libuav original
Dependents: UAVCAN UAVCAN_Subscriber
method_binder.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_UTIL_METHOD_BINDER_HPP_INCLUDED 00006 #define UAVCAN_UTIL_METHOD_BINDER_HPP_INCLUDED 00007 00008 #include <uavcan/error.hpp> 00009 #include <uavcan/build_config.hpp> 00010 #include <uavcan/util/templates.hpp> 00011 00012 namespace uavcan 00013 { 00014 /** 00015 * Use this to call member functions as callbacks in C++03 mode. 00016 * 00017 * In C++11 or newer you don't need it because you can use std::function<>/std::bind<> instead. 00018 */ 00019 template <typename ObjectPtr, typename MemFunPtr> 00020 class UAVCAN_EXPORT MethodBinder 00021 { 00022 ObjectPtr obj_; 00023 MemFunPtr fun_; 00024 00025 void validateBeforeCall() const 00026 { 00027 if (!operator bool()) 00028 { 00029 handleFatalError("Null binder"); 00030 } 00031 } 00032 00033 public: 00034 MethodBinder() 00035 : obj_() 00036 , fun_() 00037 { } 00038 00039 MethodBinder(ObjectPtr o, MemFunPtr f) 00040 : obj_(o) 00041 , fun_(f) 00042 { } 00043 00044 /** 00045 * Returns true if the binder is initialized (doesn't contain null pointers). 00046 */ 00047 operator bool() const 00048 { 00049 return coerceOrFallback<bool>(obj_, true) && coerceOrFallback<bool>(fun_, true); 00050 } 00051 00052 /** 00053 * Will raise a fatal error if either method pointer or object pointer are null. 00054 */ 00055 void operator()() 00056 { 00057 validateBeforeCall(); 00058 (obj_->*fun_)(); 00059 } 00060 00061 /** 00062 * Will raise a fatal error if either method pointer or object pointer are null. 00063 */ 00064 template <typename Par1> 00065 void operator()(Par1& p1) 00066 { 00067 validateBeforeCall(); 00068 (obj_->*fun_)(p1); 00069 } 00070 00071 /** 00072 * Will raise a fatal error if either method pointer or object pointer are null. 00073 */ 00074 template <typename Par1, typename Par2> 00075 void operator()(Par1& p1, Par2& p2) 00076 { 00077 validateBeforeCall(); 00078 (obj_->*fun_)(p1, p2); 00079 } 00080 }; 00081 00082 } 00083 00084 #endif // UAVCAN_UTIL_METHOD_BINDER_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:33 by
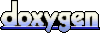