libuav original
Dependents: UAVCAN UAVCAN_Subscriber
can.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #pragma once 00006 00007 #include <uavcan/driver/can.hpp> 00008 00009 namespace uavcan_lpc11c24 00010 { 00011 /** 00012 * This class implements CAN driver interface for libuavcan. 00013 * No configuration needed other than CAN baudrate. 00014 * This class is a singleton. 00015 */ 00016 class CanDriver 00017 : public uavcan::ICanDriver 00018 , public uavcan::ICanIface 00019 , uavcan::Noncopyable 00020 { 00021 static CanDriver self; 00022 00023 CanDriver() { } 00024 00025 public: 00026 /** 00027 * Returns the singleton reference. 00028 * No other copies can be created. 00029 */ 00030 static CanDriver& instance() { return self; } 00031 00032 /** 00033 * Attempts to detect bit rate of the CAN bus. 00034 * This function may block for up to X seconds, where X is the number of bit rates to try. 00035 * This function is NOT guaranteed to reset the CAN controller upon return. 00036 * @return On success: detected bit rate, in bits per second. 00037 * On failure: zero. 00038 */ 00039 static uavcan::uint32_t detectBitRate(void (*idle_callback)() = nullptr); 00040 00041 /** 00042 * Returns negative value if the requested baudrate can't be used. 00043 * Returns zero if OK. 00044 */ 00045 int init(uavcan::uint32_t bitrate); 00046 00047 bool hasReadyRx() const; 00048 bool hasEmptyTx() const; 00049 00050 /** 00051 * This method will return true only if there was any CAN bus activity since previous call of this method. 00052 * This is intended to be used for LED iface activity indicators. 00053 */ 00054 bool hadActivity(); 00055 00056 /** 00057 * Returns the number of times the RX queue was overrun. 00058 */ 00059 uavcan::uint32_t getRxQueueOverflowCount() const; 00060 00061 /** 00062 * Whether the controller is currently in bus off state. 00063 * Note that the driver recovers the CAN controller from the bus off state automatically! 00064 * Therefore, this method serves only monitoring purposes and is not necessary to use. 00065 */ 00066 bool isInBusOffState() const; 00067 00068 uavcan::int16_t send(const uavcan::CanFrame& frame, 00069 uavcan::MonotonicTime tx_deadline, 00070 uavcan::CanIOFlags flags) override; 00071 00072 uavcan::int16_t receive(uavcan::CanFrame& out_frame, 00073 uavcan::MonotonicTime& out_ts_monotonic, 00074 uavcan::UtcTime& out_ts_utc, 00075 uavcan::CanIOFlags& out_flags) override; 00076 00077 uavcan::int16_t select(uavcan::CanSelectMasks& inout_masks, 00078 const uavcan::CanFrame* (&)[uavcan::MaxCanIfaces], 00079 uavcan::MonotonicTime blocking_deadline) override; 00080 00081 uavcan::int16_t configureFilters(const uavcan::CanFilterConfig* filter_configs, 00082 uavcan::uint16_t num_configs) override; 00083 00084 uavcan::uint64_t getErrorCount() const override; 00085 00086 uavcan::uint16_t getNumFilters() const override; 00087 00088 uavcan::ICanIface* getIface(uavcan::uint8_t iface_index) override; 00089 00090 uavcan::uint8_t getNumIfaces() const override; 00091 }; 00092 00093 }
Generated on Tue Jul 12 2022 17:17:30 by
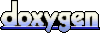