libuav original
Dependents: UAVCAN UAVCAN_Subscriber
event_tracer.hpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #pragma once 00006 00007 #include <iostream> 00008 #include <string> 00009 #include <list> 00010 #include <uavcan/protocol/dynamic_node_id_server/event.hpp> 00011 #include <uavcan/time.hpp> 00012 #include "../../clock.hpp" 00013 00014 00015 class EventTracer : public uavcan::dynamic_node_id_server::IEventTracer 00016 { 00017 struct EventLogEntry 00018 { 00019 const uavcan::dynamic_node_id_server::TraceCode code; 00020 const uavcan::int64_t argument; 00021 00022 EventLogEntry(uavcan::dynamic_node_id_server::TraceCode arg_code, uavcan::int64_t arg_argument) 00023 : code(arg_code) 00024 , argument(arg_argument) 00025 { } 00026 }; 00027 00028 const std::string id_; 00029 const uavcan::MonotonicTime startup_ts_; 00030 std::list<EventLogEntry> event_log_; 00031 00032 public: 00033 EventTracer() : 00034 startup_ts_(SystemClockDriver().getMonotonic()) 00035 { } 00036 00037 EventTracer(const std::string& id) : 00038 id_(id), 00039 startup_ts_(SystemClockDriver().getMonotonic()) 00040 { } 00041 00042 virtual void onEvent(uavcan::dynamic_node_id_server::TraceCode code, uavcan::int64_t argument) 00043 { 00044 const uavcan::MonotonicDuration ts = SystemClockDriver().getMonotonic() - startup_ts_; 00045 std::cout << "EVENT [" << id_ << "]\t" << ts.toString() << "\t" 00046 << int(code) << "\t" << getEventName(code) << "\t" << argument << std::endl; 00047 event_log_.push_back(EventLogEntry(code, argument)); 00048 } 00049 00050 unsigned countEvents(const uavcan::dynamic_node_id_server::TraceCode code) const 00051 { 00052 unsigned count = 0; 00053 for (std::list<EventLogEntry>::const_iterator it = event_log_.begin(); it != event_log_.end(); ++it) 00054 { 00055 count += (it->code == code) ? 1U : 0U; 00056 } 00057 return count; 00058 } 00059 00060 uavcan::int64_t getLastEventArgumentOrFail(const uavcan::dynamic_node_id_server::TraceCode code) const 00061 { 00062 for (std::list<EventLogEntry>::const_reverse_iterator it = event_log_.rbegin(); it != event_log_.rend(); ++it) 00063 { 00064 if (it->code == code) 00065 { 00066 return it->argument; 00067 } 00068 } 00069 00070 std::cout << "No such event in the event log, code " << int(code) << ", log length " << event_log_.size() 00071 << std::endl; 00072 00073 throw std::runtime_error("EventTracer::getLastEventArgumentOrFail()"); 00074 } 00075 00076 unsigned getNumEvents() const { return static_cast<unsigned>(event_log_.size()); } 00077 };
Generated on Tue Jul 12 2022 17:17:31 by
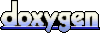