libuav original
Dependents: UAVCAN UAVCAN_Subscriber
pinint_11xx.h
00001 /* 00002 * @brief LPC11xx Pin Interrupt and Pattern Match Registers and driver 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2012 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __PININT_11XX_H_ 00033 #define __PININT_11XX_H_ 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif 00038 00039 /** @defgroup PININT_11XX CHIP: LPC11xx Pin Interrupt and Pattern Match driver 00040 * @ingroup CHIP_11XX_Drivers 00041 * For device familes identified with CHIP definitions CHIP_LPC11AXX, 00042 * CHIP_LPC11EXX, and CHIP_LPC11UXX only. 00043 * @{ 00044 */ 00045 00046 #if defined(CHIP_LPC11AXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11UXX) 00047 00048 /** 00049 * @brief LPC11xx Pin Interrupt and Pattern Match register block structure 00050 */ 00051 typedef struct { /*!< PIN_INT Structure */ 00052 __IO uint32_t ISEL ; /*!< Pin Interrupt Mode register */ 00053 __IO uint32_t IENR ; /*!< Pin Interrupt Enable (Rising) register */ 00054 __IO uint32_t SIENR ; /*!< Set Pin Interrupt Enable (Rising) register */ 00055 __IO uint32_t CIENR ; /*!< Clear Pin Interrupt Enable (Rising) register */ 00056 __IO uint32_t IENF ; /*!< Pin Interrupt Enable Falling Edge / Active Level register */ 00057 __IO uint32_t SIENF ; /*!< Set Pin Interrupt Enable Falling Edge / Active Level register */ 00058 __IO uint32_t CIENF ; /*!< Clear Pin Interrupt Enable Falling Edge / Active Level address */ 00059 __IO uint32_t RISE ; /*!< Pin Interrupt Rising Edge register */ 00060 __IO uint32_t FALL ; /*!< Pin Interrupt Falling Edge register */ 00061 __IO uint32_t IST ; /*!< Pin Interrupt Status register */ 00062 } LPC_PIN_INT_T; 00063 00064 /** 00065 * LPC11xx Pin Interrupt channel values 00066 */ 00067 #define PININTCH0 (1 << 0) 00068 #define PININTCH1 (1 << 1) 00069 #define PININTCH2 (1 << 2) 00070 #define PININTCH3 (1 << 3) 00071 #define PININTCH4 (1 << 4) 00072 #define PININTCH5 (1 << 5) 00073 #define PININTCH6 (1 << 6) 00074 #define PININTCH7 (1 << 7) 00075 #define PININTCH(ch) (1 << (ch)) 00076 00077 /** 00078 * @brief Initialize Pin interrupt block 00079 * @param pPININT : The base address of Pin interrupt block 00080 * @return Nothing 00081 * @note This function should be used after the Chip_GPIO_Init() function. 00082 */ 00083 STATIC INLINE void Chip_PININT_Init(LPC_PIN_INT_T *pPININT) {} 00084 00085 /** 00086 * @brief De-Initialize Pin interrupt block 00087 * @param pPININT : The base address of Pin interrupt block 00088 * @return Nothing 00089 */ 00090 STATIC INLINE void Chip_PININT_DeInit(LPC_PIN_INT_T *pPININT) {} 00091 00092 /** 00093 * @brief Configure the pins as edge sensitive in Pin interrupt block 00094 * @param pPININT : The base address of Pin interrupt block 00095 * @param pins : Pins (ORed value of PININTCH*) 00096 * @return Nothing 00097 */ 00098 STATIC INLINE void Chip_PININT_SetPinModeEdge(LPC_PIN_INT_T *pPININT, uint32_t pins) 00099 { 00100 pPININT->ISEL &= ~pins; 00101 } 00102 00103 /** 00104 * @brief Configure the pins as level sensitive in Pin interrupt block 00105 * @param pPININT : The base address of Pin interrupt block 00106 * @param pins : Pins (ORed value of PININTCH*) 00107 * @return Nothing 00108 */ 00109 STATIC INLINE void Chip_PININT_SetPinModeLevel(LPC_PIN_INT_T *pPININT, uint32_t pins) 00110 { 00111 pPININT->ISEL |= pins; 00112 } 00113 00114 /** 00115 * @brief Return current PININT rising edge or high level interrupt enable state 00116 * @param pPININT : The base address of Pin interrupt block 00117 * @return A bifield containing the high edge/level interrupt enables for each 00118 * interrupt. Bit 0 = PININT0, 1 = PININT1, etc. 00119 * For each bit, a 0 means the high edge/level interrupt is disabled, while a 1 00120 * means it's enabled. 00121 */ 00122 STATIC INLINE uint32_t Chip_PININT_GetHighEnabled(LPC_PIN_INT_T *pPININT) 00123 { 00124 return pPININT->IENR ; 00125 } 00126 00127 /** 00128 * @brief Enable high edge/level PININT interrupts for pins 00129 * @param pPININT : The base address of Pin interrupt block 00130 * @param pins : Pins to enable (ORed value of PININTCH*) 00131 * @return Nothing 00132 */ 00133 STATIC INLINE void Chip_PININT_EnableIntHigh(LPC_PIN_INT_T *pPININT, uint32_t pins) 00134 { 00135 pPININT->SIENR = pins; 00136 } 00137 00138 /** 00139 * @brief Disable high edge/level PININT interrupts for pins 00140 * @param pPININT : The base address of Pin interrupt block 00141 * @param pins : Pins to disable (ORed value of PININTCH*) 00142 * @return Nothing 00143 */ 00144 STATIC INLINE void Chip_PININT_DisableIntHigh(LPC_PIN_INT_T *pPININT, uint32_t pins) 00145 { 00146 pPININT->CIENR = pins; 00147 } 00148 00149 /** 00150 * @brief Return current PININT falling edge or low level interrupt enable state 00151 * @param pPININT : The base address of Pin interrupt block 00152 * @return A bifield containing the low edge/level interrupt enables for each 00153 * interrupt. Bit 0 = PININT0, 1 = PININT1, etc. 00154 * For each bit, a 0 means the low edge/level interrupt is disabled, while a 1 00155 * means it's enabled. 00156 */ 00157 STATIC INLINE uint32_t Chip_PININT_GetLowEnabled(LPC_PIN_INT_T *pPININT) 00158 { 00159 return pPININT->IENF ; 00160 } 00161 00162 /** 00163 * @brief Enable low edge/level PININT interrupts for pins 00164 * @param pPININT : The base address of Pin interrupt block 00165 * @param pins : Pins to enable (ORed value of PININTCH*) 00166 * @return Nothing 00167 */ 00168 STATIC INLINE void Chip_PININT_EnableIntLow(LPC_PIN_INT_T *pPININT, uint32_t pins) 00169 { 00170 pPININT->SIENF = pins; 00171 } 00172 00173 /** 00174 * @brief Disable low edge/level PININT interrupts for pins 00175 * @param pPININT : The base address of Pin interrupt block 00176 * @param pins : Pins to disable (ORed value of PININTCH*) 00177 * @return Nothing 00178 */ 00179 STATIC INLINE void Chip_PININT_DisableIntLow(LPC_PIN_INT_T *pPININT, uint32_t pins) 00180 { 00181 pPININT->CIENF = pins; 00182 } 00183 00184 /** 00185 * @brief Return pin states that have a detected latched high edge (RISE) state 00186 * @param pPININT : The base address of Pin interrupt block 00187 * @return PININT states (bit n = high) with a latched rise state detected 00188 */ 00189 STATIC INLINE uint32_t Chip_PININT_GetRiseStates(LPC_PIN_INT_T *pPININT) 00190 { 00191 return pPININT->RISE ; 00192 } 00193 00194 /** 00195 * @brief Clears pin states that had a latched high edge (RISE) state 00196 * @param pPININT : The base address of Pin interrupt block 00197 * @param pins : Pins with latched states to clear 00198 * @return Nothing 00199 */ 00200 STATIC INLINE void Chip_PININT_ClearRiseStates(LPC_PIN_INT_T *pPININT, uint32_t pins) 00201 { 00202 pPININT->RISE = pins; 00203 } 00204 00205 /** 00206 * @brief Return pin states that have a detected latched falling edge (FALL) state 00207 * @param pPININT : The base address of Pin interrupt block 00208 * @return PININT states (bit n = high) with a latched rise state detected 00209 */ 00210 STATIC INLINE uint32_t Chip_PININT_GetFallStates(LPC_PIN_INT_T *pPININT) 00211 { 00212 return pPININT->FALL ; 00213 } 00214 00215 /** 00216 * @brief Clears pin states that had a latched falling edge (FALL) state 00217 * @param pPININT : The base address of Pin interrupt block 00218 * @param pins : Pins with latched states to clear 00219 * @return Nothing 00220 */ 00221 STATIC INLINE void Chip_PININT_ClearFallStates(LPC_PIN_INT_T *pPININT, uint32_t pins) 00222 { 00223 pPININT->FALL = pins; 00224 } 00225 00226 /** 00227 * @brief Get interrupt status from Pin interrupt block 00228 * @param pPININT : The base address of Pin interrupt block 00229 * @return Interrupt status (bit n for PININTn = high means interrupt ie pending) 00230 */ 00231 STATIC INLINE uint32_t Chip_PININT_GetIntStatus(LPC_PIN_INT_T *pPININT) 00232 { 00233 return pPININT->IST ; 00234 } 00235 00236 /** 00237 * @brief Clear interrupt status in Pin interrupt block 00238 * @param pPININT : The base address of Pin interrupt block 00239 * @param pins : Pin interrupts to clear (ORed value of PININTCH*) 00240 * @return Nothing 00241 */ 00242 STATIC INLINE void Chip_PININT_ClearIntStatus(LPC_PIN_INT_T *pPININT, uint32_t pins) 00243 { 00244 pPININT->IST = pins; 00245 } 00246 00247 #endif /* defined(CHIP_LPC11AXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11UXX) */ 00248 00249 /** 00250 * @} 00251 */ 00252 00253 #ifdef __cplusplus 00254 } 00255 #endif 00256 00257 #endif /* __PININT_11XX_H_ */
Generated on Tue Jul 12 2022 17:17:33 by
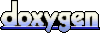