libuav original
Dependents: UAVCAN UAVCAN_Subscriber
memory_storage_backend.hpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #pragma once 00006 00007 #include <map> 00008 #include <uavcan/protocol/dynamic_node_id_server/storage_backend.hpp> 00009 00010 class MemoryStorageBackend : public uavcan::dynamic_node_id_server::IStorageBackend 00011 { 00012 typedef std::map<String, String> Container; 00013 Container container_; 00014 00015 bool fail_; 00016 00017 public: 00018 MemoryStorageBackend() 00019 : fail_(false) 00020 { } 00021 00022 virtual String get(const String& key) const 00023 { 00024 const Container::const_iterator it = container_.find(key); 00025 if (it == container_.end()) 00026 { 00027 return String(); 00028 } 00029 return it->second; 00030 } 00031 00032 virtual void set(const String& key, const String& value) 00033 { 00034 if (!fail_) 00035 { 00036 container_[key] = value; 00037 } 00038 } 00039 00040 void failOnSetCalls(bool really) { fail_ = really; } 00041 00042 void reset() { container_.clear(); } 00043 00044 unsigned getNumKeys() const { return unsigned(container_.size()); } 00045 00046 void print() const 00047 { 00048 for (Container::const_iterator it = container_.begin(); it != container_.end(); ++it) 00049 { 00050 std::cout << it->first.c_str() << "\t" << it->second.c_str() << std::endl; 00051 } 00052 } 00053 };
Generated on Tue Jul 12 2022 17:17:33 by
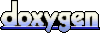