); 00089 } 00090 00091 bool operator!=(TransferID rhs) const { return !operator==(rhs); } 00092 bool operator==(TransferID rhs) const { return get() == rhs.get(); } 00093 00094 void increment() 00095 { 00096 value_ = (value_ + 1) & Max; 00097 } 00098 00099 uint8_t get() const 00100 { 00101 UAVCAN_ASSERT(value_ <= Max); 00102 return value_; 00103 } 00104 00105 /** 00106 * Amount of increment() calls to reach rhs value. 00107 */ 00108 int computeForwardDistance(TransferID rhs) const; 00109 }; 00110 00111 00112 class UAVCAN_EXPORT NodeID 00113 { 00114 static const uint8_t ValueBroadcast = 0; 00115 static const uint8_t ValueInvalid = 0xFF; 00116 uint8_t value_; 00117 00118 public: 00119 static const uint8_t BitLen = 7U; 00120 static const uint8_t Max = (1U << BitLen) - 1U; 00121 static const uint8_t MaxRecommendedForRegularNodes = Max - 2; 00122 static const NodeID Broadcast; 00123 00124 NodeID() : value_(ValueInvalid) { } 00125 00126 NodeID(uint8_t value) // Implicit 00127 : value_(value) 00128 { 00129 UAVCAN_ASSERT(isValid()); 00130 } 00131 00132 uint8_t get() const { return value_; } 00133 00134 bool isValid() const { return value_ <= Max; } 00135 bool isBroadcast() const { return value_ == ValueBroadcast; } 00136 bool isUnicast() const { return (value_ <= Max) && (value_ != ValueBroadcast); } 00137 00138 bool operator!=(NodeID rhs) const { return !operator==(rhs); } 00139 bool operator==(NodeID rhs) const { return value_ == rhs.value_; } 00140 00141 bool operator<(NodeID rhs) const { return value_ < rhs.value_; } 00142 bool operator>(NodeID rhs) const { return value_ > rhs.value_; } 00143 bool operator<=(NodeID rhs) const { return value_ <= rhs.value_; } 00144 bool operator>=(NodeID rhs) const { return value_ >= rhs.value_; } 00145 }; 00146 00147 } 00148 00149 #endif // UAVCAN_TRANSPORT_TRANSFER_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:35 by
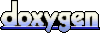