libuav original
Dependents: UAVCAN UAVCAN_Subscriber
perf_counter.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_TRANSPORT_PERF_COUNTER_HPP_INCLUDED 00006 #define UAVCAN_TRANSPORT_PERF_COUNTER_HPP_INCLUDED 00007 00008 #include <uavcan/std.hpp> 00009 #include <uavcan/build_config.hpp> 00010 #include <uavcan/util/templates.hpp> 00011 00012 namespace uavcan 00013 { 00014 00015 #if UAVCAN_TINY 00016 00017 class UAVCAN_EXPORT TransferPerfCounter : Noncopyable 00018 { 00019 public: 00020 void addTxTransfer() { } 00021 void addRxTransfer() { } 00022 void addError() { } 00023 void addErrors(unsigned) { } 00024 uint64_t getTxTransferCount() const { return 0; } 00025 uint64_t getRxTransferCount() const { return 0; } 00026 uint64_t getErrorCount() const { return 0; } 00027 }; 00028 00029 #else 00030 00031 /** 00032 * The class is declared noncopyable for two reasons: 00033 * - to prevent accidental pass-by-value into a mutator 00034 * - to make the addresses of the counters fixed and exposable to the user of the library 00035 */ 00036 class UAVCAN_EXPORT TransferPerfCounter : Noncopyable 00037 { 00038 uint64_t transfers_tx_; 00039 uint64_t transfers_rx_; 00040 uint64_t errors_; 00041 00042 public: 00043 TransferPerfCounter() 00044 : transfers_tx_(0) 00045 , transfers_rx_(0) 00046 , errors_(0) 00047 { } 00048 00049 void addTxTransfer() { transfers_tx_++; } 00050 void addRxTransfer() { transfers_rx_++; } 00051 00052 void addError() { errors_++; } 00053 00054 void addErrors(unsigned errors) 00055 { 00056 errors_ += errors; 00057 } 00058 00059 /** 00060 * Returned references are guaranteed to be valid as long as this instance of Node exists. 00061 * This is enforced by virtue of the class being Noncopyable. 00062 */ 00063 const uint64_t& getTxTransferCount() const { return transfers_tx_; } 00064 const uint64_t& getRxTransferCount() const { return transfers_rx_; } 00065 const uint64_t& getErrorCount() const { return errors_; } 00066 }; 00067 00068 #endif 00069 00070 } 00071 00072 #endif // UAVCAN_TRANSPORT_PERF_COUNTER_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:33 by
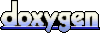