libuav original
Dependents: UAVCAN UAVCAN_Subscriber
subscriber.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_NODE_SUBSCRIBER_HPP_INCLUDED 00006 #define UAVCAN_NODE_SUBSCRIBER_HPP_INCLUDED 00007 00008 #include <cassert> 00009 #include <uavcan/build_config.hpp> 00010 #include <uavcan/node/generic_subscriber.hpp> 00011 00012 #if !defined(UAVCAN_CPP_VERSION) || !defined(UAVCAN_CPP11) 00013 # error UAVCAN_CPP_VERSION 00014 #endif 00015 00016 #if UAVCAN_CPP_VERSION >= UAVCAN_CPP11 00017 # include <functional> 00018 #endif 00019 00020 namespace uavcan 00021 { 00022 /** 00023 * Use this class to subscribe to a message. 00024 * 00025 * @tparam DataType_ Message data type. 00026 * 00027 * @tparam Callback_ Type of the callback that will be used to deliver received messages 00028 * into the application. Type of the argument of the callback can be either: 00029 * - DataType_& 00030 * - const DataType_& 00031 * - ReceivedDataStructure<DataType_>& 00032 * - const ReceivedDataStructure<DataType_>& 00033 * For the first two options, @ref ReceivedDataStructure<> will be casted implicitly. 00034 * In C++11 mode this type defaults to std::function<>. 00035 * In C++03 mode this type defaults to a plain function pointer; use binder to 00036 * call member functions as callbacks. 00037 */ 00038 template <typename DataType_, 00039 #if UAVCAN_CPP_VERSION >= UAVCAN_CPP11 00040 typename Callback_ = std::function<void (const ReceivedDataStructure<DataType_>&)> 00041 #else 00042 typename Callback_ = void (*)(const ReceivedDataStructure<DataType_>&) 00043 #endif 00044 > 00045 class UAVCAN_EXPORT Subscriber 00046 : public GenericSubscriber<DataType_, DataType_, TransferListener> 00047 { 00048 public: 00049 typedef Callback_ Callback; 00050 00051 private: 00052 typedef GenericSubscriber<DataType_, DataType_, TransferListener> BaseType; 00053 00054 Callback callback_; 00055 00056 virtual void handleReceivedDataStruct(ReceivedDataStructure<DataType_>& msg) 00057 { 00058 if (coerceOrFallback<bool>(callback_, true)) 00059 { 00060 callback_(msg); 00061 } 00062 else 00063 { 00064 handleFatalError("Sub clbk"); 00065 } 00066 } 00067 00068 public: 00069 typedef DataType_ DataType; 00070 00071 explicit Subscriber(INode& node) 00072 : BaseType(node) 00073 , callback_() 00074 { 00075 StaticAssert<DataTypeKind(DataType::DataTypeKind) == DataTypeKindMessage>::check(); 00076 } 00077 00078 /** 00079 * Begin receiving messages. 00080 * Each message will be passed to the application via the callback. 00081 * Returns negative error code. 00082 */ 00083 int start(const Callback& callback) 00084 { 00085 stop(); 00086 00087 if (!coerceOrFallback<bool>(callback, true)) 00088 { 00089 UAVCAN_TRACE("Subscriber", "Invalid callback"); 00090 return -ErrInvalidParam; 00091 } 00092 callback_ = callback; 00093 00094 return BaseType::startAsMessageListener(); 00095 } 00096 00097 using BaseType::allowAnonymousTransfers; 00098 using BaseType::stop; 00099 using BaseType::getFailureCount; 00100 }; 00101 00102 } 00103 00104 #endif // UAVCAN_NODE_SUBSCRIBER_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:34 by
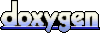