libuav original
Dependents: UAVCAN UAVCAN_Subscriber
ring_buffer.h
00001 /* 00002 * @brief Common ring buffer support functions 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2012 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __RING_BUFFER_H_ 00033 #define __RING_BUFFER_H_ 00034 00035 #include "lpc_types.h" 00036 00037 /** @defgroup Ring_Buffer CHIP: Simple ring buffer implementation 00038 * @ingroup CHIP_Common 00039 * @{ 00040 */ 00041 00042 /** 00043 * @brief Ring buffer structure 00044 */ 00045 typedef struct { 00046 void *data; 00047 int count; 00048 int itemSz; 00049 uint32_t head; 00050 uint32_t tail; 00051 } RINGBUFF_T; 00052 00053 /** 00054 * @def RB_VHEAD(rb) 00055 * volatile typecasted head index 00056 */ 00057 #define RB_VHEAD(rb) (*(volatile uint32_t *) &(rb)->head) 00058 00059 /** 00060 * @def RB_VTAIL(rb) 00061 * volatile typecasted tail index 00062 */ 00063 #define RB_VTAIL(rb) (*(volatile uint32_t *) &(rb)->tail) 00064 00065 /** 00066 * @brief Initialize ring buffer 00067 * @param RingBuff : Pointer to ring buffer to initialize 00068 * @param buffer : Pointer to buffer to associate with RingBuff 00069 * @param itemSize : Size of each buffer item size 00070 * @param count : Size of ring buffer 00071 * @note Memory pointed by @a buffer must have correct alignment of 00072 * @a itemSize, and @a count must be a power of 2 and must at 00073 * least be 2 or greater. 00074 * @return Nothing 00075 */ 00076 int RingBuffer_Init(RINGBUFF_T *RingBuff, void *buffer, int itemSize, int count); 00077 00078 /** 00079 * @brief Resets the ring buffer to empty 00080 * @param RingBuff : Pointer to ring buffer 00081 * @return Nothing 00082 */ 00083 STATIC INLINE void RingBuffer_Flush(RINGBUFF_T *RingBuff) 00084 { 00085 RingBuff->head = RingBuff->tail = 0; 00086 } 00087 00088 /** 00089 * @brief Return size the ring buffer 00090 * @param RingBuff : Pointer to ring buffer 00091 * @return Size of the ring buffer in bytes 00092 */ 00093 STATIC INLINE int RingBuffer_GetSize(RINGBUFF_T *RingBuff) 00094 { 00095 return RingBuff->count; 00096 } 00097 00098 /** 00099 * @brief Return number of items in the ring buffer 00100 * @param RingBuff : Pointer to ring buffer 00101 * @return Number of items in the ring buffer 00102 */ 00103 STATIC INLINE int RingBuffer_GetCount(RINGBUFF_T *RingBuff) 00104 { 00105 return RB_VHEAD(RingBuff) - RB_VTAIL(RingBuff); 00106 } 00107 00108 /** 00109 * @brief Return number of free items in the ring buffer 00110 * @param RingBuff : Pointer to ring buffer 00111 * @return Number of free items in the ring buffer 00112 */ 00113 STATIC INLINE int RingBuffer_GetFree(RINGBUFF_T *RingBuff) 00114 { 00115 return RingBuff->count - RingBuffer_GetCount(RingBuff); 00116 } 00117 00118 /** 00119 * @brief Return number of items in the ring buffer 00120 * @param RingBuff : Pointer to ring buffer 00121 * @return 1 if the ring buffer is full, otherwise 0 00122 */ 00123 STATIC INLINE int RingBuffer_IsFull(RINGBUFF_T *RingBuff) 00124 { 00125 return (RingBuffer_GetCount(RingBuff) >= RingBuff->count); 00126 } 00127 00128 /** 00129 * @brief Return empty status of ring buffer 00130 * @param RingBuff : Pointer to ring buffer 00131 * @return 1 if the ring buffer is empty, otherwise 0 00132 */ 00133 STATIC INLINE int RingBuffer_IsEmpty(RINGBUFF_T *RingBuff) 00134 { 00135 return RB_VHEAD(RingBuff) == RB_VTAIL(RingBuff); 00136 } 00137 00138 /** 00139 * @brief Insert a single item into ring buffer 00140 * @param RingBuff : Pointer to ring buffer 00141 * @param data : pointer to item 00142 * @return 1 when successfully inserted, 00143 * 0 on error (Buffer not initialized using 00144 * RingBuffer_Init() or attempted to insert 00145 * when buffer is full) 00146 */ 00147 int RingBuffer_Insert(RINGBUFF_T *RingBuff, const void *data); 00148 00149 /** 00150 * @brief Insert an array of items into ring buffer 00151 * @param RingBuff : Pointer to ring buffer 00152 * @param data : Pointer to first element of the item array 00153 * @param num : Number of items in the array 00154 * @return number of items successfully inserted, 00155 * 0 on error (Buffer not initialized using 00156 * RingBuffer_Init() or attempted to insert 00157 * when buffer is full) 00158 */ 00159 int RingBuffer_InsertMult(RINGBUFF_T *RingBuff, const void *data, int num); 00160 00161 /** 00162 * @brief Pop an item from the ring buffer 00163 * @param RingBuff : Pointer to ring buffer 00164 * @param data : Pointer to memory where popped item be stored 00165 * @return 1 when item popped successfuly onto @a data, 00166 * 0 When error (Buffer not initialized using 00167 * RingBuffer_Init() or attempted to pop item when 00168 * the buffer is empty) 00169 */ 00170 int RingBuffer_Pop(RINGBUFF_T *RingBuff, void *data); 00171 00172 /** 00173 * @brief Pop an array of items from the ring buffer 00174 * @param RingBuff : Pointer to ring buffer 00175 * @param data : Pointer to memory where popped items be stored 00176 * @param num : Max number of items array @a data can hold 00177 * @return Number of items popped onto @a data, 00178 * 0 on error (Buffer not initialized using RingBuffer_Init() 00179 * or attempted to pop when the buffer is empty) 00180 */ 00181 int RingBuffer_PopMult(RINGBUFF_T *RingBuff, void *data, int num); 00182 00183 00184 /** 00185 * @} 00186 */ 00187 00188 #endif /* __RING_BUFFER_H_ */
Generated on Tue Jul 12 2022 17:17:33 by
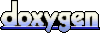