libuav original
Dependents: UAVCAN UAVCAN_Subscriber
iocon_11xx.h
00001 /* 00002 * @brief IOCON registers and control functions 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2012 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __IOCON_11XX_H_ 00033 #define __IOCON_11XX_H_ 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif 00038 00039 /** @defgroup IOCON_11XX CHIP: LPC11xx IO Control driver 00040 * @ingroup CHIP_11XX_Drivers 00041 * @{ 00042 */ 00043 00044 /** 00045 * @brief IO Configuration Unit register block structure 00046 */ 00047 #if defined(CHIP_LPC11UXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11AXX) 00048 typedef struct { /*!< LPC11AXX/LPC11UXX/LPC11EXX IOCON Structure */ 00049 __IO uint32_t PIO0[24]; 00050 __IO uint32_t PIO1[32]; 00051 } LPC_IOCON_T; 00052 00053 #else 00054 /** 00055 * @brief LPC11XX I/O Configuration register offset 00056 */ 00057 typedef enum CHIP_IOCON_PIO { 00058 IOCON_PIO0_0 = (0x00C >> 2), 00059 IOCON_PIO0_1 = (0x010 >> 2), 00060 IOCON_PIO0_2 = (0x01C >> 2), 00061 IOCON_PIO0_3 = (0x02C >> 2), 00062 IOCON_PIO0_4 = (0x030 >> 2), 00063 IOCON_PIO0_5 = (0x034 >> 2), 00064 IOCON_PIO0_6 = (0x04C >> 2), 00065 IOCON_PIO0_7 = (0x050 >> 2), 00066 IOCON_PIO0_8 = (0x060 >> 2), 00067 IOCON_PIO0_9 = (0x064 >> 2), 00068 IOCON_PIO0_10 = (0x070 >> 2), 00069 IOCON_PIO0_11 = (0x074 >> 2), 00070 00071 IOCON_PIO1_0 = (0x078 >> 2), 00072 IOCON_PIO1_1 = (0x07C >> 2), 00073 IOCON_PIO1_2 = (0x080 >> 2), 00074 IOCON_PIO1_3 = (0x090 >> 2), 00075 IOCON_PIO1_4 = (0x094 >> 2), 00076 IOCON_PIO1_5 = (0x0A0 >> 2), 00077 IOCON_PIO1_6 = (0x0A4 >> 2), 00078 IOCON_PIO1_7 = (0x0A8 >> 2), 00079 IOCON_PIO1_8 = (0x014 >> 2), 00080 IOCON_PIO1_9 = (0x038 >> 2), 00081 IOCON_PIO1_10 = (0x06C >> 2), 00082 IOCON_PIO1_11 = (0x098 >> 2), 00083 00084 IOCON_PIO2_0 = (0x008 >> 2), 00085 IOCON_PIO2_1 = (0x028 >> 2), 00086 IOCON_PIO2_2 = (0x05C >> 2), 00087 IOCON_PIO2_3 = (0x08C >> 2), 00088 IOCON_PIO2_4 = (0x040 >> 2), 00089 IOCON_PIO2_5 = (0x044 >> 2), 00090 IOCON_PIO2_6 = (0x000 >> 2), 00091 IOCON_PIO2_7 = (0x020 >> 2), 00092 IOCON_PIO2_8 = (0x024 >> 2), 00093 IOCON_PIO2_9 = (0x054 >> 2), 00094 IOCON_PIO2_10 = (0x058 >> 2), 00095 #if !defined(CHIP_LPC1125) 00096 IOCON_PIO2_11 = (0x070 >> 2), 00097 #endif 00098 00099 IOCON_PIO3_0 = (0x084 >> 2), 00100 #if !defined(CHIP_LPC1125) 00101 IOCON_PIO3_1 = (0x088 >> 2), 00102 #endif 00103 IOCON_PIO3_2 = (0x09C >> 2), 00104 IOCON_PIO3_3 = (0x0AC >> 2), 00105 IOCON_PIO3_4 = (0x03C >> 2), 00106 IOCON_PIO3_5 = (0x048 >> 2), 00107 } CHIP_IOCON_PIO_T; 00108 00109 /** 00110 * @brief LPC11XX Pin location select 00111 */ 00112 typedef enum CHIP_IOCON_PIN_LOC { 00113 IOCON_SCKLOC_PIO0_10 = (0xB0), /*!< Selects SCK0 function in pin location PIO0_10 */ 00114 #if !defined(CHIP_LPC1125) 00115 IOCON_SCKLOC_PIO2_11 = (0xB0 | 1), /*!< Selects SCK0 function in pin location PIO2_11 */ 00116 #endif 00117 IOCON_SCKLOC_PIO0_6 = (0xB0 | 2), /*!< Selects SCK0 function in pin location PIO0_6 */ 00118 00119 IOCON_DSRLOC_PIO2_1 = (0xB4), /*!< Selects DSR function in pin location PIO2_1 */ 00120 #if !defined(CHIP_LPC1125) 00121 IOCON_DSRLOC_PIO3_1 = (0xB4 | 1), /*!< Selects DSR function in pin location PIO3_1 */ 00122 #endif 00123 00124 IOCON_DCDLOC_PIO2_2 = (0xB8), /*!< Selects DCD function in pin location PIO2_2 */ 00125 IOCON_DCDLOC_PIO3_2 = (0xB8 | 1), /*!< Selects DCD function in pin location PIO3_2 */ 00126 00127 IOCON_RILOC_PIO2_3 = (0xBC), /*!< Selects RI function in pin location PIO2_3 */ 00128 IOCON_RILOC_PIO3_3 = (0xBC | 1), /*!< Selects Ri function in pin location PIO3_3 */ 00129 00130 #if defined(CHIP_LPC1125) 00131 IOCON_SSEL1_LOC_PIO2_2 = (0x18), /*!< Selects SSEL1 function in pin location PIO2_2 */ 00132 IOCON_SSEL1_LOC_PIO2_4 = (0x18 | 1), /*!< Selects SSEL1 function in pin location PIO2_4 */ 00133 00134 IOCON_CT16B0_CAP0_LOC_PIO0_2 = (0xC0), /*!< Selects SSEL1 CTB16B0_CAP0 function in pin location PIO0_2 */ 00135 IOCON_CT16B0_CAP0_LOC_PIO3_3 = (0xC0 | 1), /*!< Selects SSEL1 CTB16B0_CAP0 function in pin location PIO3_3 */ 00136 00137 IOCON_SCK1_LOC_PIO2_1 = (0xC4), /*!< Selects SCK1 function in pin location PIO2_1 */ 00138 IOCON_SCK1_LOC_PIO3_2 = (0xC4 | 1), /*!< Selects SCK1 function in pin location PIO3_2 */ 00139 00140 IOCON_MISO1_LOC_PIO2_2 = (0xC8), /*!< Selects MISO1 function in pin location PIO2_2 */ 00141 IOCON_MISO1_LOC_PIO1_10 = (0xC8 | 1), /*!< Selects MISO1 function in pin location PIO1_10 */ 00142 00143 IOCON_MOSI1_LOC_PIO2_3 = (0xCC), /*!< Selects MOSI1 function in pin location PIO2_3 */ 00144 IOCON_MOSI1_LOC_PIO1_9 = (0xCC), /*!< Selects MOSI1 function in pin location PIO1_9 */ 00145 00146 IOCON_CT326B0_CAP0_LOC_PIO1_5 = (0xD0), /*!< Selects CT32B0_CAP0 function in pin location PIO1_5 */ 00147 IOCON_CT326B0_CAP0_LOC_PIO2_9 = (0xD0 | 1), /*!< Selects CT32B0_CAP0 function in pin location PIO2_9 */ 00148 00149 IOCON_U0_RXD_LOC_PIO1_6 = (0xD4), /*!< Selects U0 RXD function in pin location PIO1_6 */ 00150 IOCON_U0_RXD_LOC_PIO2_7 = (0xD4 | 1), /*!< Selects U0 RXD function in pin location PIO2_7 */ 00151 IOCON_U0_RXD_LOC_PIO3_4 = (0xD4 | 3), /*!< Selects U0 RXD function in pin location PIO3_4 */ 00152 #endif 00153 00154 } CHIP_IOCON_PIN_LOC_T; 00155 00156 typedef struct { /*!< LPC11XX/LPC11XXLV/LPC11UXX IOCON Structure */ 00157 __IO uint32_t REG[48]; 00158 } LPC_IOCON_T; 00159 #endif 00160 00161 /** 00162 * IOCON function and mode selection definitions 00163 * See the User Manual for specific modes and functions supported by the 00164 * various LPC11xx devices. Functionality can vary per device. 00165 */ 00166 #define IOCON_FUNC0 0x0 /*!< Selects pin function 0 */ 00167 #define IOCON_FUNC1 0x1 /*!< Selects pin function 1 */ 00168 #define IOCON_FUNC2 0x2 /*!< Selects pin function 2 */ 00169 #define IOCON_FUNC3 0x3 /*!< Selects pin function 3 */ 00170 #define IOCON_FUNC4 0x4 /*!< Selects pin function 4 */ 00171 #define IOCON_FUNC5 0x5 /*!< Selects pin function 5 */ 00172 #define IOCON_FUNC6 0x6 /*!< Selects pin function 6 */ 00173 #define IOCON_FUNC7 0x7 /*!< Selects pin function 7 */ 00174 #define IOCON_MODE_INACT (0x0 << 3) /*!< No addition pin function */ 00175 #define IOCON_MODE_PULLDOWN (0x1 << 3) /*!< Selects pull-down function */ 00176 #define IOCON_MODE_PULLUP (0x2 << 3) /*!< Selects pull-up function */ 00177 #define IOCON_MODE_REPEATER (0x3 << 3) /*!< Selects pin repeater function */ 00178 #define IOCON_HYS_EN (0x1 << 5) /*!< Enables hysteresis */ 00179 #define IOCON_INV_EN (0x1 << 6) /*!< Enables invert function on input */ 00180 #define IOCON_ADMODE_EN (0x0 << 7) /*!< Enables analog input function (analog pins only) */ 00181 #define IOCON_DIGMODE_EN (0x1 << 7) /*!< Enables digital function (analog pins only) */ 00182 #define IOCON_SFI2C_EN (0x0 << 8) /*!< I2C standard mode/fast-mode */ 00183 #define IOCON_STDI2C_EN (0x1 << 8) /*!< I2C standard I/O functionality */ 00184 #define IOCON_FASTI2C_EN (0x2 << 8) /*!< I2C Fast-mode Plus */ 00185 #define IOCON_FILT_DIS (0x1 << 8) /*!< Disables noise pulses filtering (10nS glitch filter) */ 00186 #define IOCON_OPENDRAIN_EN (0x1 << 10) /*!< Enables open-drain function */ 00187 00188 /** 00189 * IOCON function and mode selection definitions (old) 00190 * For backwards compatibility. 00191 */ 00192 #define MD_PLN (0x0 << 3) /*!< Disable pull-down and pull-up resistor at resistor at pad */ 00193 #define MD_PDN (0x1 << 3) /*!< Enable pull-down resistor at pad */ 00194 #define MD_PUP (0x2 << 3) /*!< Enable pull-up resistor at pad */ 00195 #define MD_BUK (0x3 << 3) /*!< Enable pull-down and pull-up resistor at resistor at pad (repeater mode) */ 00196 #define MD_HYS (0x1 << 5) /*!< Enable hysteresis */ 00197 #define MD_INV (0x1 << 6) /*!< Invert enable */ 00198 #define MD_ADMODE (0x0 << 7) /*!< Select analog mode */ 00199 #define MD_DIGMODE (0x1 << 7) /*!< Select digitial mode */ 00200 #define MD_DISFIL (0x0 << 8) /*!< Disable 10nS input glitch filter */ 00201 #define MD_ENFIL (0x1 << 8) /*!< Enable 10nS input glitch filter */ 00202 #define MD_SFI2C (0x0 << 8) /*!< I2C standard mode/fast-mode */ 00203 #define MD_STDI2C (0x1 << 8) /*!< I2C standard I/O functionality */ 00204 #define MD_FASTI2C (0x2 << 8) /*!< I2C Fast-mode Plus */ 00205 #define MD_OPENDRAIN (0x1 << 10) /*!< Open drain mode bit */ 00206 #define FUNC0 0x0 00207 #define FUNC1 0x1 00208 #define FUNC2 0x2 00209 #define FUNC3 0x3 00210 #define FUNC4 0x4 00211 #define FUNC5 0x5 00212 #define FUNC6 0x6 00213 #define FUNC7 0x7 00214 00215 #if defined(CHIP_LPC11UXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11AXX) 00216 /** 00217 * @brief Sets I/O Control pin mux 00218 * @param pIOCON : The base of IOCON peripheral on the chip 00219 * @param port : GPIO port to mux 00220 * @param pin : GPIO pin to mux 00221 * @param modefunc : OR'ed values or type IOCON_* 00222 * @return Nothing 00223 */ 00224 void Chip_IOCON_PinMuxSet(LPC_IOCON_T *pIOCON, uint8_t port, uint8_t pin, uint32_t modefunc); 00225 00226 /** 00227 * @brief I/O Control pin mux 00228 * @param pIOCON : The base of IOCON peripheral on the chip 00229 * @param port : GPIO port to mux 00230 * @param pin : GPIO pin to mux 00231 * @param mode : OR'ed values or type IOCON_* 00232 * @param func : Pin function, value of type IOCON_FUNC? 00233 * @return Nothing 00234 */ 00235 STATIC INLINE void Chip_IOCON_PinMux(LPC_IOCON_T *pIOCON, uint8_t port, uint8_t pin, uint16_t mode, uint8_t func) 00236 { 00237 Chip_IOCON_PinMuxSet(pIOCON, port, pin, (uint32_t) (mode | func)); 00238 } 00239 00240 #else 00241 00242 /** 00243 * @brief Sets I/O Control pin mux 00244 * @param pIOCON : The base of IOCON peripheral on the chip 00245 * @param pin : GPIO pin to mux 00246 * @param modefunc : OR'ed values or type IOCON_* 00247 * @return Nothing 00248 */ 00249 STATIC INLINE void Chip_IOCON_PinMuxSet(LPC_IOCON_T *pIOCON, CHIP_IOCON_PIO_T pin, uint32_t modefunc) 00250 { 00251 pIOCON->REG [pin] = modefunc; 00252 } 00253 00254 /** 00255 * @brief I/O Control pin mux 00256 * @param pIOCON : The base of IOCON peripheral on the chip 00257 * @param pin : GPIO pin to mux 00258 * @param mode : OR'ed values or type IOCON_* 00259 * @param func : Pin function, value of type IOCON_FUNC? 00260 * @return Nothing 00261 */ 00262 STATIC INLINE void Chip_IOCON_PinMux(LPC_IOCON_T *pIOCON, CHIP_IOCON_PIO_T pin, uint16_t mode, uint8_t func) 00263 { 00264 Chip_IOCON_PinMuxSet(pIOCON, pin, (uint32_t) (mode | func)); 00265 } 00266 00267 /** 00268 * @brief Select pin location 00269 * @param pIOCON : The base of IOCON peripheral on the chip 00270 * @param sel : location selection 00271 * @return Nothing 00272 */ 00273 STATIC INLINE void Chip_IOCON_PinLocSel(LPC_IOCON_T *pIOCON, CHIP_IOCON_PIN_LOC_T sel) 00274 { 00275 pIOCON->REG [sel >> 2] = sel & 0x03; 00276 } 00277 00278 #endif /* defined(CHIP_LPC11UXX) || defined (CHIP_LPC11EXX) || defined (CHIP_LPC11AXX) */ 00279 00280 /** 00281 * @} 00282 */ 00283 00284 #ifdef __cplusplus 00285 } 00286 #endif 00287 00288 #endif /* __IOCON_11XX_H_ */
Generated on Tue Jul 12 2022 17:17:32 by
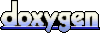