libuav original
Dependents: UAVCAN UAVCAN_Subscriber
system_clock.hpp
00001 /* 00002 * System clock driver interface. 00003 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00004 */ 00005 00006 #ifndef UAVCAN_DRIVER_SYSTEM_CLOCK_HPP_INCLUDED 00007 #define UAVCAN_DRIVER_SYSTEM_CLOCK_HPP_INCLUDED 00008 00009 #include <uavcan/std.hpp> 00010 #include <uavcan/build_config.hpp> 00011 #include <uavcan/time.hpp> 00012 00013 namespace uavcan 00014 { 00015 00016 /** 00017 * System clock interface - monotonic and UTC. 00018 */ 00019 class UAVCAN_EXPORT ISystemClock 00020 { 00021 public: 00022 virtual ~ISystemClock() { } 00023 00024 /** 00025 * Monototic system clock. 00026 * 00027 * This clock shall never jump or change rate; the base time is irrelevant. 00028 * This clock is mandatory and must remain functional at all times. 00029 * 00030 * On POSIX systems use clock_gettime() with CLOCK_MONOTONIC. 00031 */ 00032 virtual MonotonicTime getMonotonic() const = 0; 00033 00034 /** 00035 * Global network clock. 00036 * It doesn't have to be UTC, the name is a bit misleading - actual time base doesn't matter. 00037 * 00038 * This clock can be synchronized with other nodes on the bus, hence it can jump and/or change 00039 * rate occasionally. 00040 * This clock is optional; if it is not supported, return zero. Also return zero if the UTC time 00041 * is not available yet (e.g. the device has just started up with no battery clock). 00042 * 00043 * For POSIX refer to clock_gettime(), gettimeofday(). 00044 */ 00045 virtual UtcTime getUtc() const = 0; 00046 00047 /** 00048 * Adjust the network-synchronized clock. 00049 * Refer to @ref getUtc() for details. 00050 * 00051 * For POSIX refer to adjtime(), settimeofday(). 00052 * 00053 * @param [in] adjustment Amount of time to add to the clock value. 00054 */ 00055 virtual void adjustUtc(UtcDuration adjustment) = 0; 00056 }; 00057 00058 } 00059 00060 #endif // UAVCAN_DRIVER_SYSTEM_CLOCK_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:34 by
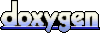