libuav original
Dependents: UAVCAN UAVCAN_Subscriber
gpio_11xx_2.h
00001 /* 00002 * @brief LPC11xx GPIO driver for CHIP_LPC11CXX, CHIP_LPC110X, CHIP_LPC11XXLV, 00003 * and CHIP_LPC1125 families only. 00004 * 00005 * @note 00006 * Copyright(C) NXP Semiconductors, 2013 00007 * All rights reserved. 00008 * 00009 * @par 00010 * Software that is described herein is for illustrative purposes only 00011 * which provides customers with programming information regarding the 00012 * LPC products. This software is supplied "AS IS" without any warranties of 00013 * any kind, and NXP Semiconductors and its licensor disclaim any and 00014 * all warranties, express or implied, including all implied warranties of 00015 * merchantability, fitness for a particular purpose and non-infringement of 00016 * intellectual property rights. NXP Semiconductors assumes no responsibility 00017 * or liability for the use of the software, conveys no license or rights under any 00018 * patent, copyright, mask work right, or any other intellectual property rights in 00019 * or to any products. NXP Semiconductors reserves the right to make changes 00020 * in the software without notification. NXP Semiconductors also makes no 00021 * representation or warranty that such application will be suitable for the 00022 * specified use without further testing or modification. 00023 * 00024 * @par 00025 * Permission to use, copy, modify, and distribute this software and its 00026 * documentation is hereby granted, under NXP Semiconductors' and its 00027 * licensor's relevant copyrights in the software, without fee, provided that it 00028 * is used in conjunction with NXP Semiconductors microcontrollers. This 00029 * copyright, permission, and disclaimer notice must appear in all copies of 00030 * this code. 00031 */ 00032 00033 #ifndef __GPIO_11XX_2_H_ 00034 #define __GPIO_11XX_2_H_ 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** @defgroup GPIO_11XX_2 CHIP: LPC11xx GPIO driver for CHIP_LPC11CXX, CHIP_LPC110X, and CHIP_LPC11XXLV families 00041 * @ingroup CHIP_11XX_Drivers 00042 * For device familes identified with CHIP definitions CHIP_LPC11CXX, 00043 * CHIP_LPC110X, and CHIP_LPC11XXLV only. 00044 * @{ 00045 */ 00046 00047 #if defined(CHIP_LPC11CXX) || defined(CHIP_LPC110X) || defined(CHIP_LPC11XXLV) || defined(CHIP_LPC1125) 00048 00049 /** 00050 * @brief GPIO port register block structure 00051 */ 00052 typedef struct { /*!< GPIO_PORT Structure */ 00053 __IO uint32_t DATA[4096]; /*!< Offset: 0x0000 to 0x3FFC Data address masking register (R/W) */ 00054 uint32_t RESERVED1[4096]; 00055 __IO uint32_t DIR ; /*!< Offset: 0x8000 Data direction register (R/W) */ 00056 __IO uint32_t IS ; /*!< Offset: 0x8004 Interrupt sense register (R/W) */ 00057 __IO uint32_t IBE ; /*!< Offset: 0x8008 Interrupt both edges register (R/W) */ 00058 __IO uint32_t IEV ; /*!< Offset: 0x800C Interrupt event register (R/W) */ 00059 __IO uint32_t IE ; /*!< Offset: 0x8010 Interrupt mask register (R/W) */ 00060 __I uint32_t RIS ; /*!< Offset: 0x8014 Raw interrupt status register (R/ ) */ 00061 __I uint32_t MIS ; /*!< Offset: 0x8018 Masked interrupt status register (R/ ) */ 00062 __O uint32_t IC ; /*!< Offset: 0x801C Interrupt clear register (W) */ 00063 uint32_t RESERVED2[8184]; /* Padding added for aligning contiguous GPIO blocks */ 00064 } LPC_GPIO_T; 00065 00066 /** 00067 * @brief Initialize GPIO block 00068 * @param pGPIO : The base of GPIO peripheral on the chip 00069 * @return Nothing 00070 */ 00071 void Chip_GPIO_Init(LPC_GPIO_T *pGPIO); 00072 00073 /** 00074 * @brief De-Initialize GPIO block 00075 * @param pGPIO : The base of GPIO peripheral on the chip 00076 * @return Nothing 00077 */ 00078 void Chip_GPIO_DeInit(LPC_GPIO_T *pGPIO); 00079 00080 /** 00081 * @brief Set a GPIO port/bit state 00082 * @param pGPIO : The base of GPIO peripheral on the chip 00083 * @param port : GPIO port to set 00084 * @param bit : GPIO bit to set 00085 * @param setting : true for high, false for low 00086 * @return Nothing 00087 */ 00088 STATIC INLINE void Chip_GPIO_WritePortBit(LPC_GPIO_T *pGPIO, uint32_t port, uint8_t bit, bool setting) 00089 { 00090 pGPIO[port].DATA [1 << bit] = setting << bit; 00091 } 00092 00093 /** 00094 * @brief Set a GPIO pin state via the GPIO byte register 00095 * @param pGPIO : The base of GPIO peripheral on the chip 00096 * @param port : Port number 00097 * @param pin : GPIO pin to set 00098 * @param setting : true for high, false for low 00099 * @return Nothing 00100 * @note This function replaces Chip_GPIO_WritePortBit() 00101 */ 00102 STATIC INLINE void Chip_GPIO_SetPinState(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin, bool setting) 00103 { 00104 pGPIO[port].DATA [1 << pin] = setting << pin; 00105 } 00106 00107 /** 00108 * @brief Read a GPIO state 00109 * @param pGPIO : The base of GPIO peripheral on the chip 00110 * @param port : GPIO port to read 00111 * @param bit : GPIO bit to read 00112 * @return true of the GPIO is high, false if low 00113 * @note It is recommended to use the Chip_GPIO_GetPinState() function instead. 00114 */ 00115 STATIC INLINE bool Chip_GPIO_ReadPortBit(LPC_GPIO_T *pGPIO, uint32_t port, uint8_t bit) 00116 { 00117 return (bool) ((pGPIO[port].DATA [1 << bit] >> bit) & 1); 00118 } 00119 00120 /** 00121 * @brief Get a GPIO pin state via the GPIO byte register 00122 * @param pGPIO : The base of GPIO peripheral on the chip 00123 * @param port : Port number 00124 * @param pin : GPIO pin to get state for 00125 * @return true if the GPIO is high, false if low 00126 * @note This function replaces Chip_GPIO_ReadPortBit() 00127 */ 00128 STATIC INLINE bool Chip_GPIO_GetPinState(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin) 00129 { 00130 return (pGPIO[port].DATA[1 << pin]) != 0; 00131 } 00132 00133 /** 00134 * @brief Seta GPIO direction 00135 * @param pGPIO : The base of GPIO peripheral on the chip 00136 * @param port : GPIO port to set 00137 * @param bit : GPIO bit to set 00138 * @param setting : true for output, false for input 00139 * @return Nothing 00140 * @note It is recommended to use the Chip_GPIO_SetPinDIROutput(), 00141 * Chip_GPIO_SetPinDIRInput() or Chip_GPIO_SetPinDIR() functions instead 00142 * of this function. 00143 */ 00144 void Chip_GPIO_WriteDirBit(LPC_GPIO_T *pGPIO, uint32_t port, uint8_t bit, bool setting); 00145 00146 /** 00147 * @brief Set GPIO direction for a single GPIO pin to an output 00148 * @param pGPIO : The base of GPIO peripheral on the chip 00149 * @param port : Port number 00150 * @param pin : GPIO pin to set direction on as output 00151 * @return Nothing 00152 */ 00153 STATIC INLINE void Chip_GPIO_SetPinDIROutput(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin) 00154 { 00155 pGPIO[port].DIR |= (1UL << pin); 00156 } 00157 00158 /** 00159 * @brief Set GPIO direction for a single GPIO pin to an input 00160 * @param pGPIO : The base of GPIO peripheral on the chip 00161 * @param port : Port number 00162 * @param pin : GPIO pin to set direction on as input 00163 * @return Nothing 00164 */ 00165 STATIC INLINE void Chip_GPIO_SetPinDIRInput(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin) 00166 { 00167 pGPIO[port].DIR &= ~(1UL << pin); 00168 } 00169 00170 /** 00171 * @brief Set GPIO direction for a single GPIO pin 00172 * @param pGPIO : The base of GPIO peripheral on the chip 00173 * @param port : Port number 00174 * @param pin : GPIO pin to set direction for 00175 * @param output : true for output, false for input 00176 * @return Nothing 00177 */ 00178 void Chip_GPIO_SetPinDIR(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin, bool output); 00179 00180 /** 00181 * @brief Read a GPIO direction (out or in) 00182 * @param pGPIO : The base of GPIO peripheral on the chip 00183 * @param port : GPIO port to read 00184 * @param bit : GPIO bit to read 00185 * @return true of the GPIO is an output, false if input 00186 * @note It is recommended to use the Chip_GPIO_GetPinDIR() function instead. 00187 */ 00188 STATIC INLINE bool Chip_GPIO_ReadDirBit(LPC_GPIO_T *pGPIO, uint32_t port, uint8_t bit) 00189 { 00190 return (bool) (((pGPIO[port].DIR ) >> bit) & 1); 00191 } 00192 00193 /** 00194 * @brief Get GPIO direction for a single GPIO pin 00195 * @param pGPIO : The base of GPIO peripheral on the chip 00196 * @param port : Port number 00197 * @param pin : GPIO pin to get direction for 00198 * @return true if the GPIO is an output, false if input 00199 */ 00200 STATIC INLINE bool Chip_GPIO_GetPinDIR(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin) 00201 { 00202 return (bool) (pGPIO[port].DIR >> pin) & 1; 00203 } 00204 00205 /** 00206 * @brief Set Direction for a GPIO port 00207 * @param pGPIO : The base of GPIO peripheral on the chip 00208 * @param port : Port Number 00209 * @param bit : GPIO bit to set 00210 * @param out : Direction value, 0 = input, !0 = output 00211 * @return None 00212 * @note Bits set to '0' are not altered. It is recommended to use the 00213 * Chip_GPIO_SetPortDIR() function instead. 00214 */ 00215 void Chip_GPIO_SetDir(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t bit, uint8_t out); 00216 00217 /** 00218 * @brief Set GPIO direction for a all selected GPIO pins to an output 00219 * @param pGPIO : The base of GPIO peripheral on the chip 00220 * @param port : Port number 00221 * @param pinMask : GPIO pin mask to set direction on as output (bits 0..n for pins 0..n) 00222 * @return Nothing 00223 * @note Sets multiple GPIO pins to the output direction, each bit's position that is 00224 * high sets the corresponding pin number for that bit to an output. 00225 */ 00226 STATIC INLINE void Chip_GPIO_SetPortDIROutput(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinMask) 00227 { 00228 pGPIO[port].DIR |= pinMask; 00229 } 00230 00231 /** 00232 * @brief Set GPIO direction for a all selected GPIO pins to an input 00233 * @param pGPIO : The base of GPIO peripheral on the chip 00234 * @param port : Port number 00235 * @param pinMask : GPIO pin mask to set direction on as input (bits 0..b for pins 0..n) 00236 * @return Nothing 00237 * @note Sets multiple GPIO pins to the input direction, each bit's position that is 00238 * high sets the corresponding pin number for that bit to an input. 00239 */ 00240 STATIC INLINE void Chip_GPIO_SetPortDIRInput(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinMask) 00241 { 00242 pGPIO[port].DIR &= ~pinMask; 00243 } 00244 00245 /** 00246 * @brief Set GPIO direction for a all selected GPIO pins to an input or output 00247 * @param pGPIO : The base of GPIO peripheral on the chip 00248 * @param port : Port number 00249 * @param pinMask : GPIO pin mask to set direction on (bits 0..b for pins 0..n) 00250 * @param outSet : Direction value, false = set as inputs, true = set as outputs 00251 * @return Nothing 00252 * @note Sets multiple GPIO pins to the input direction, each bit's position that is 00253 * high sets the corresponding pin number for that bit to an input. 00254 */ 00255 void Chip_GPIO_SetPortDIR(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinMask, bool outSet); 00256 00257 /** 00258 * @brief Get GPIO direction for a all GPIO pins 00259 * @param pGPIO : The base of GPIO peripheral on the chip 00260 * @param port : Port number 00261 * @return a bitfield containing the input and output states for each pin 00262 * @note For pins 0..n, a high state in a bit corresponds to an output state for the 00263 * same pin, while a low state corresponds to an input state. 00264 */ 00265 STATIC INLINE uint32_t Chip_GPIO_GetPortDIR(LPC_GPIO_T *pGPIO, uint8_t port) 00266 { 00267 return pGPIO[port].DIR ; 00268 } 00269 00270 /** 00271 * @brief Set all GPIO raw pin states (regardless of masking) 00272 * @param pGPIO : The base of GPIO peripheral on the chip 00273 * @param port : Port number 00274 * @param value : Value to set all GPIO pin states (0..n) to 00275 * @return Nothing 00276 */ 00277 STATIC INLINE void Chip_GPIO_SetPortValue(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t value) 00278 { 00279 pGPIO[port].DATA [0xFFF] = value; 00280 } 00281 00282 /** 00283 * @brief Get all GPIO raw pin states (regardless of masking) 00284 * @param pGPIO : The base of GPIO peripheral on the chip 00285 * @param port : Port number 00286 * @return Current (raw) state of all GPIO pins 00287 */ 00288 STATIC INLINE uint32_t Chip_GPIO_GetPortValue(LPC_GPIO_T *pGPIO, uint8_t port) 00289 { 00290 return pGPIO[port].DATA [0xFFF]; 00291 } 00292 00293 /** 00294 * @brief Set a GPIO port/bit to the high state 00295 * @param pGPIO : The base of GPIO peripheral on the chip 00296 * @param port : Port number 00297 * @param bit : Bit(s) in the port to set high 00298 * @return None 00299 * @note Any bit set as a '0' will not have it's state changed. This only 00300 * applies to ports configured as an output. It is recommended to use the 00301 * Chip_GPIO_SetPortOutHigh() function instead. 00302 */ 00303 STATIC INLINE void Chip_GPIO_SetValue(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t bit) 00304 { 00305 pGPIO[port].DATA [bit] = bit; 00306 } 00307 00308 /** 00309 * @brief Set selected GPIO output pins to the high state 00310 * @param pGPIO : The base of GPIO peripheral on the chip 00311 * @param port : Port number 00312 * @param pins : pins (0..n) to set high 00313 * @return None 00314 * @note Any bit set as a '0' will not have it's state changed. This only 00315 * applies to ports configured as an output. 00316 */ 00317 STATIC INLINE void Chip_GPIO_SetPortOutHigh(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pins) 00318 { 00319 pGPIO[port].DATA [pins] = 0xFFF; 00320 } 00321 00322 /** 00323 * @brief Set an individual GPIO output pin to the high state 00324 * @param pGPIO : The base of GPIO peripheral on the chip 00325 * @param port : Port number 00326 * @param pin : pin number (0..n) to set high 00327 * @return None 00328 * @note Any bit set as a '0' will not have it's state changed. This only 00329 * applies to ports configured as an output. 00330 */ 00331 STATIC INLINE void Chip_GPIO_SetPinOutHigh(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin) 00332 { 00333 pGPIO[port].DATA [1 << pin] = (1 << pin); 00334 } 00335 00336 /** 00337 * @brief Set a GPIO port/bit to the low state 00338 * @param pGPIO : The base of GPIO peripheral on the chip 00339 * @param port : Port number 00340 * @param bit : Bit(s) in the port to set low 00341 * @return None 00342 * @note Any bit set as a '0' will not have it's state changed. This only 00343 * applies to ports configured as an output. 00344 */ 00345 STATIC INLINE void Chip_GPIO_ClearValue(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t bit) 00346 { 00347 pGPIO[port].DATA [bit] = ~bit; 00348 } 00349 00350 /** 00351 * @brief Set selected GPIO output pins to the low state 00352 * @param pGPIO : The base of GPIO peripheral on the chip 00353 * @param port : Port number 00354 * @param pins : pins (0..n) to set low 00355 * @return None 00356 * @note Any bit set as a '0' will not have it's state changed. This only 00357 * applies to ports configured as an output. 00358 */ 00359 STATIC INLINE void Chip_GPIO_SetPortOutLow(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pins) 00360 { 00361 pGPIO[port].DATA [pins] = 0; 00362 } 00363 00364 /** 00365 * @brief Set an individual GPIO output pin to the low state 00366 * @param pGPIO : The base of GPIO peripheral on the chip 00367 * @param port : Port number 00368 * @param pin : pin number (0..n) to set low 00369 * @return None 00370 * @note Any bit set as a '0' will not have it's state changed. This only 00371 * applies to ports configured as an output. 00372 */ 00373 STATIC INLINE void Chip_GPIO_SetPinOutLow(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin) 00374 { 00375 pGPIO[port].DATA [1 << pin] = 0; 00376 } 00377 00378 /** 00379 * @brief Toggle selected GPIO output pins to the opposite state 00380 * @param pGPIO : The base of GPIO peripheral on the chip 00381 * @param port : Port number 00382 * @param pins : pins (0..n) to toggle 00383 * @return None 00384 * @note Any bit set as a '0' will not have it's state changed. This only 00385 * applies to ports configured as an output. 00386 */ 00387 STATIC INLINE void Chip_GPIO_SetPortToggle(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pins) 00388 { 00389 pGPIO[port].DATA [pins] ^= 0xFFF; 00390 } 00391 00392 /** 00393 * @brief Toggle an individual GPIO output pin to the opposite state 00394 * @param pGPIO : The base of GPIO peripheral on the chip 00395 * @param port : Port number 00396 * @param pin : pin number (0..n) to toggle 00397 * @return None 00398 * @note Any bit set as a '0' will not have it's state changed. This only 00399 * applies to ports configured as an output. 00400 */ 00401 STATIC INLINE void Chip_GPIO_SetPinToggle(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin) 00402 { 00403 pGPIO[port].DATA [1 << pin] ^= (1 << pin); 00404 } 00405 00406 /** 00407 * @brief Read current bit states for the selected port 00408 * @param pGPIO : The base of GPIO peripheral on the chip 00409 * @param port : Port number to read 00410 * @return Current value of GPIO port 00411 * @note The current states of the bits for the port are read, regardless of 00412 * whether the GPIO port bits are input or output. 00413 */ 00414 STATIC INLINE uint32_t Chip_GPIO_ReadValue(LPC_GPIO_T *pGPIO, uint8_t port) 00415 { 00416 return pGPIO[port].DATA [4095]; 00417 } 00418 00419 /** 00420 * @brief Configure the pins as edge sensitive for interrupts 00421 * @param pGPIO : The base of GPIO peripheral on the chip 00422 * @param port : Port number 00423 * @param pinmask : Pins to set to edge mode (ORed value of bits 0..11) 00424 * @return Nothing 00425 */ 00426 STATIC INLINE void Chip_GPIO_SetPinModeEdge(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00427 { 00428 pGPIO[port].IS &= ~pinmask; 00429 } 00430 00431 /** 00432 * @brief Configure the pins as level sensitive for interrupts 00433 * @param pGPIO : The base of GPIO peripheral on the chip 00434 * @param port : Port number 00435 * @param pinmask : Pins to set to level mode (ORed value of bits 0..11) 00436 * @return Nothing 00437 */ 00438 STATIC INLINE void Chip_GPIO_SetPinModeLevel(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00439 { 00440 pGPIO[port].IS |= pinmask; 00441 } 00442 00443 /** 00444 * @brief Returns current GPIO edge or high level interrupt configuration for all pins for a port 00445 * @param pGPIO : The base of GPIO peripheral on the chip 00446 * @param port : Port number 00447 * @return A bifield containing the edge/level interrupt configuration for each 00448 * pin for the selected port. Bit 0 = pin 0, 1 = pin 1. 00449 * For each bit, a 0 means the edge interrupt is configured, while a 1 means a level 00450 * interrupt is configured. Mask with this return value to determine the 00451 * edge/level configuration for each pin in a port. 00452 */ 00453 STATIC INLINE uint32_t Chip_GPIO_IsLevelEnabled(LPC_GPIO_T *pGPIO, uint8_t port) 00454 { 00455 return pGPIO[port].IS ; 00456 } 00457 00458 /** 00459 * @brief Sets GPIO interrupt configuration for both edges for selected pins 00460 * @param pGPIO : The base of GPIO peripheral on the chip 00461 * @param port : Port number 00462 * @param pinmask : Pins to set to dual edge mode (ORed value of bits 0..11) 00463 * @return Nothing 00464 */ 00465 STATIC INLINE void Chip_GPIO_SetEdgeModeBoth(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00466 { 00467 pGPIO[port].IBE |= pinmask; 00468 } 00469 00470 /** 00471 * @brief Sets GPIO interrupt configuration for a single edge for selected pins 00472 * @param pGPIO : The base of GPIO peripheral on the chip 00473 * @param port : Port number 00474 * @param pinmask : Pins to set to single edge mode (ORed value of bits 0..11) 00475 * @return Nothing 00476 */ 00477 STATIC INLINE void Chip_GPIO_SetEdgeModeSingle(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00478 { 00479 pGPIO[port].IBE &= ~pinmask; 00480 } 00481 00482 /** 00483 * @brief Returns current GPIO interrupt dual or single edge configuration for all pins for a port 00484 * @param pGPIO : The base of GPIO peripheral on the chip 00485 * @param port : Port number 00486 * @return A bifield containing the single/dual interrupt configuration for each 00487 * pin for the selected port. Bit 0 = pin 0, 1 = pin 1. 00488 * For each bit, a 0 means the interrupt triggers on a single edge (use the 00489 * Chip_GPIO_SetEdgeModeHigh() and Chip_GPIO_SetEdgeModeLow() functions to configure 00490 * selected edge), while a 1 means the interrupt triggers on both edges. Mask 00491 * with this return value to determine the edge/level configuration for each pin in 00492 * a port. 00493 */ 00494 STATIC INLINE uint32_t Chip_GPIO_GetEdgeModeDir(LPC_GPIO_T *pGPIO, uint8_t port) 00495 { 00496 return pGPIO[port].IBE ; 00497 } 00498 00499 /** 00500 * @brief Sets GPIO interrupt configuration when in single edge or level mode to high edge trigger or high level 00501 * @param pGPIO : The base of GPIO peripheral on the chip 00502 * @param port : Port number 00503 * @param pinmask : Pins to set to high mode (ORed value of bits 0..11) 00504 * @return Nothing 00505 * @note Use this function to select high level or high edge interrupt mode 00506 * for the selected pins on the selected port when not in dual edge mode. 00507 */ 00508 STATIC INLINE void Chip_GPIO_SetModeHigh(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00509 { 00510 pGPIO[port].IEV |= pinmask; 00511 } 00512 00513 /** 00514 * @brief Sets GPIO interrupt configuration when in single edge or level mode to low edge trigger or low level 00515 * @param pGPIO : The base of GPIO peripheral on the chip 00516 * @param port : Port number 00517 * @param pinmask : Pins to set to low mode (ORed value of bits 0..11) 00518 * @return Nothing 00519 * @note Use this function to select low level or low edge interrupt mode 00520 * for the selected pins on the selected port when not in dual edge mode. 00521 */ 00522 STATIC INLINE void Chip_GPIO_SetModeLow(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00523 { 00524 pGPIO[port].IEV &= ~pinmask; 00525 } 00526 00527 /** 00528 * @brief Returns current GPIO interrupt edge direction or level mode 00529 * @param pGPIO : The base of GPIO peripheral on the chip 00530 * @param port : Port number 00531 * @return A bifield containing the low or high direction of the interrupt 00532 * configuration for each pin for the selected port. Bit 0 = pin 0, 1 = pin 1. 00533 * For each bit, a 0 means the interrupt triggers on a low level or edge, while a 00534 * 1 means the interrupt triggers on a high level or edge. Mask with this 00535 * return value to determine the high/low configuration for each pin in a port. 00536 */ 00537 STATIC INLINE uint32_t Chip_GPIO_GetModeHighLow(LPC_GPIO_T *pGPIO, uint8_t port) 00538 { 00539 return pGPIO[port].IEV ; 00540 } 00541 00542 /** 00543 * @brief Enables interrupts for selected pins on a port 00544 * @param pGPIO : The base of GPIO peripheral on the chip 00545 * @param port : Port number 00546 * @param pinmask : Pins to enable interrupts for (ORed value of bits 0..11) 00547 * @return Nothing 00548 */ 00549 STATIC INLINE void Chip_GPIO_EnableInt(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00550 { 00551 pGPIO[port].IE |= pinmask; 00552 } 00553 00554 /** 00555 * @brief Disables interrupts for selected pins on a port 00556 * @param pGPIO : The base of GPIO peripheral on the chip 00557 * @param port : Port number 00558 * @param pinmask : Pins to disable interrupts for (ORed value of bits 0..11) 00559 * @return Nothing 00560 */ 00561 STATIC INLINE void Chip_GPIO_DisableInt(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00562 { 00563 pGPIO[port].IE &= ~pinmask; 00564 } 00565 00566 /** 00567 * @brief Returns current enable pin interrupts for a port 00568 * @param pGPIO : The base of GPIO peripheral on the chip 00569 * @param port : Port number 00570 * @return A bifield containing the enabled pin interrupts (0..11) 00571 */ 00572 STATIC INLINE uint32_t Chip_GPIO_GetEnabledInts(LPC_GPIO_T *pGPIO, uint8_t port) 00573 { 00574 return pGPIO[port].IE ; 00575 } 00576 00577 /** 00578 * @brief Returns raw interrupt pending status for pin interrupts for a port 00579 * @param pGPIO : The base of GPIO peripheral on the chip 00580 * @param port : Port number 00581 * @return A bifield containing the raw pending interrupt states for each pin (0..11) on the port 00582 */ 00583 STATIC INLINE uint32_t Chip_GPIO_GetRawInts(LPC_GPIO_T *pGPIO, uint8_t port) 00584 { 00585 return pGPIO[port].RIS ; 00586 } 00587 00588 /** 00589 * @brief Returns masked interrupt pending status for pin interrupts for a port 00590 * @param pGPIO : The base of GPIO peripheral on the chip 00591 * @param port : Port number 00592 * @return A bifield containing the masked pending interrupt states for each pin (0..11) on the port 00593 */ 00594 STATIC INLINE uint32_t Chip_GPIO_GetMaskedInts(LPC_GPIO_T *pGPIO, uint8_t port) 00595 { 00596 return pGPIO[port].MIS ; 00597 } 00598 00599 /** 00600 * @brief Clears pending interrupts for selected pins for a port 00601 * @param pGPIO : The base of GPIO peripheral on the chip 00602 * @param port : Port number 00603 * @param pinmask : Pins to clear interrupts for (ORed value of bits 0..11) 00604 * @return Nothing 00605 */ 00606 STATIC INLINE void Chip_GPIO_ClearInts(LPC_GPIO_T *pGPIO, uint8_t port, uint32_t pinmask) 00607 { 00608 pGPIO[port].IC = pinmask; 00609 } 00610 00611 /** 00612 * @brief GPIO interrupt mode definitions 00613 */ 00614 typedef enum { 00615 GPIO_INT_ACTIVE_LOW_LEVEL = 0x0, /*!< Selects interrupt on pin to be triggered on LOW level */ 00616 GPIO_INT_ACTIVE_HIGH_LEVEL = 0x1, /*!< Selects interrupt on pin to be triggered on HIGH level */ 00617 GPIO_INT_FALLING_EDGE = 0x2, /*!< Selects interrupt on pin to be triggered on FALLING level */ 00618 GPIO_INT_RISING_EDGE = 0x3, /*!< Selects interrupt on pin to be triggered on RISING level */ 00619 GPIO_INT_BOTH_EDGES = 0x6 /*!< Selects interrupt on pin to be triggered on both edges */ 00620 } GPIO_INT_MODE_T; 00621 00622 /** 00623 * @brief Composite function for setting up a full interrupt configuration for a single pin 00624 * @param pGPIO : The base of GPIO peripheral on the chip 00625 * @param port : Port number 00626 * @param pin : Pin number (0..11) 00627 * @param modeFlags : GPIO interrupt mode selection 00628 * @return Nothing 00629 */ 00630 void Chip_GPIO_SetupPinInt(LPC_GPIO_T *pGPIO, uint8_t port, uint8_t pin, GPIO_INT_MODE_T mode); 00631 00632 #endif /* defined(CHIP_LPC11CXX) || defined(CHIP_LPC110X) || defined(CHIP_LPC11XXLV) || defined(CHIP_LPC1125) */ 00633 00634 /** 00635 * @} 00636 */ 00637 00638 #ifdef __cplusplus 00639 } 00640 #endif 00641 00642 #endif /* __GPIO_11XX_2_H_ */
Generated on Tue Jul 12 2022 17:17:32 by
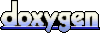