libuav original
Dependents: UAVCAN UAVCAN_Subscriber
cmsis_11cxx.h
00001 /* 00002 * @brief Basic CMSIS include file for LPC11CXX 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2013 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __CMSIS_11CXX_H_ 00033 #define __CMSIS_11CXX_H_ 00034 00035 #include "lpc_types.h" 00036 #include "sys_config.h" 00037 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 00042 /** @defgroup CMSIS_11CXX CHIP: LPC11Cxx CMSIS include file 00043 * @ingroup CHIP_11XX_CMSIS_Drivers 00044 * Applies to LPC1111, LPC1112, LPC1113, LPC1114, LPC11D14, LPC1115, 00045 * LPC11C12, LPC11C13, LPC11C22, and LPC11C34 devices. 00046 * @{ 00047 */ 00048 00049 #if defined(__ARMCC_VERSION) 00050 // Kill warning "#pragma push with no matching #pragma pop" 00051 #pragma diag_suppress 2525 00052 #pragma push 00053 #pragma anon_unions 00054 #elif defined(__CWCC__) 00055 #pragma push 00056 #pragma cpp_extensions on 00057 #elif defined(__GNUC__) 00058 /* anonymous unions are enabled by default */ 00059 #elif defined(__IAR_SYSTEMS_ICC__) 00060 // #pragma push // FIXME not usable for IAR 00061 #pragma language=extended 00062 #else 00063 #error Not supported compiler type 00064 #endif 00065 00066 /* 00067 * ========================================================================== 00068 * ---------- Interrupt Number Definition ----------------------------------- 00069 * ========================================================================== 00070 */ 00071 00072 #if !defined(CHIP_LPC11CXX) 00073 #error Incorrect or missing device variant (CHIP_LPC11AXX) 00074 #endif 00075 00076 /** @defgroup CMSIS_11CXX_IRQ CHIP_LPC11CXX: LPC11CXX/LPC111X peripheral interrupt numbers 00077 * @{ 00078 */ 00079 00080 typedef enum LPC11CXX_IRQn { 00081 NonMaskableInt_IRQn = -14, /*!< 2 Non Maskable Interrupt */ 00082 HardFault_IRQn = -13, /*!< 3 Cortex-M0 Hard Fault Interrupt */ 00083 SVCall_IRQn = -5, /*!< 11 Cortex-M0 SV Call Interrupt */ 00084 PendSV_IRQn = -2, /*!< 14 Cortex-M0 Pend SV Interrupt */ 00085 SysTick_IRQn = -1, /*!< 15 Cortex-M0 System Tick Interrupt */ 00086 00087 PIO0_0_IRQn = 0, /*!< GPIO port 0, pin 0 Interrupt */ 00088 PIO0_1_IRQn = 1, /*!< GPIO port 0, pin 1 Interrupt */ 00089 PIO0_2_IRQn = 2, /*!< GPIO port 0, pin 2 Interrupt */ 00090 PIO0_3_IRQn = 3, /*!< GPIO port 0, pin 3 Interrupt */ 00091 PIO0_4_IRQn = 4, /*!< GPIO port 0, pin 4 Interrupt */ 00092 PIO0_5_IRQn = 5, /*!< GPIO port 0, pin 5 Interrupt */ 00093 PIO0_6_IRQn = 6, /*!< GPIO port 0, pin 6 Interrupt */ 00094 PIO0_7_IRQn = 7, /*!< GPIO port 0, pin 7 Interrupt */ 00095 PIO0_8_IRQn = 8, /*!< GPIO port 0, pin 8 Interrupt */ 00096 PIO0_9_IRQn = 9, /*!< GPIO port 0, pin 9 Interrupt */ 00097 PIO0_10_IRQn = 10, /*!< GPIO port 0, pin 10 Interrupt */ 00098 PIO0_11_IRQn = 11, /*!< GPIO port 0, pin 11 Interrupt */ 00099 PIO1_0_IRQn = 12, /*!< GPIO port 1, pin 0 Interrupt */ 00100 CAN_IRQn = 13, /*!< CAN Interrupt */ 00101 SSP1_IRQn = 14, /*!< SSP1 Interrupt */ 00102 I2C0_IRQn = 15, /*!< I2C Interrupt */ 00103 TIMER_16_0_IRQn = 16, /*!< 16-bit Timer0 Interrupt */ 00104 TIMER_16_1_IRQn = 17, /*!< 16-bit Timer1 Interrupt */ 00105 TIMER_32_0_IRQn = 18, /*!< 32-bit Timer0 Interrupt */ 00106 TIMER_32_1_IRQn = 19, /*!< 32-bit Timer1 Interrupt */ 00107 SSP0_IRQn = 20, /*!< SSP0 Interrupt */ 00108 UART0_IRQn = 21, /*!< UART Interrupt */ 00109 Reserved22_IRQn = 22, 00110 Reserved23_IRQn = 23, 00111 ADC_IRQn = 24, /*!< A/D Converter Interrupt */ 00112 WDT_IRQn = 25, /*!< Watchdog timer Interrupt */ 00113 BOD_IRQn = 26, /*!< Brown Out Detect(BOD) Interrupt */ 00114 Reserved27_IRQn = 27, 00115 EINT3_IRQn = 28, /*!< External Interrupt 3 Interrupt */ 00116 EINT2_IRQn = 29, /*!< External Interrupt 2 Interrupt */ 00117 EINT1_IRQn = 30, /*!< External Interrupt 1 Interrupt */ 00118 EINT0_IRQn = 31, /*!< External Interrupt 0 Interrupt */ 00119 } LPC11CXX_IRQn_Type; 00120 00121 /** 00122 * @} 00123 */ 00124 00125 /* 00126 * ========================================================================== 00127 * ----------- Processor and Core Peripheral Section ------------------------ 00128 * ========================================================================== 00129 */ 00130 00131 /** @defgroup CMSIS_11CXX_COMMON CHIP: LPC11Cxx Cortex CMSIS definitions 00132 * @{ 00133 */ 00134 00135 /* Configuration of the Cortex-M0 Processor and Core Peripherals */ 00136 #define __MPU_PRESENT 0 /*!< MPU present or not */ 00137 #define __NVIC_PRIO_BITS 2 /*!< Number of Bits used for Priority Levels */ 00138 #define __Vendor_SysTickConfig 0 /*!< Set to 1 if different SysTick Config is used */ 00139 00140 /** 00141 * @} 00142 */ 00143 00144 /** 00145 * @} 00146 */ 00147 00148 #ifdef __cplusplus 00149 } 00150 #endif 00151 00152 #endif /* __CMSIS_11CXX_H_ */
Generated on Tue Jul 12 2022 17:17:30 by
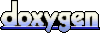