libuav original
Dependents: UAVCAN UAVCAN_Subscriber
lazy_constructor.cpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include <gtest/gtest.h> 00006 #include <uavcan/util/lazy_constructor.hpp> 00007 00008 00009 TEST(LazyConstructor, Basic) 00010 { 00011 using ::uavcan::LazyConstructor; 00012 00013 LazyConstructor<std::string> a; 00014 LazyConstructor<std::string> b; 00015 00016 ASSERT_FALSE(a); 00017 ASSERT_FALSE(b.isConstructed()); 00018 00019 /* 00020 * Construction 00021 */ 00022 a.destroy(); // no-op 00023 a.construct(); 00024 b.construct<const char*>("Hello world"); 00025 00026 ASSERT_TRUE(a); 00027 ASSERT_TRUE(b.isConstructed()); 00028 00029 ASSERT_NE(*a, *b); 00030 ASSERT_STRNE(a->c_str(), b->c_str()); 00031 00032 ASSERT_EQ(*a, ""); 00033 ASSERT_EQ(*b, "Hello world"); 00034 00035 /* 00036 * Copying 00037 */ 00038 a = b; // Assignment operator performs destruction and immediate copy construction 00039 ASSERT_EQ(*a, *b); 00040 ASSERT_EQ(*a, "Hello world"); 00041 00042 LazyConstructor<std::string> c(a); // Copy constructor call is forwarded to std::string 00043 00044 ASSERT_EQ(*c, *a); 00045 00046 *a = "123"; 00047 ASSERT_NE(*c, *a); 00048 ASSERT_EQ(*c, *b); 00049 00050 *c = "456"; 00051 ASSERT_NE(*a, *c); 00052 ASSERT_NE(*b, *a); 00053 ASSERT_NE(*c, *b); 00054 00055 /* 00056 * Destruction 00057 */ 00058 ASSERT_TRUE(c); 00059 c.destroy(); 00060 ASSERT_FALSE(c); 00061 }
Generated on Tue Jul 12 2022 17:17:32 by
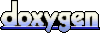