libuav original
Dependents: UAVCAN UAVCAN_Subscriber
internal.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #pragma once 00006 00007 #include <uavcan_stm32/build_config.hpp> 00008 00009 #if UAVCAN_STM32_CHIBIOS 00010 # include <hal.h> 00011 #elif UAVCAN_STM32_NUTTX 00012 # include <nuttx/arch.h> 00013 # include <arch/board/board.h> 00014 # include <chip/stm32_tim.h> 00015 # include <syslog.h> 00016 #elif UAVCAN_STM32_BAREMETAL 00017 #include <chip.h> // See http://uavcan.org/Implementations/Libuavcan/Platforms/STM32/ 00018 #elif UAVCAN_STM32_FREERTOS 00019 # include <chip.h> 00020 # include <cmsis_os.h> 00021 #else 00022 # error "Unknown OS" 00023 #endif 00024 00025 /** 00026 * Debug output 00027 */ 00028 #ifndef UAVCAN_STM32_LOG 00029 // syslog() crashes the system in this context 00030 // # if UAVCAN_STM32_NUTTX && CONFIG_ARCH_LOWPUTC 00031 # if 0 00032 # define UAVCAN_STM32_LOG(fmt, ...) syslog("uavcan_stm32: " fmt "\n", ##__VA_ARGS__) 00033 # else 00034 # define UAVCAN_STM32_LOG(...) ((void)0) 00035 # endif 00036 #endif 00037 00038 /** 00039 * IRQ handler macros 00040 */ 00041 #if UAVCAN_STM32_CHIBIOS 00042 # define UAVCAN_STM32_IRQ_HANDLER(id) CH_IRQ_HANDLER(id) 00043 # define UAVCAN_STM32_IRQ_PROLOGUE() CH_IRQ_PROLOGUE() 00044 # define UAVCAN_STM32_IRQ_EPILOGUE() CH_IRQ_EPILOGUE() 00045 #elif UAVCAN_STM32_NUTTX 00046 # define UAVCAN_STM32_IRQ_HANDLER(id) int id(int irq, FAR void* context) 00047 # define UAVCAN_STM32_IRQ_PROLOGUE() 00048 # define UAVCAN_STM32_IRQ_EPILOGUE() return 0; 00049 #else 00050 # define UAVCAN_STM32_IRQ_HANDLER(id) void id(void) 00051 # define UAVCAN_STM32_IRQ_PROLOGUE() 00052 # define UAVCAN_STM32_IRQ_EPILOGUE() 00053 #endif 00054 00055 #if UAVCAN_STM32_CHIBIOS 00056 /** 00057 * Priority mask for timer and CAN interrupts. 00058 */ 00059 # ifndef UAVCAN_STM32_IRQ_PRIORITY_MASK 00060 # if (CH_KERNEL_MAJOR == 2) 00061 # define UAVCAN_STM32_IRQ_PRIORITY_MASK CORTEX_PRIORITY_MASK(CORTEX_MAX_KERNEL_PRIORITY) 00062 # else // ChibiOS 3+ 00063 # define UAVCAN_STM32_IRQ_PRIORITY_MASK CORTEX_MAX_KERNEL_PRIORITY 00064 # endif 00065 # endif 00066 #endif 00067 00068 #if UAVCAN_STM32_BAREMETAL 00069 /** 00070 * Priority mask for timer and CAN interrupts. 00071 */ 00072 # ifndef UAVCAN_STM32_IRQ_PRIORITY_MASK 00073 # define UAVCAN_STM32_IRQ_PRIORITY_MASK 0 00074 # endif 00075 #endif 00076 00077 #if UAVCAN_STM32_FREERTOS 00078 /** 00079 * Priority mask for timer and CAN interrupts. 00080 */ 00081 # ifndef UAVCAN_STM32_IRQ_PRIORITY_MASK 00082 # define UAVCAN_STM32_IRQ_PRIORITY_MASK configLIBRARY_MAX_SYSCALL_INTERRUPT_PRIORITY 00083 # endif 00084 #endif 00085 00086 /** 00087 * Glue macros 00088 */ 00089 #define UAVCAN_STM32_GLUE2_(A, B) A##B 00090 #define UAVCAN_STM32_GLUE2(A, B) UAVCAN_STM32_GLUE2_(A, B) 00091 00092 #define UAVCAN_STM32_GLUE3_(A, B, C) A##B##C 00093 #define UAVCAN_STM32_GLUE3(A, B, C) UAVCAN_STM32_GLUE3_(A, B, C) 00094 00095 namespace uavcan_stm32 00096 { 00097 #if UAVCAN_STM32_CHIBIOS 00098 00099 struct CriticalSectionLocker 00100 { 00101 CriticalSectionLocker() { chSysSuspend(); } 00102 ~CriticalSectionLocker() { chSysEnable(); } 00103 }; 00104 00105 #elif UAVCAN_STM32_NUTTX 00106 00107 struct CriticalSectionLocker 00108 { 00109 const irqstate_t flags_; 00110 00111 CriticalSectionLocker() 00112 : flags_(enter_critical_section()) 00113 { } 00114 00115 ~CriticalSectionLocker() 00116 { 00117 leave_critical_section(flags_); 00118 } 00119 }; 00120 00121 #elif UAVCAN_STM32_BAREMETAL 00122 00123 struct CriticalSectionLocker 00124 { 00125 00126 CriticalSectionLocker() 00127 { 00128 __disable_irq(); 00129 } 00130 00131 ~CriticalSectionLocker() 00132 { 00133 __enable_irq(); 00134 } 00135 }; 00136 00137 #elif UAVCAN_STM32_FREERTOS 00138 00139 struct CriticalSectionLocker 00140 { 00141 00142 CriticalSectionLocker() 00143 { 00144 taskENTER_CRITICAL(); 00145 } 00146 00147 ~CriticalSectionLocker() 00148 { 00149 taskEXIT_CRITICAL(); 00150 } 00151 }; 00152 00153 #endif 00154 00155 namespace clock 00156 { 00157 uavcan::uint64_t getUtcUSecFromCanInterrupt(); 00158 } 00159 }
Generated on Tue Jul 12 2022 17:17:32 by
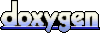