libuav original
Dependents: UAVCAN UAVCAN_Subscriber
core_cmInstr.h
00001 /**************************************************************************//** 00002 * @file core_cmInstr.h 00003 * @brief CMSIS Cortex-M Core Instruction Access Header File 00004 * @version V3.01 00005 * @date 06. March 2012 00006 * 00007 * @note 00008 * Copyright (C) 2009-2012 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef __CORE_CMINSTR_H 00025 #define __CORE_CMINSTR_H 00026 00027 00028 /* ########################## Core Instruction Access ######################### */ 00029 /** \defgroup CMSIS_Core_InstructionInterface CMSIS Core Instruction Interface 00030 Access to dedicated instructions 00031 @{ 00032 */ 00033 00034 #if defined ( __CC_ARM ) /*------------------RealView Compiler -----------------*/ 00035 /* ARM armcc specific functions */ 00036 00037 #if (__ARMCC_VERSION < 400677) 00038 #error "Please use ARM Compiler Toolchain V4.0.677 or later!" 00039 #endif 00040 00041 00042 /** \brief No Operation 00043 00044 No Operation does nothing. This instruction can be used for code alignment purposes. 00045 */ 00046 #define __NOP __nop 00047 00048 00049 /** \brief Wait For Interrupt 00050 00051 Wait For Interrupt is a hint instruction that suspends execution 00052 until one of a number of events occurs. 00053 */ 00054 #define __WFI __wfi 00055 00056 00057 /** \brief Wait For Event 00058 00059 Wait For Event is a hint instruction that permits the processor to enter 00060 a low-power state until one of a number of events occurs. 00061 */ 00062 #define __WFE __wfe 00063 00064 00065 /** \brief Send Event 00066 00067 Send Event is a hint instruction. It causes an event to be signaled to the CPU. 00068 */ 00069 #define __SEV __sev 00070 00071 00072 /** \brief Instruction Synchronization Barrier 00073 00074 Instruction Synchronization Barrier flushes the pipeline in the processor, 00075 so that all instructions following the ISB are fetched from cache or 00076 memory, after the instruction has been completed. 00077 */ 00078 #define __ISB() __isb(0xF) 00079 00080 00081 /** \brief Data Synchronization Barrier 00082 00083 This function acts as a special kind of Data Memory Barrier. 00084 It completes when all explicit memory accesses before this instruction complete. 00085 */ 00086 #define __DSB() __dsb(0xF) 00087 00088 00089 /** \brief Data Memory Barrier 00090 00091 This function ensures the apparent order of the explicit memory operations before 00092 and after the instruction, without ensuring their completion. 00093 */ 00094 #define __DMB() __dmb(0xF) 00095 00096 00097 /** \brief Reverse byte order (32 bit) 00098 00099 This function reverses the byte order in integer value. 00100 00101 \param [in] value Value to reverse 00102 \return Reversed value 00103 */ 00104 #define __REV __rev 00105 00106 00107 /** \brief Reverse byte order (16 bit) 00108 00109 This function reverses the byte order in two unsigned short values. 00110 00111 \param [in] value Value to reverse 00112 \return Reversed value 00113 */ 00114 __attribute__((section(".rev16_text"))) __STATIC_INLINE __ASM uint32_t __REV16(uint32_t value) 00115 { 00116 rev16 r0, r0 00117 bx lr 00118 } 00119 00120 00121 /** \brief Reverse byte order in signed short value 00122 00123 This function reverses the byte order in a signed short value with sign extension to integer. 00124 00125 \param [in] value Value to reverse 00126 \return Reversed value 00127 */ 00128 __attribute__((section(".revsh_text"))) __STATIC_INLINE __ASM int32_t __REVSH(int32_t value) 00129 { 00130 revsh r0, r0 00131 bx lr 00132 } 00133 00134 00135 /** \brief Rotate Right in unsigned value (32 bit) 00136 00137 This function Rotate Right (immediate) provides the value of the contents of a register rotated by a variable number of bits. 00138 00139 \param [in] value Value to rotate 00140 \param [in] value Number of Bits to rotate 00141 \return Rotated value 00142 */ 00143 #define __ROR __ror 00144 00145 00146 #if (__CORTEX_M >= 0x03) 00147 00148 /** \brief Reverse bit order of value 00149 00150 This function reverses the bit order of the given value. 00151 00152 \param [in] value Value to reverse 00153 \return Reversed value 00154 */ 00155 #define __RBIT __rbit 00156 00157 00158 /** \brief LDR Exclusive (8 bit) 00159 00160 This function performs a exclusive LDR command for 8 bit value. 00161 00162 \param [in] ptr Pointer to data 00163 \return value of type uint8_t at (*ptr) 00164 */ 00165 #define __LDREXB(ptr) ((uint8_t ) __ldrex(ptr)) 00166 00167 00168 /** \brief LDR Exclusive (16 bit) 00169 00170 This function performs a exclusive LDR command for 16 bit values. 00171 00172 \param [in] ptr Pointer to data 00173 \return value of type uint16_t at (*ptr) 00174 */ 00175 #define __LDREXH(ptr) ((uint16_t) __ldrex(ptr)) 00176 00177 00178 /** \brief LDR Exclusive (32 bit) 00179 00180 This function performs a exclusive LDR command for 32 bit values. 00181 00182 \param [in] ptr Pointer to data 00183 \return value of type uint32_t at (*ptr) 00184 */ 00185 #define __LDREXW(ptr) ((uint32_t ) __ldrex(ptr)) 00186 00187 00188 /** \brief STR Exclusive (8 bit) 00189 00190 This function performs a exclusive STR command for 8 bit values. 00191 00192 \param [in] value Value to store 00193 \param [in] ptr Pointer to location 00194 \return 0 Function succeeded 00195 \return 1 Function failed 00196 */ 00197 #define __STREXB(value, ptr) __strex(value, ptr) 00198 00199 00200 /** \brief STR Exclusive (16 bit) 00201 00202 This function performs a exclusive STR command for 16 bit values. 00203 00204 \param [in] value Value to store 00205 \param [in] ptr Pointer to location 00206 \return 0 Function succeeded 00207 \return 1 Function failed 00208 */ 00209 #define __STREXH(value, ptr) __strex(value, ptr) 00210 00211 00212 /** \brief STR Exclusive (32 bit) 00213 00214 This function performs a exclusive STR command for 32 bit values. 00215 00216 \param [in] value Value to store 00217 \param [in] ptr Pointer to location 00218 \return 0 Function succeeded 00219 \return 1 Function failed 00220 */ 00221 #define __STREXW(value, ptr) __strex(value, ptr) 00222 00223 00224 /** \brief Remove the exclusive lock 00225 00226 This function removes the exclusive lock which is created by LDREX. 00227 00228 */ 00229 #define __CLREX __clrex 00230 00231 00232 /** \brief Signed Saturate 00233 00234 This function saturates a signed value. 00235 00236 \param [in] value Value to be saturated 00237 \param [in] sat Bit position to saturate to (1..32) 00238 \return Saturated value 00239 */ 00240 #define __SSAT __ssat 00241 00242 00243 /** \brief Unsigned Saturate 00244 00245 This function saturates an unsigned value. 00246 00247 \param [in] value Value to be saturated 00248 \param [in] sat Bit position to saturate to (0..31) 00249 \return Saturated value 00250 */ 00251 #define __USAT __usat 00252 00253 00254 /** \brief Count leading zeros 00255 00256 This function counts the number of leading zeros of a data value. 00257 00258 \param [in] value Value to count the leading zeros 00259 \return number of leading zeros in value 00260 */ 00261 #define __CLZ __clz 00262 00263 #endif /* (__CORTEX_M >= 0x03) */ 00264 00265 00266 00267 #elif defined ( __ICCARM__ ) /*------------------ ICC Compiler -------------------*/ 00268 /* IAR iccarm specific functions */ 00269 00270 #include <cmsis_iar.h> 00271 00272 00273 #elif defined ( __TMS470__ ) /*---------------- TI CCS Compiler ------------------*/ 00274 /* TI CCS specific functions */ 00275 00276 #include <cmsis_ccs.h> 00277 00278 00279 #elif defined ( __GNUC__ ) /*------------------ GNU Compiler ---------------------*/ 00280 /* GNU gcc specific functions */ 00281 00282 /** \brief No Operation 00283 00284 No Operation does nothing. This instruction can be used for code alignment purposes. 00285 */ 00286 __attribute__( ( always_inline ) ) __STATIC_INLINE void __NOP(void) 00287 { 00288 __ASM volatile ("nop"); 00289 } 00290 00291 00292 /** \brief Wait For Interrupt 00293 00294 Wait For Interrupt is a hint instruction that suspends execution 00295 until one of a number of events occurs. 00296 */ 00297 __attribute__( ( always_inline ) ) __STATIC_INLINE void __WFI(void) 00298 { 00299 __ASM volatile ("wfi"); 00300 } 00301 00302 00303 /** \brief Wait For Event 00304 00305 Wait For Event is a hint instruction that permits the processor to enter 00306 a low-power state until one of a number of events occurs. 00307 */ 00308 __attribute__( ( always_inline ) ) __STATIC_INLINE void __WFE(void) 00309 { 00310 __ASM volatile ("wfe"); 00311 } 00312 00313 00314 /** \brief Send Event 00315 00316 Send Event is a hint instruction. It causes an event to be signaled to the CPU. 00317 */ 00318 __attribute__( ( always_inline ) ) __STATIC_INLINE void __SEV(void) 00319 { 00320 __ASM volatile ("sev"); 00321 } 00322 00323 00324 /** \brief Instruction Synchronization Barrier 00325 00326 Instruction Synchronization Barrier flushes the pipeline in the processor, 00327 so that all instructions following the ISB are fetched from cache or 00328 memory, after the instruction has been completed. 00329 */ 00330 __attribute__( ( always_inline ) ) __STATIC_INLINE void __ISB(void) 00331 { 00332 __ASM volatile ("isb"); 00333 } 00334 00335 00336 /** \brief Data Synchronization Barrier 00337 00338 This function acts as a special kind of Data Memory Barrier. 00339 It completes when all explicit memory accesses before this instruction complete. 00340 */ 00341 __attribute__( ( always_inline ) ) __STATIC_INLINE void __DSB(void) 00342 { 00343 __ASM volatile ("dsb"); 00344 } 00345 00346 00347 /** \brief Data Memory Barrier 00348 00349 This function ensures the apparent order of the explicit memory operations before 00350 and after the instruction, without ensuring their completion. 00351 */ 00352 __attribute__( ( always_inline ) ) __STATIC_INLINE void __DMB(void) 00353 { 00354 __ASM volatile ("dmb"); 00355 } 00356 00357 00358 /** \brief Reverse byte order (32 bit) 00359 00360 This function reverses the byte order in integer value. 00361 00362 \param [in] value Value to reverse 00363 \return Reversed value 00364 */ 00365 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __REV(uint32_t value) 00366 { 00367 uint32_t result; 00368 00369 __ASM volatile ("rev %0, %1" : "=r" (result) : "r" (value) ); 00370 return(result); 00371 } 00372 00373 00374 /** \brief Reverse byte order (16 bit) 00375 00376 This function reverses the byte order in two unsigned short values. 00377 00378 \param [in] value Value to reverse 00379 \return Reversed value 00380 */ 00381 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __REV16(uint32_t value) 00382 { 00383 uint32_t result; 00384 00385 __ASM volatile ("rev16 %0, %1" : "=r" (result) : "r" (value) ); 00386 return(result); 00387 } 00388 00389 00390 /** \brief Reverse byte order in signed short value 00391 00392 This function reverses the byte order in a signed short value with sign extension to integer. 00393 00394 \param [in] value Value to reverse 00395 \return Reversed value 00396 */ 00397 __attribute__( ( always_inline ) ) __STATIC_INLINE int32_t __REVSH(int32_t value) 00398 { 00399 uint32_t result; 00400 00401 __ASM volatile ("revsh %0, %1" : "=r" (result) : "r" (value) ); 00402 return(result); 00403 } 00404 00405 00406 /** \brief Rotate Right in unsigned value (32 bit) 00407 00408 This function Rotate Right (immediate) provides the value of the contents of a register rotated by a variable number of bits. 00409 00410 \param [in] value Value to rotate 00411 \param [in] value Number of Bits to rotate 00412 \return Rotated value 00413 */ 00414 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __ROR(uint32_t op1, uint32_t op2) 00415 { 00416 00417 __ASM volatile ("ror %0, %0, %1" : "+r" (op1) : "r" (op2) ); 00418 return(op1); 00419 } 00420 00421 00422 #if (__CORTEX_M >= 0x03) 00423 00424 /** \brief Reverse bit order of value 00425 00426 This function reverses the bit order of the given value. 00427 00428 \param [in] value Value to reverse 00429 \return Reversed value 00430 */ 00431 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __RBIT(uint32_t value) 00432 { 00433 uint32_t result; 00434 00435 __ASM volatile ("rbit %0, %1" : "=r" (result) : "r" (value) ); 00436 return(result); 00437 } 00438 00439 00440 /** \brief LDR Exclusive (8 bit) 00441 00442 This function performs a exclusive LDR command for 8 bit value. 00443 00444 \param [in] ptr Pointer to data 00445 \return value of type uint8_t at (*ptr) 00446 */ 00447 __attribute__( ( always_inline ) ) __STATIC_INLINE uint8_t __LDREXB(volatile uint8_t *addr) 00448 { 00449 uint8_t result; 00450 00451 __ASM volatile ("ldrexb %0, [%1]" : "=r" (result) : "r" (addr) ); 00452 return(result); 00453 } 00454 00455 00456 /** \brief LDR Exclusive (16 bit) 00457 00458 This function performs a exclusive LDR command for 16 bit values. 00459 00460 \param [in] ptr Pointer to data 00461 \return value of type uint16_t at (*ptr) 00462 */ 00463 __attribute__( ( always_inline ) ) __STATIC_INLINE uint16_t __LDREXH(volatile uint16_t *addr) 00464 { 00465 uint16_t result; 00466 00467 __ASM volatile ("ldrexh %0, [%1]" : "=r" (result) : "r" (addr) ); 00468 return(result); 00469 } 00470 00471 00472 /** \brief LDR Exclusive (32 bit) 00473 00474 This function performs a exclusive LDR command for 32 bit values. 00475 00476 \param [in] ptr Pointer to data 00477 \return value of type uint32_t at (*ptr) 00478 */ 00479 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __LDREXW(volatile uint32_t *addr) 00480 { 00481 uint32_t result; 00482 00483 __ASM volatile ("ldrex %0, [%1]" : "=r" (result) : "r" (addr) ); 00484 return(result); 00485 } 00486 00487 00488 /** \brief STR Exclusive (8 bit) 00489 00490 This function performs a exclusive STR command for 8 bit values. 00491 00492 \param [in] value Value to store 00493 \param [in] ptr Pointer to location 00494 \return 0 Function succeeded 00495 \return 1 Function failed 00496 */ 00497 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __STREXB(uint8_t value, volatile uint8_t *addr) 00498 { 00499 uint32_t result; 00500 00501 __ASM volatile ("strexb %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00502 return(result); 00503 } 00504 00505 00506 /** \brief STR Exclusive (16 bit) 00507 00508 This function performs a exclusive STR command for 16 bit values. 00509 00510 \param [in] value Value to store 00511 \param [in] ptr Pointer to location 00512 \return 0 Function succeeded 00513 \return 1 Function failed 00514 */ 00515 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __STREXH(uint16_t value, volatile uint16_t *addr) 00516 { 00517 uint32_t result; 00518 00519 __ASM volatile ("strexh %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00520 return(result); 00521 } 00522 00523 00524 /** \brief STR Exclusive (32 bit) 00525 00526 This function performs a exclusive STR command for 32 bit values. 00527 00528 \param [in] value Value to store 00529 \param [in] ptr Pointer to location 00530 \return 0 Function succeeded 00531 \return 1 Function failed 00532 */ 00533 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __STREXW(uint32_t value, volatile uint32_t *addr) 00534 { 00535 uint32_t result; 00536 00537 __ASM volatile ("strex %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00538 return(result); 00539 } 00540 00541 00542 /** \brief Remove the exclusive lock 00543 00544 This function removes the exclusive lock which is created by LDREX. 00545 00546 */ 00547 __attribute__( ( always_inline ) ) __STATIC_INLINE void __CLREX(void) 00548 { 00549 __ASM volatile ("clrex"); 00550 } 00551 00552 00553 /** \brief Signed Saturate 00554 00555 This function saturates a signed value. 00556 00557 \param [in] value Value to be saturated 00558 \param [in] sat Bit position to saturate to (1..32) 00559 \return Saturated value 00560 */ 00561 #define __SSAT(ARG1,ARG2) \ 00562 ({ \ 00563 uint32_t __RES, __ARG1 = (ARG1); \ 00564 __ASM ("ssat %0, %1, %2" : "=r" (__RES) : "I" (ARG2), "r" (__ARG1) ); \ 00565 __RES; \ 00566 }) 00567 00568 00569 /** \brief Unsigned Saturate 00570 00571 This function saturates an unsigned value. 00572 00573 \param [in] value Value to be saturated 00574 \param [in] sat Bit position to saturate to (0..31) 00575 \return Saturated value 00576 */ 00577 #define __USAT(ARG1,ARG2) \ 00578 ({ \ 00579 uint32_t __RES, __ARG1 = (ARG1); \ 00580 __ASM ("usat %0, %1, %2" : "=r" (__RES) : "I" (ARG2), "r" (__ARG1) ); \ 00581 __RES; \ 00582 }) 00583 00584 00585 /** \brief Count leading zeros 00586 00587 This function counts the number of leading zeros of a data value. 00588 00589 \param [in] value Value to count the leading zeros 00590 \return number of leading zeros in value 00591 */ 00592 __attribute__( ( always_inline ) ) __STATIC_INLINE uint8_t __CLZ(uint32_t value) 00593 { 00594 uint8_t result; 00595 00596 __ASM volatile ("clz %0, %1" : "=r" (result) : "r" (value) ); 00597 return(result); 00598 } 00599 00600 #endif /* (__CORTEX_M >= 0x03) */ 00601 00602 00603 00604 00605 #elif defined ( __TASKING__ ) /*------------------ TASKING Compiler --------------*/ 00606 /* TASKING carm specific functions */ 00607 00608 /* 00609 * The CMSIS functions have been implemented as intrinsics in the compiler. 00610 * Please use "carm -?i" to get an up to date list of all intrinsics, 00611 * Including the CMSIS ones. 00612 */ 00613 00614 #endif 00615 00616 /*@}*/ /* end of group CMSIS_Core_InstructionInterface */ 00617 00618 #endif /* __CORE_CMINSTR_H */
Generated on Tue Jul 12 2022 17:17:30 by
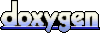