libuav original
Dependents: UAVCAN UAVCAN_Subscriber
event.hpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_PROTOCOL_DYNAMIC_NODE_ID_SERVER_EVENT_HPP_INCLUDED 00006 #define UAVCAN_PROTOCOL_DYNAMIC_NODE_ID_SERVER_EVENT_HPP_INCLUDED 00007 00008 #include <uavcan/build_config.hpp> 00009 #include <uavcan/protocol/dynamic_node_id_server/types.hpp> 00010 00011 namespace uavcan 00012 { 00013 namespace dynamic_node_id_server 00014 { 00015 /** 00016 * @ref IEventTracer. 00017 * Event codes cannot be changed, only new ones can be added. 00018 */ 00019 enum UAVCAN_EXPORT TraceCode 00020 { 00021 // Event name Argument 00022 // 0 00023 TraceError, // error code (may be negated) 00024 TraceRaftLogLastIndexRestored, // recovered last index value 00025 TraceRaftLogAppend, // index of new entry 00026 TraceRaftLogRemove, // new last index value 00027 TraceRaftCurrentTermRestored, // current term 00028 // 5 00029 TraceRaftCurrentTermUpdate, // current term 00030 TraceRaftVotedForRestored, // value of votedFor 00031 TraceRaftVotedForUpdate, // value of votedFor 00032 TraceRaftDiscoveryBroadcast, // number of known servers 00033 TraceRaftNewServerDiscovered, // node ID of the new server 00034 // 10 00035 TraceRaftDiscoveryReceived, // node ID of the sender 00036 TraceRaftClusterSizeInited, // cluster size 00037 TraceRaftBadClusterSizeReceived, // received cluster size 00038 TraceRaftCoreInited, // update interval in usec 00039 TraceRaftStateSwitch, // 0 - Follower, 1 - Candidate, 2 - Leader 00040 // 15 00041 Trace0, 00042 TraceRaftNewLogEntry, // node ID value 00043 TraceRaftRequestIgnored, // node ID of the client 00044 TraceRaftVoteRequestReceived, // node ID of the client 00045 TraceRaftVoteRequestSucceeded, // node ID of the server 00046 // 20 00047 TraceRaftVoteRequestInitiation, // node ID of the server 00048 TraceRaftPersistStateUpdateError, // negative error code 00049 TraceRaftCommitIndexUpdate, // new commit index value 00050 TraceRaftNewerTermInResponse, // new term value 00051 TraceRaftNewEntryCommitted, // new commit index value 00052 // 25 00053 TraceRaftAppendEntriesCallFailure, // error code (may be negated) 00054 TraceRaftElectionComplete, // number of votes collected 00055 TraceRaftAppendEntriesRespUnsucfl, // node ID of the client 00056 Trace2, 00057 Trace3, 00058 // 30 00059 TraceAllocationFollowupResponse, // number of unique ID bytes in this response 00060 TraceAllocationFollowupDenied, // reason code (see sources for details) 00061 TraceAllocationFollowupTimeout, // timeout value in microseconds 00062 TraceAllocationBadRequest, // number of unique ID bytes in this request 00063 TraceAllocationUnexpectedStage, // stage number in the request - 1, 2, or 3 00064 // 35 00065 TraceAllocationRequestAccepted, // number of bytes of unique ID after request 00066 TraceAllocationExchangeComplete, // first 8 bytes of unique ID interpreted as signed 64 bit big endian 00067 TraceAllocationResponse, // allocated node ID 00068 TraceAllocationActivity, // source node ID of the message 00069 Trace12, 00070 // 40 00071 TraceDiscoveryNewNodeFound, // node ID 00072 TraceDiscoveryCommitCacheUpdated, // node ID marked as committed 00073 TraceDiscoveryNodeFinalized, // node ID in lower 7 bits, bit 8 (256, 0x100) is set if unique ID is known 00074 TraceDiscoveryGetNodeInfoFailure, // node ID 00075 TraceDiscoveryTimerStart, // interval in microseconds 00076 // 45 00077 TraceDiscoveryTimerStop, // reason code (see sources for details) 00078 TraceDiscoveryGetNodeInfoRequest, // target node ID 00079 TraceDiscoveryNodeRestartDetected, // node ID 00080 TraceDiscoveryNodeRemoved, // node ID 00081 Trace22, 00082 // 50 00083 00084 NumTraceCodes 00085 }; 00086 00087 /** 00088 * This interface allows the application to trace events that happen in the server. 00089 */ 00090 class UAVCAN_EXPORT IEventTracer 00091 { 00092 public: 00093 #if UAVCAN_TOSTRING 00094 /** 00095 * It is safe to call this function with any argument. 00096 * If the event code is out of range, an assertion failure will be triggered and an error text will be returned. 00097 */ 00098 static const char* getEventName(TraceCode code) 00099 { 00100 // import re;m = lambda s:',\n'.join('"%s"' % x for x in re.findall(r'\ {4}Trace[0-9]*([A-Za-z0-9]*),',s)) 00101 static const char* const Strings[] = 00102 { 00103 "Error", 00104 "RaftLogLastIndexRestored", 00105 "RaftLogAppend", 00106 "RaftLogRemove", 00107 "RaftCurrentTermRestored", 00108 "RaftCurrentTermUpdate", 00109 "RaftVotedForRestored", 00110 "RaftVotedForUpdate", 00111 "RaftDiscoveryBroadcast", 00112 "RaftNewServerDiscovered", 00113 "RaftDiscoveryReceived", 00114 "RaftClusterSizeInited", 00115 "RaftBadClusterSizeReceived", 00116 "RaftCoreInited", 00117 "RaftStateSwitch", 00118 "", 00119 "RaftNewLogEntry", 00120 "RaftRequestIgnored", 00121 "RaftVoteRequestReceived", 00122 "RaftVoteRequestSucceeded", 00123 "RaftVoteRequestInitiation", 00124 "RaftPersistStateUpdateError", 00125 "RaftCommitIndexUpdate", 00126 "RaftNewerTermInResponse", 00127 "RaftNewEntryCommitted", 00128 "RaftAppendEntriesCallFailure", 00129 "RaftElectionComplete", 00130 "RaftAppendEntriesRespUnsucfl", 00131 "", 00132 "", 00133 "AllocationFollowupResponse", 00134 "AllocationFollowupDenied", 00135 "AllocationFollowupTimeout", 00136 "AllocationBadRequest", 00137 "AllocationUnexpectedStage", 00138 "AllocationRequestAccepted", 00139 "AllocationExchangeComplete", 00140 "AllocationResponse", 00141 "AllocationActivity", 00142 "", 00143 "DiscoveryNewNodeFound", 00144 "DiscoveryCommitCacheUpdated", 00145 "DiscoveryNodeFinalized", 00146 "DiscoveryGetNodeInfoFailure", 00147 "DiscoveryTimerStart", 00148 "DiscoveryTimerStop", 00149 "DiscoveryGetNodeInfoRequest", 00150 "DiscoveryNodeRestartDetected", 00151 "DiscoveryNodeRemoved", 00152 "" 00153 }; 00154 uavcan::StaticAssert<sizeof(Strings) / sizeof(Strings[0]) == NumTraceCodes>::check(); 00155 UAVCAN_ASSERT(code < NumTraceCodes); 00156 // coverity[dead_error_line] 00157 return (code < NumTraceCodes) ? Strings[static_cast<unsigned>(code)] : "INVALID_EVENT_CODE"; 00158 } 00159 #endif 00160 00161 /** 00162 * The server invokes this method every time it believes that a noteworthy event has happened. 00163 * It is guaranteed that event code values will never change, but new ones can be added in future. This ensures 00164 * full backward compatibility. 00165 * @param event_code Event code, see the sources for the enum with values. 00166 * @param event_argument Value associated with the event; its meaning depends on the event code. 00167 */ 00168 virtual void onEvent(TraceCode event_code, int64_t event_argument) = 0; 00169 00170 virtual ~IEventTracer() { } 00171 }; 00172 00173 } 00174 } 00175 00176 #endif // Include guard
Generated on Tue Jul 12 2022 17:17:31 by
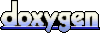