libuav original
Dependents: UAVCAN UAVCAN_Subscriber
chip.h
00001 /* 00002 * @brief LPC11xx basic chip inclusion file 00003 * 00004 * Copyright(C) NXP Semiconductors, 2012 00005 * All rights reserved. 00006 * 00007 * Software that is described herein is for illustrative purposes only 00008 * which provides customers with programming information regarding the 00009 * LPC products. This software is supplied "AS IS" without any warranties of 00010 * any kind, and NXP Semiconductors and its licensor disclaim any and 00011 * all warranties, express or implied, including all implied warranties of 00012 * merchantability, fitness for a particular purpose and non-infringement of 00013 * intellectual property rights. NXP Semiconductors assumes no responsibility 00014 * or liability for the use of the software, conveys no license or rights under any 00015 * patent, copyright, mask work right, or any other intellectual property rights in 00016 * or to any products. NXP Semiconductors reserves the right to make changes 00017 * in the software without notification. NXP Semiconductors also makes no 00018 * representation or warranty that such application will be suitable for the 00019 * specified use without further testing or modification. 00020 * 00021 * Permission to use, copy, modify, and distribute this software and its 00022 * documentation is hereby granted, under NXP Semiconductors' and its 00023 * licensor's relevant copyrights in the software, without fee, provided that it 00024 * is used in conjunction with NXP Semiconductors microcontrollers. This 00025 * copyright, permission, and disclaimer notice must appear in all copies of 00026 * this code. 00027 */ 00028 00029 #ifndef __CHIP_H_ 00030 #define __CHIP_H_ 00031 00032 #include "lpc_types.h" 00033 #include "sys_config.h" 00034 #include "cmsis.h" 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 #ifndef CORE_M0 00041 #error CORE_M0 is not defined for the LPC11xx architecture 00042 #error CORE_M0 should be defined as part of your compiler define list 00043 #endif 00044 00045 #if !defined(ENABLE_UNTESTED_CODE) 00046 #if defined(CHIP_LPC110X) 00047 #warning The LCP110X code has not been tested with a platform. This code should \ 00048 build without errors but may not work correctly for the device. To disable this \ 00049 #warning message, define ENABLE_UNTESTED_CODE. 00050 #endif 00051 #if defined(CHIP_LPC11XXLV) 00052 #warning The LPC11XXLV code has not been tested with a platform. This code should \ 00053 build without errors but may not work correctly for the device. To disable this \ 00054 #warning message, define ENABLE_UNTESTED_CODE. 00055 #endif 00056 #if defined(CHIP_LPC11AXX) 00057 #warning The LPC11AXX code has not been tested with a platform. This code should \ 00058 build without errors but may not work correctly for the device. To disable this \ 00059 #warning message, define ENABLE_UNTESTED_CODE. 00060 #endif 00061 #if defined(CHIP_LPC11EXX) 00062 #warning The LPC11EXX code has not been tested with a platform. This code should \ 00063 build without errors but may not work correctly for the device. To disable this \ 00064 warning message, define ENABLE_UNTESTED_CODE. 00065 #endif 00066 #endif 00067 00068 #if !defined(CHIP_LPC110X) && !defined(CHIP_LPC11XXLV) && !defined(CHIP_LPC11AXX) && \ 00069 !defined(CHIP_LPC11CXX) && !defined(CHIP_LPC11EXX) && !defined(CHIP_LPC11UXX) && \ 00070 !defined(CHIP_LPC1125) 00071 #error CHIP_LPC110x/CHIP_LPC11XXLV/CHIP_LPC11AXX/CHIP_LPC11CXX/CHIP_LPC11EXX/CHIP_LPC11UXX/CHIP_LPC1125 is not defined! 00072 #endif 00073 00074 /* Peripheral mapping per device 00075 Peripheral Device(s) 00076 ---------------------------- ------------------------------------------------------------------------------------------------------------- 00077 I2C(40000000) CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00078 WDT(40004000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00079 UART0(40008000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX CHIP_LPC1125 00080 UART1(40020000) CHIP_LPC1125 00081 UART2(40024000) CHIP_LPC1125 00082 USART/SMARTCARD(40008000) CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX 00083 TIMER0_16(4000C000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00084 TIMER1_16(40010000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00085 TIMER0_32(40014000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00086 TIMER1_32(40018000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00087 ADC(4001C000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00088 DAC(40024000) CHIP_LPC11AXX 00089 ACMP(40028000) CHIP_LPC11AXX 00090 PMU(40038000) CHIP_LPC110x/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX CHIP_LPC1125 00091 FLASH_CTRL(4003C000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX CHIP_LPC1125 00092 FLASH_EEPROM(4003C000) CHIP_LPC11EXX/ CHIP_LPC11AXX 00093 SPI0(40040000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX 00094 SSP0(40040000) CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00095 IOCONF(40044000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00096 SYSCON(40048000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX/ CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00097 GPIOINTS(4004C000) CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX 00098 USB(40080000) CHIP_LPC11UXX 00099 CCAN(40050000) CHIP_LPC11CXX 00100 SPI1(40058000) CHIP_LPC11CXX 00101 SSP1(40058000) CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX CHIP_LPC1125 00102 GPIO_GRP0_INT(4005C000) CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX 00103 GPIO_GRP1_INT(40060000) CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX 00104 GPIO_PORT(50000000) CHIP_LPC11UXX/ CHIP_LPC11EXX/ CHIP_LPC11AXX 00105 GPIO_PIO0(50000000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX CHIP_LPC1125 00106 GPIO_PIO1(50010000) CHIP_LPC110x/ CHIP_LPC11XXLV/ CHIP_LPC11CXX CHIP_LPC1125 00107 GPIO_PIO2(50020000) CHIP_LPC11XXLV/ CHIP_LPC11CXX CHIP_LPC1125 00108 GPIO_PIO3(50030000) CHIP_LPC11XXLV/ CHIP_LPC11CXX CHIP_LPC1125 00109 */ 00110 00111 /** @defgroup PERIPH_11XX_BASE CHIP: LPC11xx Peripheral addresses and register set declarations 00112 * @ingroup CHIP_11XX_Drivers 00113 * @{ 00114 */ 00115 00116 #define LPC_I2C_BASE 0x40000000 00117 #define LPC_WWDT_BASE 0x40004000 00118 #define LPC_USART_BASE 0x40008000 00119 #define LPC_TIMER16_0_BASE 0x4000C000 00120 #define LPC_TIMER16_1_BASE 0x40010000 00121 #define LPC_TIMER32_0_BASE 0x40014000 00122 #define LPC_TIMER32_1_BASE 0x40018000 00123 #define LPC_ADC_BASE 0x4001C000 00124 #define LPC_DAC_BASE 0x40024000 00125 #define LPC_ACMP_BASE 0x40028000 00126 #define LPC_PMU_BASE 0x40038000 00127 #define LPC_FLASH_BASE 0x4003C000 00128 #define LPC_SSP0_BASE 0x40040000 00129 #define LPC_IOCON_BASE 0x40044000 00130 #define LPC_SYSCTL_BASE 0x40048000 00131 #define LPC_USB0_BASE 0x40080000 00132 #define LPC_CAN0_BASE 0x40050000 00133 #define LPC_SSP1_BASE 0x40058000 00134 #if defined(CHIP_LPC1125) 00135 #define LPC_USART1_BASE 0x40020000 00136 #define LPC_USART2_BASE 0x40024000 00137 #endif 00138 #if defined(CHIP_LPC11UXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11AXX) 00139 #define LPC_GPIO_PIN_INT_BASE 0x4004C000 00140 #define LPC_GPIO_GROUP_INT0_BASE 0x4005C000 00141 #define LPC_GPIO_GROUP_INT1_BASE 0x40060000 00142 #define LPC_GPIO_PORT_BASE 0x50000000 00143 #else 00144 #define LPC_GPIO_PORT0_BASE 0x50000000 00145 #define LPC_GPIO_PORT1_BASE 0x50010000 00146 #if defined(CHIP_LPC11XXLV) || defined(CHIP_LPC11CXX) || defined(CHIP_LPC1125) 00147 #define LPC_GPIO_PORT2_BASE 0x50020000 00148 #define LPC_GPIO_PORT3_BASE 0x50030000 00149 #endif /* defined(CHIP_LPC11XXLV) || defined(CHIP_LPC11CXX) || defined(CHIP_LPC1125) */ 00150 #endif /* defined(CHIP_LPC11UXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11AXX) */ 00151 #define IAP_ENTRY_LOCATION 0X1FFF1FF1 00152 #define LPC_ROM_API_BASE_LOC 0x1FFF1FF8 00153 00154 #if !defined(CHIP_LPC110x) 00155 #define LPC_I2C ((LPC_I2C_T *) LPC_I2C_BASE) 00156 #endif 00157 00158 #define LPC_WWDT ((LPC_WWDT_T *) LPC_WWDT_BASE) 00159 #define LPC_USART ((LPC_USART_T *) LPC_USART_BASE) 00160 #define LPC_TIMER16_0 ((LPC_TIMER_T *) LPC_TIMER16_0_BASE) 00161 #define LPC_TIMER16_1 ((LPC_TIMER_T *) LPC_TIMER16_1_BASE) 00162 #define LPC_TIMER32_0 ((LPC_TIMER_T *) LPC_TIMER32_0_BASE) 00163 #define LPC_TIMER32_1 ((LPC_TIMER_T *) LPC_TIMER32_1_BASE) 00164 #define LPC_ADC ((LPC_ADC_T *) LPC_ADC_BASE) 00165 00166 #if defined(CHIP_LPC1125) 00167 #define LPC_USART0 LPC_USART 00168 #define LPC_USART1 ((LPC_USART_T *) LPC_USART1_BASE) 00169 #define LPC_USART2 ((LPC_USART_T *) LPC_USART2_BASE) 00170 #endif 00171 00172 #if defined(CHIP_LPC11AXX) 00173 #define LPC_DAC ((LPC_DAC_T *) LPC_DAC_BASE) 00174 #define LPC_CMP ((LPC_CMP_T *) LPC_ACMP_BASE) 00175 #endif 00176 00177 #define LPC_PMU ((LPC_PMU_T *) LPC_PMU_BASE) 00178 #define LPC_FMC ((LPC_FMC_T *) LPC_FLASH_BASE) 00179 #define LPC_SSP0 ((LPC_SSP_T *) LPC_SSP0_BASE) 00180 #define LPC_IOCON ((LPC_IOCON_T *) LPC_IOCON_BASE) 00181 #define LPC_SYSCTL ((LPC_SYSCTL_T *) LPC_SYSCTL_BASE) 00182 #if defined(CHIP_LPC11CXX) || defined(CHIP_LPC11UXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11AXX) || defined(CHIP_LPC1125) 00183 #define LPC_SSP1 ((LPC_SSP_T *) LPC_SSP1_BASE) 00184 #endif 00185 #define LPC_USB ((LPC_USB_T *) LPC_USB0_BASE) 00186 00187 #if defined(CHIP_LPC11UXX) || defined(CHIP_LPC11EXX) || defined(CHIP_LPC11AXX) 00188 #define LPC_PININT ((LPC_PIN_INT_T *) LPC_GPIO_PIN_INT_BASE) 00189 #define LPC_GPIOGROUP ((LPC_GPIOGROUPINT_T *) LPC_GPIO_GROUP_INT0_BASE) 00190 #define LPC_GPIO ((LPC_GPIO_T *) LPC_GPIO_PORT_BASE) 00191 #else 00192 #define LPC_GPIO ((LPC_GPIO_T *) LPC_GPIO_PORT0_BASE) 00193 #endif 00194 00195 #define LPC_ROM_API (*((LPC_ROM_API_T * *) LPC_ROM_API_BASE_LOC)) 00196 00197 00198 /** 00199 * @} 00200 */ 00201 00202 /** @ingroup CHIP_11XX_DRIVER_OPTIONS 00203 */ 00204 00205 /** 00206 * @brief System oscillator rate 00207 * This value is defined externally to the chip layer and contains 00208 * the value in Hz for the external oscillator for the board. If using the 00209 * internal oscillator, this rate can be 0. 00210 */ 00211 extern const uint32_t OscRateIn; 00212 00213 /** 00214 * @} 00215 */ 00216 00217 /** @ingroup CHIP_11XX_DRIVER_OPTIONS 00218 */ 00219 00220 /** 00221 * @brief Clock rate on the CLKIN pin 00222 * This value is defined externally to the chip layer and contains 00223 * the value in Hz for the CLKIN pin for the board. If this pin isn't used, 00224 * this rate can be 0. 00225 */ 00226 extern const uint32_t ExtRateIn; 00227 00228 /** 00229 * @} 00230 */ 00231 00232 #include "pmu_11xx.h" 00233 #include "fmc_11xx.h" 00234 #include "sysctl_11xx.h" 00235 #include "clock_11xx.h" 00236 #include "iocon_11xx.h" 00237 #include "timer_11xx.h" 00238 #include "uart_11xx.h" 00239 #include "wwdt_11xx.h" 00240 #include "ssp_11xx.h" 00241 #include "romapi_11xx.h" 00242 00243 #if !defined(CHIP_LPC1125) 00244 /* All LPC1xx devices except the LPC1125 */ 00245 #include "adc_11xx.h" 00246 00247 #else 00248 /* LPC1125 has different IP than other LPC11xx devices */ 00249 #include "adc_1125.h" 00250 #endif 00251 00252 /* Different GPIO/GPIOGROUP/PININT blocks for parts with similar numbers */ 00253 #if defined(CHIP_LPC11CXX) || defined(CHIP_LPC110X) || defined(CHIP_LPC11XXLV) || defined(CHIP_LPC1125) 00254 #include "gpio_11xx_2.h" 00255 #else 00256 #include "gpio_11xx_1.h" 00257 #include "gpiogroup_11xx.h" 00258 #include "pinint_11xx.h" 00259 #endif 00260 00261 /* Family specific drivers */ 00262 #if defined(CHIP_LPC11AXX) 00263 #include "acmp_11xx.h" 00264 #include "dac_11xx.h" 00265 #endif 00266 #if !defined(CHIP_LPC110X) 00267 #include "i2c_11xx.h" 00268 #endif 00269 #if defined(CHIP_LPC11CXX) 00270 #include "ccand_11xx.h" 00271 #endif 00272 #if defined(CHIP_LPC11UXX) 00273 #include "usbd_11xx.h" 00274 #endif 00275 00276 /** @defgroup SUPPORT_11XX_FUNC CHIP: LPC11xx support functions 00277 * @ingroup CHIP_11XX_Drivers 00278 * @{ 00279 */ 00280 00281 /** 00282 * @brief Current system clock rate, mainly used for sysTick 00283 */ 00284 extern uint32_t SystemCoreClock; 00285 00286 /** 00287 * @brief Update system core clock rate, should be called if the 00288 * system has a clock rate change 00289 * @return None 00290 */ 00291 void SystemCoreClockUpdate(void); 00292 00293 /** 00294 * @brief Set up and initialize hardware prior to call to main() 00295 * @return None 00296 * @note Chip_SystemInit() is called prior to the application and sets up 00297 * system clocking prior to the application starting. 00298 */ 00299 void Chip_SystemInit(void); 00300 00301 /** 00302 * @} 00303 */ 00304 00305 #ifdef __cplusplus 00306 } 00307 #endif 00308 00309 #endif /* __CHIP_H_ */
Generated on Tue Jul 12 2022 17:17:30 by
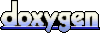