libuav original
Dependents: UAVCAN UAVCAN_Subscriber
test_posix.cpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include <uavcan_posix/dynamic_node_id_server/file_event_tracer.hpp> 00006 #include <uavcan_posix/dynamic_node_id_server/file_storage_backend.hpp> 00007 #include <uavcan_linux/uavcan_linux.hpp> 00008 #include <iostream> 00009 #include <iomanip> 00010 #include "debug.hpp" 00011 00012 int main(int argc, const char** argv) 00013 { 00014 (void)argc; 00015 (void)argv; 00016 try 00017 { 00018 ENFORCE(0 == std::system("mkdir -p /tmp/uavcan_posix/dynamic_node_id_server")); 00019 00020 /* 00021 * Event tracer test 00022 */ 00023 { 00024 using namespace uavcan::dynamic_node_id_server; 00025 00026 const std::string event_log_file("/tmp/uavcan_posix/dynamic_node_id_server/event.log"); 00027 00028 uavcan_posix::dynamic_node_id_server::FileEventTracer tracer; 00029 ENFORCE(0 <= tracer.init(event_log_file.c_str())); 00030 00031 // Adding a line 00032 static_cast<IEventTracer&>(tracer).onEvent(TraceError, 123456); 00033 ENFORCE(0 == std::system(("cat " + event_log_file).c_str())); 00034 00035 // Removing the log file 00036 ENFORCE(0 == std::system(("rm -f " + event_log_file).c_str())); 00037 00038 // Adding another line 00039 static_cast<IEventTracer&>(tracer).onEvent(TraceError, 789123); 00040 ENFORCE(0 == std::system(("cat " + event_log_file).c_str())); 00041 } 00042 00043 /* 00044 * Storage backend test 00045 */ 00046 { 00047 using namespace uavcan::dynamic_node_id_server; 00048 00049 uavcan_posix::dynamic_node_id_server::FileStorageBackend backend; 00050 ENFORCE(0 <= backend.init("/tmp/uavcan_posix/dynamic_node_id_server/storage")); 00051 00052 auto print_key = [&](const char* key) { 00053 std::cout << static_cast<IStorageBackend&>(backend).get(key).c_str() << std::endl; 00054 }; 00055 00056 print_key("foobar"); 00057 00058 static_cast<IStorageBackend&>(backend).set("foobar", "0123456789abcdef0123456789abcdef"); 00059 static_cast<IStorageBackend&>(backend).set("the_answer", "42"); 00060 00061 print_key("foobar"); 00062 print_key("the_answer"); 00063 print_key("nonexistent"); 00064 } 00065 00066 return 0; 00067 } 00068 catch (const std::exception& ex) 00069 { 00070 std::cerr << "Exception: " << ex.what() << std::endl; 00071 return 1; 00072 } 00073 }
Generated on Tue Jul 12 2022 17:17:34 by
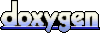