libuav original
Dependents: UAVCAN UAVCAN_Subscriber
board.cpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include "board.hpp" 00006 #include <cstring> 00007 #include <unistd.h> 00008 #include <ch.h> 00009 #include <ch.hpp> 00010 #include <hal.h> 00011 #include <zubax_chibios/sys/sys.h> 00012 00013 /** 00014 * GPIO config for ChibiOS PAL driver 00015 */ 00016 const PALConfig pal_default_config = 00017 { 00018 { VAL_GPIOAODR, VAL_GPIOACRL, VAL_GPIOACRH }, 00019 { VAL_GPIOBODR, VAL_GPIOBCRL, VAL_GPIOBCRH }, 00020 { VAL_GPIOCODR, VAL_GPIOCCRL, VAL_GPIOCCRH }, 00021 { VAL_GPIODODR, VAL_GPIODCRL, VAL_GPIODCRH }, 00022 { VAL_GPIOEODR, VAL_GPIOECRL, VAL_GPIOECRH } 00023 }; 00024 00025 namespace board 00026 { 00027 00028 void init() 00029 { 00030 halInit(); 00031 00032 chibios_rt::System::init(); 00033 00034 sdStart(&STDOUT_SD, nullptr); 00035 } 00036 00037 __attribute__((noreturn)) 00038 void die(int error) 00039 { 00040 lowsyslog("Fatal error %i\n", error); 00041 while (1) 00042 { 00043 setLed(false); 00044 ::sleep(1); 00045 setLed(true); 00046 ::sleep(1); 00047 } 00048 } 00049 00050 void setLed(bool state) 00051 { 00052 palWritePad(GPIO_PORT_LED, GPIO_PIN_LED, state); 00053 } 00054 00055 void restart() 00056 { 00057 NVIC_SystemReset(); 00058 } 00059 00060 void readUniqueID(std::uint8_t bytes[UniqueIDSize]) 00061 { 00062 std::memcpy(bytes, reinterpret_cast<const void*>(0x1FFFF7E8), UniqueIDSize); 00063 } 00064 00065 } 00066 00067 /* 00068 * Early init from ChibiOS 00069 */ 00070 extern "C" 00071 { 00072 00073 void __early_init(void) 00074 { 00075 stm32_clock_init(); 00076 } 00077 00078 void boardInit(void) 00079 { 00080 AFIO->MAPR |= 00081 AFIO_MAPR_CAN_REMAP_REMAP3 | 00082 AFIO_MAPR_CAN2_REMAP | 00083 AFIO_MAPR_USART2_REMAP; 00084 00085 /* 00086 * Enabling the CAN controllers, then configuring GPIO functions for CAN_TX. 00087 * Order matters, otherwise the CAN_TX pins will twitch, disturbing the CAN bus. 00088 * This is why we can't perform this initialization using ChibiOS GPIO configuration. 00089 */ 00090 RCC->APB1ENR |= RCC_APB1ENR_CAN1EN; 00091 palSetPadMode(GPIOD, 1, PAL_MODE_STM32_ALTERNATE_PUSHPULL); 00092 00093 #if UAVCAN_STM32_NUM_IFACES > 1 00094 RCC->APB1ENR |= RCC_APB1ENR_CAN2EN; 00095 palSetPadMode(GPIOB, 6, PAL_MODE_STM32_ALTERNATE_PUSHPULL); 00096 #endif 00097 } 00098 00099 }
Generated on Tue Jul 12 2022 17:17:30 by
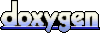