libuav original
Dependents: UAVCAN UAVCAN_Subscriber
clock.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #pragma once 00006 00007 #include <uavcan/driver/system_clock.hpp> 00008 00009 namespace uavcan_lpc11c24 00010 { 00011 namespace clock 00012 { 00013 /** 00014 * Starts the clock. 00015 * Can be called multiple times, only the first call will be effective. 00016 */ 00017 void init(); 00018 00019 /** 00020 * Returns current monotonic time passed since the moment when clock::init() was called. 00021 * Note that both monotonic and UTC clocks are implemented using SysTick timer. 00022 */ 00023 uavcan::MonotonicTime getMonotonic(); 00024 00025 /** 00026 * Returns UTC time if it has been set, otherwise returns zero time. 00027 * Note that both monotonic and UTC clocks are implemented using SysTick timer. 00028 */ 00029 uavcan::UtcTime getUtc(); 00030 00031 /** 00032 * Performs UTC time adjustment. 00033 * The UTC time will be zero until first adjustment has been performed. 00034 */ 00035 void adjustUtc(uavcan::UtcDuration adjustment); 00036 00037 /** 00038 * Returns clock error sampled at previous UTC adjustment. 00039 * Positive if the hardware timer is slower than reference time. 00040 */ 00041 uavcan::UtcDuration getPrevUtcAdjustment(); 00042 00043 } 00044 00045 /** 00046 * Adapter for uavcan::ISystemClock. 00047 */ 00048 class SystemClock : public uavcan::ISystemClock, uavcan::Noncopyable 00049 { 00050 static SystemClock self; 00051 00052 SystemClock() { } 00053 00054 uavcan::MonotonicTime getMonotonic() const override { return clock::getMonotonic(); } 00055 uavcan::UtcTime getUtc() const override { return clock::getUtc(); } 00056 void adjustUtc(uavcan::UtcDuration adjustment) override { clock::adjustUtc(adjustment); } 00057 00058 public: 00059 /** 00060 * Calls clock::init() as needed. 00061 */ 00062 static SystemClock& instance(); 00063 }; 00064 00065 }
Generated on Tue Jul 12 2022 17:17:30 by
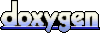