libuav original
Dependents: UAVCAN UAVCAN_Subscriber
libstubs.cpp
00001 /* 00002 * Pavel Kirienko, 2014 <pavel.kirienko@gmail.com> 00003 * Standard library stubs 00004 */ 00005 00006 #include <cstdlib> 00007 #include <unistd.h> 00008 #include <sys/types.h> 00009 00010 #if __GNUC__ 00011 # pragma GCC diagnostic ignored "-Wmissing-declarations" 00012 #endif 00013 00014 void* __dso_handle; 00015 00016 void* operator new(std::size_t) 00017 { 00018 std::abort(); 00019 return reinterpret_cast<void*>(0xFFFFFFFF); 00020 } 00021 00022 void* operator new[](std::size_t) 00023 { 00024 std::abort(); 00025 return reinterpret_cast<void*>(0xFFFFFFFF); 00026 } 00027 00028 void operator delete(void*) 00029 { 00030 std::abort(); 00031 } 00032 00033 void operator delete[](void*) 00034 { 00035 std::abort(); 00036 } 00037 00038 namespace __gnu_cxx 00039 { 00040 00041 void __verbose_terminate_handler() 00042 { 00043 std::abort(); 00044 } 00045 00046 } 00047 00048 /* 00049 * libstdc++ stubs 00050 */ 00051 extern "C" 00052 { 00053 00054 int __aeabi_atexit(void*, void(*)(void*), void*) 00055 { 00056 return 0; 00057 } 00058 00059 __extension__ typedef int __guard __attribute__((mode (__DI__))); 00060 00061 void __cxa_atexit(void(*)(void *), void*, void*) 00062 { 00063 } 00064 00065 int __cxa_guard_acquire(__guard* g) 00066 { 00067 return !*g; 00068 } 00069 00070 void __cxa_guard_release (__guard* g) 00071 { 00072 *g = 1; 00073 } 00074 00075 void __cxa_guard_abort (__guard*) 00076 { 00077 } 00078 00079 void __cxa_pure_virtual() 00080 { 00081 std::abort(); 00082 } 00083 00084 } 00085 00086 /* 00087 * stdio 00088 */ 00089 extern "C" 00090 { 00091 00092 __attribute__((used)) 00093 void abort() 00094 { 00095 while (true) { } 00096 } 00097 00098 int _read_r(struct _reent*, int, char*, int) 00099 { 00100 return -1; 00101 } 00102 00103 int _lseek_r(struct _reent*, int, int, int) 00104 { 00105 return -1; 00106 } 00107 00108 int _write_r(struct _reent*, int, char*, int) 00109 { 00110 return -1; 00111 } 00112 00113 int _close_r(struct _reent*, int) 00114 { 00115 return -1; 00116 } 00117 00118 __attribute__((used)) 00119 caddr_t _sbrk_r(struct _reent*, int) 00120 { 00121 return 0; 00122 } 00123 00124 int _fstat_r(struct _reent*, int, struct stat*) 00125 { 00126 return -1; 00127 } 00128 00129 int _isatty_r(struct _reent*, int) 00130 { 00131 return -1; 00132 } 00133 00134 void _exit(int) 00135 { 00136 abort(); 00137 } 00138 00139 pid_t _getpid(void) 00140 { 00141 return 1; 00142 } 00143 00144 void _kill(pid_t) 00145 { 00146 } 00147 00148 }
Generated on Tue Jul 12 2022 17:17:32 by
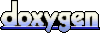